Note
Go to the end to download the full example code
Visualize bad sensors per trial#
This example demonstrates how to use autoreject
to
visualize the bad sensors in each trial
# Author: Mainak Jas <mainak.jas@telecom-paristech.fr>
# Denis A. Engemann <denis.engemann@gmail.com>
# License: BSD-3-Clause
# sphinx_gallery_thumbnail_number = 2
First, we download the data from OpenfMRI which is hosted on OpenNeuro.
We will do this using openneuro-py
which can be installed using pip
(pip install openneuro-py
).
import os
import openneuro
import autoreject
dataset = 'ds000117' # The id code on OpenNeuro for this example dataset
subject_id = 16 # OpenfMRI format of subject numbering
target_dir = os.path.join(
os.path.dirname(autoreject.__file__), '..', 'examples', dataset)
os.makedirs(target_dir, exist_ok=True)
openneuro.download(dataset=dataset, target_dir=target_dir,
include=[f'sub-{subject_id}/ses-meg/'])
Hello! This is openneuro-py 2022.4.0. Great to see you!
Please report problems and bugs at
https://github.com/hoechenberger/openneuro-py/issues
Preparing to download ds000117 ...
Traversing directories for ds000117: 0 entities [00:00, ? entities/s]
Traversing directories for ds000117: 23 entities [00:00, 48.68 entities/s]
Traversing directories for ds000117: 28 entities [00:01, 13.24 entities/s]
Traversing directories for ds000117: 490 entities [00:02, 226.99 entities/s]
Traversing directories for ds000117: 956 entities [00:03, 406.78 entities/s]
Traversing directories for ds000117: 1007 entities [00:14, 48.20 entities/s]
Traversing directories for ds000117: 1019 entities [00:14, 47.00 entities/s]
Traversing directories for ds000117: 1053 entities [00:15, 48.53 entities/s]
Traversing directories for ds000117: 1066 entities [00:17, 60.46 entities/s]
Retrieving up to 22 files (5 concurrent downloads).
Skipping dataset_description.json: already downloaded.: 100%|#######################################################################################| 1.07k/1.07k [00:00<?, ?B/s]
Skipping participants.tsv: already downloaded.: 100%|###################################################################################################| 333/333 [00:00<?, ?B/s]
Skipping sub-16_ses-meg_scans.tsv: already downloaded.: 100%|###########################################################################################| 475/475 [00:00<?, ?B/s]
Skipping CHANGES: already downloaded.: 100%|########################################################################################################| 1.79k/1.79k [00:00<?, ?B/s]
Skipping README: already downloaded.: 100%|#########################################################################################################| 5.92k/5.92k [00:00<?, ?B/s]
Skipping sub-16_ses-meg_task-facerecognition_channels.tsv: already downloaded.: 100%|###############################################################| 23.0k/23.0k [00:00<?, ?B/s]
Skipping sub-16_ses-meg_task-facerecognition_meg.json: already downloaded.: 100%|###################################################################| 1.67k/1.67k [00:00<?, ?B/s]
Skipping sub-16_ses-meg_task-facerecognition_events.tsv: already downloaded.: 100%|#################################################################| 8.75k/8.75k [00:00<?, ?B/s]
Skipping sub-16_ses-meg_coordsystem.json: already downloaded.: 100%|################################################################################| 1.81k/1.81k [00:00<?, ?B/s]
Skipping sub-16_ses-meg_headshape.pos: already downloaded.: 100%|###################################################################################| 4.22k/4.22k [00:00<?, ?B/s]
Skipping sub-16_ses-meg_task-facerecognition_run-01_events.tsv: already downloaded.: 100%|##########################################################| 6.19k/6.19k [00:00<?, ?B/s]
Skipping sub-16_ses-meg_task-facerecognition_run-02_events.tsv: already downloaded.: 100%|##########################################################| 6.06k/6.06k [00:00<?, ?B/s]
Skipping sub-16_ses-meg_task-facerecognition_run-03_events.tsv: already downloaded.: 100%|##########################################################| 6.19k/6.19k [00:00<?, ?B/s]
Skipping sub-16_ses-meg_task-facerecognition_run-04_events.tsv: already downloaded.: 100%|##########################################################| 6.23k/6.23k [00:00<?, ?B/s]
Skipping sub-16_ses-meg_task-facerecognition_run-05_events.tsv: already downloaded.: 100%|##########################################################| 6.15k/6.15k [00:00<?, ?B/s]
Skipping sub-16_ses-meg_task-facerecognition_run-06_events.tsv: already downloaded.: 100%|##########################################################| 6.24k/6.24k [00:00<?, ?B/s]
Skipping sub-16_ses-meg_task-facerecognition_run-01_meg.fif: already downloaded.: 100%|###############################################################| 824M/824M [00:00<?, ?B/s]
Skipping sub-16_ses-meg_task-facerecognition_run-02_meg.fif: already downloaded.: 100%|###############################################################| 835M/835M [00:00<?, ?B/s]
Skipping sub-16_ses-meg_task-facerecognition_run-05_meg.fif: already downloaded.: 100%|###############################################################| 824M/824M [00:00<?, ?B/s]
Skipping sub-16_ses-meg_task-facerecognition_run-03_meg.fif: already downloaded.: 100%|###############################################################| 832M/832M [00:00<?, ?B/s]
Skipping sub-16_ses-meg_task-facerecognition_run-04_meg.fif: already downloaded.: 100%|###############################################################| 830M/830M [00:00<?, ?B/s]
Skipping sub-16_ses-meg_task-facerecognition_run-06_meg.fif: already downloaded.: 100%|###############################################################| 822M/822M [00:00<?, ?B/s]
Finished downloading ds000117.
Please enjoy your brains.
We will create epochs with data starting 200 ms before trigger onset and continuing up to 800 ms after that. The data contains visual stimuli for famous faces, unfamiliar faces, as well as scrambled faces.
Let us now load all the epochs into memory and concatenate them
import mne # noqa
epochs = list()
for run in range(3, 7):
run_fname = os.path.join(target_dir, f'sub-{subject_id}', 'ses-meg', 'meg',
f'sub-{subject_id}_ses-meg_task-facerecognition'
'_run-{:02d}_meg.fif'.format(run))
raw = mne.io.read_raw_fif(run_fname, preload=True)
raw.pick_types(eeg=True, meg=False, stim=True) # less memory + computation
raw.filter(1., 40., l_trans_bandwidth=0.5, n_jobs=1, verbose='INFO')
raw.set_channel_types({'EEG061': 'eog', 'EEG062': 'eog',
'EEG063': 'ecg', 'EEG064': 'misc'})
raw.rename_channels({'EEG061': 'EOG061', 'EEG062': 'EOG062',
'EEG063': 'ECG063', 'EEG064': 'MISC'})
events = mne.find_events(raw, stim_channel='STI101',
consecutive='increasing',
min_duration=0.003, verbose=True)
# Read epochs
mne.io.set_eeg_reference(raw)
epoch = mne.Epochs(raw, events, events_id, tmin, tmax, proj=True,
baseline=None,
preload=False, reject=None, decim=4)
epochs.append(epoch)
# Same `dev_head_t` for all runs so that we can concatenate them.
epoch.info['dev_head_t'] = epochs[0].info['dev_head_t']
epochs = mne.epochs.concatenate_epochs(epochs)
Opening raw data file c:\users\stefan\desktop\git-repos\autoreject\autoreject\..\examples\ds000117\sub-16\ses-meg\meg\sub-16_ses-meg_task-facerecognition_run-03_meg.fif...
Read a total of 8 projection items:
mag_ssp_upright.fif : PCA-mags-v1 (1 x 306) idle
mag_ssp_upright.fif : PCA-mags-v2 (1 x 306) idle
mag_ssp_upright.fif : PCA-mags-v3 (1 x 306) idle
mag_ssp_upright.fif : PCA-mags-v4 (1 x 306) idle
mag_ssp_upright.fif : PCA-mags-v5 (1 x 306) idle
grad_ssp_upright.fif : PCA-grad-v1 (1 x 306) idle
grad_ssp_upright.fif : PCA-grad-v2 (1 x 306) idle
grad_ssp_upright.fif : PCA-grad-v3 (1 x 306) idle
Range : 40700 ... 588499 = 37.000 ... 534.999 secs
Ready.
Reading 0 ... 547799 = 0.000 ... 497.999 secs...
NOTE: pick_types() is a legacy function. New code should use inst.pick(...).
Removing projector <Projection | mag_ssp_upright.fif : PCA-mags-v1, active : False, n_channels : 306>
Removing projector <Projection | mag_ssp_upright.fif : PCA-mags-v2, active : False, n_channels : 306>
Removing projector <Projection | mag_ssp_upright.fif : PCA-mags-v3, active : False, n_channels : 306>
Removing projector <Projection | mag_ssp_upright.fif : PCA-mags-v4, active : False, n_channels : 306>
Removing projector <Projection | mag_ssp_upright.fif : PCA-mags-v5, active : False, n_channels : 306>
Removing projector <Projection | grad_ssp_upright.fif : PCA-grad-v1, active : False, n_channels : 306>
Removing projector <Projection | grad_ssp_upright.fif : PCA-grad-v2, active : False, n_channels : 306>
Removing projector <Projection | grad_ssp_upright.fif : PCA-grad-v3, active : False, n_channels : 306>
Filtering raw data in 1 contiguous segment
Setting up band-pass filter from 1 - 40 Hz
FIR filter parameters
---------------------
Designing a one-pass, zero-phase, non-causal bandpass filter:
- Windowed time-domain design (firwin) method
- Hamming window with 0.0194 passband ripple and 53 dB stopband attenuation
- Lower passband edge: 1.00
- Lower transition bandwidth: 0.50 Hz (-6 dB cutoff frequency: 0.75 Hz)
- Upper passband edge: 40.00 Hz
- Upper transition bandwidth: 10.00 Hz (-6 dB cutoff frequency: 45.00 Hz)
- Filter length: 7261 samples (6.601 s)
C:\Users\stefan\Desktop\git-repos\autoreject\examples\plot_visualize_bad_epochs.py:57: RuntimeWarning: The unit for channel(s) EEG064 has changed from V to NA.
raw.set_channel_types({'EEG061': 'eog', 'EEG062': 'eog',
296 events found
Event IDs: [ 5 6 7 13 14 15 17 18 19 261 262 263 269 271
273 274 275 4096 4101 4102 4103 4109 4110 4111 4113 4114 4115]
EEG channel type selected for re-referencing
Applying average reference.
Applying a custom ('EEG',) reference.
Not setting metadata
49 matching events found
No baseline correction applied
0 projection items activated
Opening raw data file c:\users\stefan\desktop\git-repos\autoreject\autoreject\..\examples\ds000117\sub-16\ses-meg\meg\sub-16_ses-meg_task-facerecognition_run-04_meg.fif...
Read a total of 8 projection items:
mag_ssp_upright.fif : PCA-mags-v1 (1 x 306) idle
mag_ssp_upright.fif : PCA-mags-v2 (1 x 306) idle
mag_ssp_upright.fif : PCA-mags-v3 (1 x 306) idle
mag_ssp_upright.fif : PCA-mags-v4 (1 x 306) idle
mag_ssp_upright.fif : PCA-mags-v5 (1 x 306) idle
grad_ssp_upright.fif : PCA-grad-v1 (1 x 306) idle
grad_ssp_upright.fif : PCA-grad-v2 (1 x 306) idle
grad_ssp_upright.fif : PCA-grad-v3 (1 x 306) idle
Range : 36300 ... 582999 = 33.000 ... 529.999 secs
Ready.
Reading 0 ... 546699 = 0.000 ... 496.999 secs...
NOTE: pick_types() is a legacy function. New code should use inst.pick(...).
Removing projector <Projection | mag_ssp_upright.fif : PCA-mags-v1, active : False, n_channels : 306>
Removing projector <Projection | mag_ssp_upright.fif : PCA-mags-v2, active : False, n_channels : 306>
Removing projector <Projection | mag_ssp_upright.fif : PCA-mags-v3, active : False, n_channels : 306>
Removing projector <Projection | mag_ssp_upright.fif : PCA-mags-v4, active : False, n_channels : 306>
Removing projector <Projection | mag_ssp_upright.fif : PCA-mags-v5, active : False, n_channels : 306>
Removing projector <Projection | grad_ssp_upright.fif : PCA-grad-v1, active : False, n_channels : 306>
Removing projector <Projection | grad_ssp_upright.fif : PCA-grad-v2, active : False, n_channels : 306>
Removing projector <Projection | grad_ssp_upright.fif : PCA-grad-v3, active : False, n_channels : 306>
Filtering raw data in 1 contiguous segment
Setting up band-pass filter from 1 - 40 Hz
FIR filter parameters
---------------------
Designing a one-pass, zero-phase, non-causal bandpass filter:
- Windowed time-domain design (firwin) method
- Hamming window with 0.0194 passband ripple and 53 dB stopband attenuation
- Lower passband edge: 1.00
- Lower transition bandwidth: 0.50 Hz (-6 dB cutoff frequency: 0.75 Hz)
- Upper passband edge: 40.00 Hz
- Upper transition bandwidth: 10.00 Hz (-6 dB cutoff frequency: 45.00 Hz)
- Filter length: 7261 samples (6.601 s)
C:\Users\stefan\Desktop\git-repos\autoreject\examples\plot_visualize_bad_epochs.py:57: RuntimeWarning: The unit for channel(s) EEG064 has changed from V to NA.
raw.set_channel_types({'EEG061': 'eog', 'EEG062': 'eog',
296 events found
Event IDs: [ 5 6 7 13 14 15 17 18 19 256 261 262 263 269
271 273 274 275 4096 4101 4102 4103 4109 4110 4111 4113 4114 4115]
EEG channel type selected for re-referencing
Applying average reference.
Applying a custom ('EEG',) reference.
Not setting metadata
49 matching events found
No baseline correction applied
0 projection items activated
Opening raw data file c:\users\stefan\desktop\git-repos\autoreject\autoreject\..\examples\ds000117\sub-16\ses-meg\meg\sub-16_ses-meg_task-facerecognition_run-05_meg.fif...
Read a total of 8 projection items:
mag_ssp_upright.fif : PCA-mags-v1 (1 x 306) idle
mag_ssp_upright.fif : PCA-mags-v2 (1 x 306) idle
mag_ssp_upright.fif : PCA-mags-v3 (1 x 306) idle
mag_ssp_upright.fif : PCA-mags-v4 (1 x 306) idle
mag_ssp_upright.fif : PCA-mags-v5 (1 x 306) idle
grad_ssp_upright.fif : PCA-grad-v1 (1 x 306) idle
grad_ssp_upright.fif : PCA-grad-v2 (1 x 306) idle
grad_ssp_upright.fif : PCA-grad-v3 (1 x 306) idle
Range : 60500 ... 602799 = 55.000 ... 547.999 secs
Ready.
Reading 0 ... 542299 = 0.000 ... 492.999 secs...
NOTE: pick_types() is a legacy function. New code should use inst.pick(...).
Removing projector <Projection | mag_ssp_upright.fif : PCA-mags-v1, active : False, n_channels : 306>
Removing projector <Projection | mag_ssp_upright.fif : PCA-mags-v2, active : False, n_channels : 306>
Removing projector <Projection | mag_ssp_upright.fif : PCA-mags-v3, active : False, n_channels : 306>
Removing projector <Projection | mag_ssp_upright.fif : PCA-mags-v4, active : False, n_channels : 306>
Removing projector <Projection | mag_ssp_upright.fif : PCA-mags-v5, active : False, n_channels : 306>
Removing projector <Projection | grad_ssp_upright.fif : PCA-grad-v1, active : False, n_channels : 306>
Removing projector <Projection | grad_ssp_upright.fif : PCA-grad-v2, active : False, n_channels : 306>
Removing projector <Projection | grad_ssp_upright.fif : PCA-grad-v3, active : False, n_channels : 306>
Filtering raw data in 1 contiguous segment
Setting up band-pass filter from 1 - 40 Hz
FIR filter parameters
---------------------
Designing a one-pass, zero-phase, non-causal bandpass filter:
- Windowed time-domain design (firwin) method
- Hamming window with 0.0194 passband ripple and 53 dB stopband attenuation
- Lower passband edge: 1.00
- Lower transition bandwidth: 0.50 Hz (-6 dB cutoff frequency: 0.75 Hz)
- Upper passband edge: 40.00 Hz
- Upper transition bandwidth: 10.00 Hz (-6 dB cutoff frequency: 45.00 Hz)
- Filter length: 7261 samples (6.601 s)
C:\Users\stefan\Desktop\git-repos\autoreject\examples\plot_visualize_bad_epochs.py:57: RuntimeWarning: The unit for channel(s) EEG064 has changed from V to NA.
raw.set_channel_types({'EEG061': 'eog', 'EEG062': 'eog',
292 events found
Event IDs: [ 5 6 7 13 14 15 17 18 19 256 261 269 271 273
274 275 4096 4101 4102 4103 4109 4110 4111 4113 4114 4115]
EEG channel type selected for re-referencing
Applying average reference.
Applying a custom ('EEG',) reference.
Not setting metadata
49 matching events found
No baseline correction applied
0 projection items activated
Opening raw data file c:\users\stefan\desktop\git-repos\autoreject\autoreject\..\examples\ds000117\sub-16\ses-meg\meg\sub-16_ses-meg_task-facerecognition_run-06_meg.fif...
Read a total of 8 projection items:
mag_ssp_upright.fif : PCA-mags-v1 (1 x 306) idle
mag_ssp_upright.fif : PCA-mags-v2 (1 x 306) idle
mag_ssp_upright.fif : PCA-mags-v3 (1 x 306) idle
mag_ssp_upright.fif : PCA-mags-v4 (1 x 306) idle
mag_ssp_upright.fif : PCA-mags-v5 (1 x 306) idle
grad_ssp_upright.fif : PCA-grad-v1 (1 x 306) idle
grad_ssp_upright.fif : PCA-grad-v2 (1 x 306) idle
grad_ssp_upright.fif : PCA-grad-v3 (1 x 306) idle
Range : 27500 ... 568699 = 25.000 ... 516.999 secs
Ready.
Reading 0 ... 541199 = 0.000 ... 491.999 secs...
NOTE: pick_types() is a legacy function. New code should use inst.pick(...).
Removing projector <Projection | mag_ssp_upright.fif : PCA-mags-v1, active : False, n_channels : 306>
Removing projector <Projection | mag_ssp_upright.fif : PCA-mags-v2, active : False, n_channels : 306>
Removing projector <Projection | mag_ssp_upright.fif : PCA-mags-v3, active : False, n_channels : 306>
Removing projector <Projection | mag_ssp_upright.fif : PCA-mags-v4, active : False, n_channels : 306>
Removing projector <Projection | mag_ssp_upright.fif : PCA-mags-v5, active : False, n_channels : 306>
Removing projector <Projection | grad_ssp_upright.fif : PCA-grad-v1, active : False, n_channels : 306>
Removing projector <Projection | grad_ssp_upright.fif : PCA-grad-v2, active : False, n_channels : 306>
Removing projector <Projection | grad_ssp_upright.fif : PCA-grad-v3, active : False, n_channels : 306>
Filtering raw data in 1 contiguous segment
Setting up band-pass filter from 1 - 40 Hz
FIR filter parameters
---------------------
Designing a one-pass, zero-phase, non-causal bandpass filter:
- Windowed time-domain design (firwin) method
- Hamming window with 0.0194 passband ripple and 53 dB stopband attenuation
- Lower passband edge: 1.00
- Lower transition bandwidth: 0.50 Hz (-6 dB cutoff frequency: 0.75 Hz)
- Upper passband edge: 40.00 Hz
- Upper transition bandwidth: 10.00 Hz (-6 dB cutoff frequency: 45.00 Hz)
- Filter length: 7261 samples (6.601 s)
C:\Users\stefan\Desktop\git-repos\autoreject\examples\plot_visualize_bad_epochs.py:57: RuntimeWarning: The unit for channel(s) EEG064 has changed from V to NA.
raw.set_channel_types({'EEG061': 'eog', 'EEG062': 'eog',
295 events found
Event IDs: [ 5 6 7 13 14 15 17 18 19 256 261 262 263 269
270 271 273 274 275 4096 4101 4102 4103 4109 4110 4111 4113 4114
4115]
EEG channel type selected for re-referencing
Applying average reference.
Applying a custom ('EEG',) reference.
Not setting metadata
50 matching events found
No baseline correction applied
0 projection items activated
Using data from preloaded Raw for 49 events and 1101 original time points (prior to decimation) ...
0 bad epochs dropped
Using data from preloaded Raw for 49 events and 1101 original time points (prior to decimation) ...
0 bad epochs dropped
Using data from preloaded Raw for 49 events and 1101 original time points (prior to decimation) ...
0 bad epochs dropped
Using data from preloaded Raw for 50 events and 1101 original time points (prior to decimation) ...
0 bad epochs dropped
Using data from preloaded Raw for 49 events and 1101 original time points (prior to decimation) ...
Using data from preloaded Raw for 49 events and 1101 original time points (prior to decimation) ...
Using data from preloaded Raw for 49 events and 1101 original time points (prior to decimation) ...
Using data from preloaded Raw for 50 events and 1101 original time points (prior to decimation) ...
Not setting metadata
197 matching events found
No baseline correction applied
Now, we apply autoreject
from autoreject import AutoReject, compute_thresholds # noqa
this_epoch = epochs['famous']
exclude = [] # XXX
picks = mne.pick_types(epochs.info, meg=False, eeg=True, stim=False,
eog=False, exclude=exclude)
Note that autoreject.AutoReject
by design supports multiple
channels. If no picks are passed separate solutions will be computed for each
channel type and internally combines. This then readily supports cleaning
unseen epochs from the different channel types used during fit.
Here we only use a subset of channels to save time.
Also note that once the parameters are learned, any data can be repaired
that contains channels that were used during fit. This also means that time
may be saved by fitting autoreject.AutoReject
on a
representative subsample of the data.
ar = AutoReject(picks=picks, random_state=42, n_jobs=1, verbose=True)
epochs_ar, reject_log = ar.fit_transform(this_epoch, return_log=True)
Running autoreject on ch_type=eeg
0%| | Creating augmented epochs : 0/70 [00:00<?, ?it/s]
1%|#5 | Creating augmented epochs : 1/70 [00:00<00:02, 24.99it/s]
3%|###1 | Creating augmented epochs : 2/70 [00:00<00:02, 25.97it/s]
4%|####7 | Creating augmented epochs : 3/70 [00:00<00:02, 26.92it/s]
6%|######3 | Creating augmented epochs : 4/70 [00:00<00:02, 27.13it/s]
7%|#######9 | Creating augmented epochs : 5/70 [00:00<00:02, 27.27it/s]
9%|#########5 | Creating augmented epochs : 6/70 [00:00<00:02, 26.79it/s]
10%|###########1 | Creating augmented epochs : 7/70 [00:00<00:02, 27.00it/s]
11%|############6 | Creating augmented epochs : 8/70 [00:00<00:02, 27.09it/s]
13%|##############2 | Creating augmented epochs : 9/70 [00:00<00:02, 27.18it/s]
14%|###############7 | Creating augmented epochs : 10/70 [00:00<00:02, 27.50it/s]
16%|#################2 | Creating augmented epochs : 11/70 [00:00<00:02, 27.88it/s]
17%|##################8 | Creating augmented epochs : 12/70 [00:00<00:02, 27.99it/s]
19%|####################4 | Creating augmented epochs : 13/70 [00:00<00:02, 28.29it/s]
20%|###################### | Creating augmented epochs : 14/70 [00:00<00:01, 28.23it/s]
21%|#######################5 | Creating augmented epochs : 15/70 [00:00<00:01, 28.46it/s]
23%|#########################1 | Creating augmented epochs : 16/70 [00:00<00:01, 28.70it/s]
24%|##########################7 | Creating augmented epochs : 17/70 [00:00<00:01, 28.94it/s]
26%|############################2 | Creating augmented epochs : 18/70 [00:00<00:01, 28.80it/s]
27%|#############################8 | Creating augmented epochs : 19/70 [00:00<00:01, 29.00it/s]
29%|###############################4 | Creating augmented epochs : 20/70 [00:00<00:01, 29.16it/s]
30%|################################# | Creating augmented epochs : 21/70 [00:00<00:01, 29.05it/s]
31%|##################################5 | Creating augmented epochs : 22/70 [00:00<00:01, 29.20it/s]
33%|####################################1 | Creating augmented epochs : 23/70 [00:00<00:01, 29.35it/s]
34%|#####################################7 | Creating augmented epochs : 24/70 [00:00<00:01, 29.47it/s]
36%|#######################################2 | Creating augmented epochs : 25/70 [00:00<00:01, 29.30it/s]
37%|########################################8 | Creating augmented epochs : 26/70 [00:00<00:01, 29.40it/s]
39%|##########################################4 | Creating augmented epochs : 27/70 [00:00<00:01, 29.45it/s]
40%|############################################ | Creating augmented epochs : 28/70 [00:00<00:01, 29.55it/s]
41%|#############################################5 | Creating augmented epochs : 29/70 [00:00<00:01, 29.50it/s]
43%|###############################################1 | Creating augmented epochs : 30/70 [00:01<00:01, 29.36it/s]
44%|################################################7 | Creating augmented epochs : 31/70 [00:01<00:01, 29.52it/s]
46%|##################################################2 | Creating augmented epochs : 32/70 [00:01<00:01, 29.57it/s]
47%|###################################################8 | Creating augmented epochs : 33/70 [00:01<00:01, 29.48it/s]
49%|#####################################################4 | Creating augmented epochs : 34/70 [00:01<00:01, 29.57it/s]
50%|####################################################### | Creating augmented epochs : 35/70 [00:01<00:01, 29.60it/s]
51%|########################################################5 | Creating augmented epochs : 36/70 [00:01<00:01, 29.48it/s]
53%|##########################################################1 | Creating augmented epochs : 37/70 [00:01<00:01, 29.58it/s]
54%|###########################################################7 | Creating augmented epochs : 38/70 [00:01<00:01, 29.67it/s]
56%|#############################################################2 | Creating augmented epochs : 39/70 [00:01<00:01, 29.76it/s]
57%|##############################################################8 | Creating augmented epochs : 40/70 [00:01<00:01, 29.84it/s]
59%|################################################################4 | Creating augmented epochs : 41/70 [00:01<00:00, 29.73it/s]
60%|################################################################## | Creating augmented epochs : 42/70 [00:01<00:00, 29.81it/s]
61%|###################################################################5 | Creating augmented epochs : 43/70 [00:01<00:00, 29.88it/s]
63%|#####################################################################1 | Creating augmented epochs : 44/70 [00:01<00:00, 29.90it/s]
64%|######################################################################7 | Creating augmented epochs : 45/70 [00:01<00:00, 29.96it/s]
66%|########################################################################2 | Creating augmented epochs : 46/70 [00:01<00:00, 29.97it/s]
67%|#########################################################################8 | Creating augmented epochs : 47/70 [00:01<00:00, 30.09it/s]
69%|###########################################################################4 | Creating augmented epochs : 48/70 [00:01<00:00, 30.02it/s]
70%|############################################################################# | Creating augmented epochs : 49/70 [00:01<00:00, 30.07it/s]
71%|##############################################################################5 | Creating augmented epochs : 50/70 [00:01<00:00, 30.13it/s]
73%|################################################################################1 | Creating augmented epochs : 51/70 [00:01<00:00, 30.21it/s]
74%|#################################################################################7 | Creating augmented epochs : 52/70 [00:01<00:00, 30.06it/s]
76%|###################################################################################2 | Creating augmented epochs : 53/70 [00:01<00:00, 30.12it/s]
77%|####################################################################################8 | Creating augmented epochs : 54/70 [00:01<00:00, 30.18it/s]
79%|######################################################################################4 | Creating augmented epochs : 55/70 [00:01<00:00, 30.06it/s]
80%|######################################################################################## | Creating augmented epochs : 56/70 [00:01<00:00, 30.10it/s]
81%|#########################################################################################5 | Creating augmented epochs : 57/70 [00:01<00:00, 30.18it/s]
83%|###########################################################################################1 | Creating augmented epochs : 58/70 [00:01<00:00, 30.23it/s]
84%|############################################################################################7 | Creating augmented epochs : 59/70 [00:01<00:00, 30.08it/s]
86%|##############################################################################################2 | Creating augmented epochs : 60/70 [00:02<00:00, 29.76it/s]
87%|###############################################################################################8 | Creating augmented epochs : 61/70 [00:02<00:00, 29.66it/s]
89%|#################################################################################################4 | Creating augmented epochs : 62/70 [00:02<00:00, 29.49it/s]
90%|################################################################################################### | Creating augmented epochs : 63/70 [00:02<00:00, 29.45it/s]
91%|####################################################################################################5 | Creating augmented epochs : 64/70 [00:02<00:00, 29.19it/s]
93%|######################################################################################################1 | Creating augmented epochs : 65/70 [00:02<00:00, 28.76it/s]
94%|#######################################################################################################7 | Creating augmented epochs : 66/70 [00:02<00:00, 28.52it/s]
96%|#########################################################################################################2 | Creating augmented epochs : 67/70 [00:02<00:00, 28.49it/s]
97%|##########################################################################################################8 | Creating augmented epochs : 68/70 [00:02<00:00, 28.64it/s]
99%|############################################################################################################4 | Creating augmented epochs : 69/70 [00:02<00:00, 28.59it/s]
100%|##############################################################################################################| Creating augmented epochs : 70/70 [00:02<00:00, 28.71it/s]
100%|##############################################################################################################| Creating augmented epochs : 70/70 [00:02<00:00, 29.13it/s]
0%| | Computing thresholds ... : 0/70 [00:00<?, ?it/s]
1%|#5 | Computing thresholds ... : 1/70 [00:00<00:42, 1.63it/s]
3%|###1 | Computing thresholds ... : 2/70 [00:01<00:40, 1.69it/s]
4%|####8 | Computing thresholds ... : 3/70 [00:01<00:40, 1.66it/s]
6%|######3 | Computing thresholds ... : 4/70 [00:02<00:38, 1.69it/s]
7%|######## | Computing thresholds ... : 5/70 [00:02<00:38, 1.70it/s]
9%|#########6 | Computing thresholds ... : 6/70 [00:03<00:37, 1.71it/s]
10%|###########2 | Computing thresholds ... : 7/70 [00:04<00:37, 1.70it/s]
11%|############7 | Computing thresholds ... : 8/70 [00:04<00:36, 1.69it/s]
13%|##############4 | Computing thresholds ... : 9/70 [00:05<00:36, 1.68it/s]
14%|###############8 | Computing thresholds ... : 10/70 [00:05<00:35, 1.69it/s]
16%|#################4 | Computing thresholds ... : 11/70 [00:06<00:34, 1.69it/s]
17%|################### | Computing thresholds ... : 12/70 [00:07<00:34, 1.68it/s]
19%|####################6 | Computing thresholds ... : 13/70 [00:07<00:33, 1.70it/s]
20%|######################2 | Computing thresholds ... : 14/70 [00:08<00:33, 1.70it/s]
21%|#######################7 | Computing thresholds ... : 15/70 [00:08<00:32, 1.69it/s]
23%|#########################3 | Computing thresholds ... : 16/70 [00:09<00:31, 1.70it/s]
24%|##########################9 | Computing thresholds ... : 17/70 [00:09<00:30, 1.71it/s]
26%|############################5 | Computing thresholds ... : 18/70 [00:10<00:30, 1.73it/s]
27%|##############################1 | Computing thresholds ... : 19/70 [00:11<00:29, 1.73it/s]
29%|###############################7 | Computing thresholds ... : 20/70 [00:11<00:28, 1.73it/s]
30%|#################################3 | Computing thresholds ... : 21/70 [00:12<00:28, 1.73it/s]
31%|##################################8 | Computing thresholds ... : 22/70 [00:12<00:27, 1.73it/s]
33%|####################################4 | Computing thresholds ... : 23/70 [00:13<00:27, 1.73it/s]
34%|###################################### | Computing thresholds ... : 24/70 [00:13<00:26, 1.74it/s]
36%|#######################################6 | Computing thresholds ... : 25/70 [00:14<00:25, 1.73it/s]
37%|#########################################2 | Computing thresholds ... : 26/70 [00:15<00:25, 1.74it/s]
39%|##########################################8 | Computing thresholds ... : 27/70 [00:15<00:24, 1.74it/s]
40%|############################################4 | Computing thresholds ... : 28/70 [00:16<00:24, 1.75it/s]
41%|#############################################9 | Computing thresholds ... : 29/70 [00:16<00:23, 1.75it/s]
43%|###############################################5 | Computing thresholds ... : 30/70 [00:17<00:22, 1.75it/s]
44%|#################################################1 | Computing thresholds ... : 31/70 [00:17<00:22, 1.75it/s]
46%|##################################################7 | Computing thresholds ... : 32/70 [00:18<00:21, 1.75it/s]
47%|####################################################3 | Computing thresholds ... : 33/70 [00:18<00:21, 1.76it/s]
49%|#####################################################9 | Computing thresholds ... : 34/70 [00:19<00:20, 1.76it/s]
50%|#######################################################5 | Computing thresholds ... : 35/70 [00:20<00:19, 1.76it/s]
51%|######################################################### | Computing thresholds ... : 36/70 [00:20<00:19, 1.76it/s]
53%|##########################################################6 | Computing thresholds ... : 37/70 [00:21<00:18, 1.76it/s]
54%|############################################################2 | Computing thresholds ... : 38/70 [00:21<00:18, 1.76it/s]
56%|#############################################################8 | Computing thresholds ... : 39/70 [00:22<00:17, 1.77it/s]
57%|###############################################################4 | Computing thresholds ... : 40/70 [00:22<00:16, 1.77it/s]
59%|################################################################# | Computing thresholds ... : 41/70 [00:23<00:16, 1.77it/s]
60%|##################################################################6 | Computing thresholds ... : 42/70 [00:24<00:15, 1.77it/s]
61%|####################################################################1 | Computing thresholds ... : 43/70 [00:24<00:15, 1.77it/s]
63%|#####################################################################7 | Computing thresholds ... : 44/70 [00:25<00:14, 1.76it/s]
64%|#######################################################################3 | Computing thresholds ... : 45/70 [00:25<00:14, 1.76it/s]
66%|########################################################################9 | Computing thresholds ... : 46/70 [00:26<00:13, 1.76it/s]
67%|##########################################################################5 | Computing thresholds ... : 47/70 [00:26<00:13, 1.76it/s]
69%|############################################################################1 | Computing thresholds ... : 48/70 [00:27<00:12, 1.76it/s]
70%|#############################################################################6 | Computing thresholds ... : 49/70 [00:28<00:11, 1.76it/s]
71%|###############################################################################2 | Computing thresholds ... : 50/70 [00:28<00:11, 1.76it/s]
73%|################################################################################8 | Computing thresholds ... : 51/70 [00:29<00:10, 1.76it/s]
74%|##################################################################################4 | Computing thresholds ... : 52/70 [00:29<00:10, 1.76it/s]
76%|#################################################################################### | Computing thresholds ... : 53/70 [00:30<00:09, 1.76it/s]
77%|#####################################################################################6 | Computing thresholds ... : 54/70 [00:30<00:09, 1.76it/s]
79%|#######################################################################################2 | Computing thresholds ... : 55/70 [00:31<00:08, 1.75it/s]
80%|########################################################################################8 | Computing thresholds ... : 56/70 [00:32<00:07, 1.76it/s]
81%|##########################################################################################3 | Computing thresholds ... : 57/70 [00:32<00:07, 1.76it/s]
83%|###########################################################################################9 | Computing thresholds ... : 58/70 [00:33<00:06, 1.76it/s]
84%|#############################################################################################5 | Computing thresholds ... : 59/70 [00:33<00:06, 1.76it/s]
86%|###############################################################################################1 | Computing thresholds ... : 60/70 [00:34<00:05, 1.76it/s]
87%|################################################################################################7 | Computing thresholds ... : 61/70 [00:34<00:05, 1.75it/s]
89%|##################################################################################################3 | Computing thresholds ... : 62/70 [00:35<00:04, 1.74it/s]
90%|###################################################################################################9 | Computing thresholds ... : 63/70 [00:36<00:04, 1.74it/s]
91%|#####################################################################################################4 | Computing thresholds ... : 64/70 [00:36<00:03, 1.74it/s]
93%|####################################################################################################### | Computing thresholds ... : 65/70 [00:37<00:02, 1.74it/s]
94%|########################################################################################################6 | Computing thresholds ... : 66/70 [00:37<00:02, 1.73it/s]
96%|##########################################################################################################2 | Computing thresholds ... : 67/70 [00:38<00:01, 1.74it/s]
97%|###########################################################################################################8 | Computing thresholds ... : 68/70 [00:39<00:01, 1.74it/s]
99%|#############################################################################################################4 | Computing thresholds ... : 69/70 [00:39<00:00, 1.74it/s]
100%|###############################################################################################################| Computing thresholds ... : 70/70 [00:40<00:00, 1.74it/s]
100%|###############################################################################################################| Computing thresholds ... : 70/70 [00:40<00:00, 1.74it/s]
0%| | Repairing epochs : 0/197 [00:00<?, ?it/s]
2%|#8 | Repairing epochs : 3/197 [00:00<00:01, 153.46it/s]
3%|###6 | Repairing epochs : 6/197 [00:00<00:01, 169.07it/s]
5%|#####9 | Repairing epochs : 10/197 [00:00<00:01, 180.54it/s]
7%|########3 | Repairing epochs : 14/197 [00:00<00:00, 185.80it/s]
9%|##########7 | Repairing epochs : 18/197 [00:00<00:00, 195.71it/s]
11%|#############1 | Repairing epochs : 22/197 [00:00<00:00, 198.48it/s]
13%|###############5 | Repairing epochs : 26/197 [00:00<00:00, 205.87it/s]
15%|#################3 | Repairing epochs : 29/197 [00:00<00:00, 203.40it/s]
17%|###################7 | Repairing epochs : 33/197 [00:00<00:00, 209.05it/s]
19%|######################1 | Repairing epochs : 37/197 [00:00<00:00, 208.42it/s]
21%|########################5 | Repairing epochs : 41/197 [00:00<00:00, 207.30it/s]
23%|##########################9 | Repairing epochs : 45/197 [00:00<00:00, 211.41it/s]
24%|############################7 | Repairing epochs : 48/197 [00:00<00:00, 208.54it/s]
27%|###############################7 | Repairing epochs : 53/197 [00:00<00:00, 211.85it/s]
29%|##################################1 | Repairing epochs : 57/197 [00:00<00:00, 212.02it/s]
31%|####################################5 | Repairing epochs : 61/197 [00:00<00:00, 210.79it/s]
33%|######################################9 | Repairing epochs : 65/197 [00:00<00:00, 213.73it/s]
35%|########################################7 | Repairing epochs : 68/197 [00:00<00:00, 211.76it/s]
37%|###########################################7 | Repairing epochs : 73/197 [00:00<00:00, 211.80it/s]
39%|##############################################1 | Repairing epochs : 77/197 [00:00<00:00, 214.38it/s]
41%|###############################################9 | Repairing epochs : 80/197 [00:00<00:00, 212.53it/s]
43%|##################################################3 | Repairing epochs : 84/197 [00:00<00:00, 214.97it/s]
44%|####################################################1 | Repairing epochs : 87/197 [00:00<00:00, 213.15it/s]
46%|######################################################5 | Repairing epochs : 91/197 [00:00<00:00, 212.08it/s]
48%|########################################################9 | Repairing epochs : 95/197 [00:00<00:00, 214.40it/s]
50%|##########################################################7 | Repairing epochs : 98/197 [00:00<00:00, 212.73it/s]
52%|############################################################5 | Repairing epochs : 102/197 [00:00<00:00, 214.94it/s]
53%|##############################################################3 | Repairing epochs : 105/197 [00:00<00:00, 213.28it/s]
55%|################################################################7 | Repairing epochs : 109/197 [00:00<00:00, 215.42it/s]
57%|###################################################################1 | Repairing epochs : 113/197 [00:00<00:00, 214.64it/s]
59%|#####################################################################4 | Repairing epochs : 117/197 [00:00<00:00, 216.66it/s]
61%|#######################################################################2 | Repairing epochs : 120/197 [00:00<00:00, 214.96it/s]
63%|#########################################################################6 | Repairing epochs : 124/197 [00:00<00:00, 216.92it/s]
64%|###########################################################################4 | Repairing epochs : 127/197 [00:00<00:00, 215.11it/s]
66%|#############################################################################8 | Repairing epochs : 131/197 [00:00<00:00, 217.04it/s]
69%|################################################################################1 | Repairing epochs : 135/197 [00:00<00:00, 218.82it/s]
70%|#################################################################################9 | Repairing epochs : 138/197 [00:00<00:00, 217.06it/s]
72%|####################################################################################3 | Repairing epochs : 142/197 [00:00<00:00, 215.68it/s]
75%|#######################################################################################3 | Repairing epochs : 147/197 [00:00<00:00, 217.95it/s]
77%|#########################################################################################6 | Repairing epochs : 151/197 [00:00<00:00, 216.36it/s]
79%|############################################################################################ | Repairing epochs : 155/197 [00:00<00:00, 217.04it/s]
81%|##############################################################################################4 | Repairing epochs : 159/197 [00:00<00:00, 216.89it/s]
83%|################################################################################################8 | Repairing epochs : 163/197 [00:00<00:00, 218.56it/s]
84%|##################################################################################################5 | Repairing epochs : 166/197 [00:00<00:00, 216.93it/s]
86%|####################################################################################################9 | Repairing epochs : 170/197 [00:00<00:00, 218.59it/s]
88%|#######################################################################################################3 | Repairing epochs : 174/197 [00:00<00:00, 217.10it/s]
90%|#########################################################################################################7 | Repairing epochs : 178/197 [00:00<00:00, 218.39it/s]
92%|############################################################################################################ | Repairing epochs : 182/197 [00:00<00:00, 217.22it/s]
94%|##############################################################################################################4 | Repairing epochs : 186/197 [00:00<00:00, 218.82it/s]
96%|################################################################################################################8 | Repairing epochs : 190/197 [00:00<00:00, 217.63it/s]
98%|###################################################################################################################2 | Repairing epochs : 194/197 [00:00<00:00, 219.19it/s]
100%|#####################################################################################################################| Repairing epochs : 197/197 [00:00<00:00, 216.38it/s]
0%| | n_interp : 0/3 [00:00<?, ?it/s]
0%| | Repairing epochs : 0/197 [00:00<?, ?it/s]
1%|#2 | Repairing epochs : 2/197 [00:00<00:02, 83.12it/s]
2%|#8 | Repairing epochs : 3/197 [00:00<00:02, 74.55it/s]
3%|### | Repairing epochs : 5/197 [00:00<00:02, 73.25it/s]
4%|####2 | Repairing epochs : 7/197 [00:00<00:02, 76.47it/s]
4%|####8 | Repairing epochs : 8/197 [00:00<00:02, 74.02it/s]
5%|#####9 | Repairing epochs : 10/197 [00:00<00:02, 74.32it/s]
6%|#######1 | Repairing epochs : 12/197 [00:00<00:02, 75.02it/s]
7%|########3 | Repairing epochs : 14/197 [00:00<00:02, 76.28it/s]
8%|########9 | Repairing epochs : 15/197 [00:00<00:02, 74.83it/s]
9%|##########1 | Repairing epochs : 17/197 [00:00<00:02, 76.16it/s]
9%|##########7 | Repairing epochs : 18/197 [00:00<00:02, 74.97it/s]
10%|###########9 | Repairing epochs : 20/197 [00:00<00:02, 75.89it/s]
11%|#############1 | Repairing epochs : 22/197 [00:00<00:02, 75.30it/s]
12%|##############3 | Repairing epochs : 24/197 [00:00<00:02, 76.27it/s]
13%|##############9 | Repairing epochs : 25/197 [00:00<00:02, 75.20it/s]
14%|################1 | Repairing epochs : 27/197 [00:00<00:02, 75.81it/s]
15%|#################3 | Repairing epochs : 29/197 [00:00<00:02, 76.31it/s]
16%|##################5 | Repairing epochs : 31/197 [00:00<00:02, 76.92it/s]
16%|###################1 | Repairing epochs : 32/197 [00:00<00:02, 75.79it/s]
17%|####################3 | Repairing epochs : 34/197 [00:00<00:02, 76.66it/s]
18%|####################9 | Repairing epochs : 35/197 [00:00<00:02, 75.86it/s]
19%|######################1 | Repairing epochs : 37/197 [00:00<00:02, 76.19it/s]
20%|#######################3 | Repairing epochs : 39/197 [00:00<00:02, 76.11it/s]
21%|########################5 | Repairing epochs : 41/197 [00:00<00:02, 76.04it/s]
22%|#########################7 | Repairing epochs : 43/197 [00:00<00:02, 76.21it/s]
22%|##########################3 | Repairing epochs : 44/197 [00:00<00:02, 75.53it/s]
23%|###########################5 | Repairing epochs : 46/197 [00:00<00:01, 77.91it/s]
24%|############################7 | Repairing epochs : 48/197 [00:00<00:01, 77.48it/s]
25%|#############################9 | Repairing epochs : 50/197 [00:00<00:01, 77.86it/s]
26%|###############################1 | Repairing epochs : 52/197 [00:00<00:01, 79.12it/s]
27%|################################3 | Repairing epochs : 54/197 [00:00<00:01, 78.51it/s]
28%|#################################5 | Repairing epochs : 56/197 [00:00<00:01, 78.70it/s]
29%|##################################7 | Repairing epochs : 58/197 [00:00<00:01, 78.25it/s]
30%|###################################3 | Repairing epochs : 59/197 [00:00<00:01, 77.57it/s]
31%|####################################5 | Repairing epochs : 61/197 [00:00<00:01, 79.61it/s]
32%|#####################################7 | Repairing epochs : 63/197 [00:00<00:01, 80.69it/s]
33%|######################################9 | Repairing epochs : 65/197 [00:00<00:01, 80.06it/s]
34%|########################################1 | Repairing epochs : 67/197 [00:00<00:01, 79.43it/s]
35%|########################################7 | Repairing epochs : 68/197 [00:00<00:01, 78.58it/s]
36%|#########################################9 | Repairing epochs : 70/197 [00:00<00:01, 80.09it/s]
37%|###########################################7 | Repairing epochs : 73/197 [00:00<00:01, 81.38it/s]
38%|############################################9 | Repairing epochs : 75/197 [00:00<00:01, 80.78it/s]
39%|##############################################1 | Repairing epochs : 77/197 [00:00<00:01, 80.42it/s]
40%|###############################################3 | Repairing epochs : 79/197 [00:01<00:01, 80.27it/s]
41%|################################################5 | Repairing epochs : 81/197 [00:01<00:01, 79.76it/s]
42%|#################################################7 | Repairing epochs : 83/197 [00:01<00:01, 79.95it/s]
43%|##################################################3 | Repairing epochs : 84/197 [00:01<00:01, 79.28it/s]
44%|###################################################5 | Repairing epochs : 86/197 [00:01<00:01, 79.50it/s]
45%|####################################################7 | Repairing epochs : 88/197 [00:01<00:01, 78.91it/s]
46%|######################################################5 | Repairing epochs : 91/197 [00:01<00:01, 82.30it/s]
47%|#######################################################1 | Repairing epochs : 92/197 [00:01<00:01, 81.55it/s]
48%|########################################################3 | Repairing epochs : 94/197 [00:01<00:01, 80.87it/s]
49%|#########################################################5 | Repairing epochs : 96/197 [00:01<00:01, 80.81it/s]
50%|##########################################################7 | Repairing epochs : 98/197 [00:01<00:01, 80.39it/s]
51%|###########################################################3 | Repairing epochs : 100/197 [00:01<00:01, 79.88it/s]
52%|############################################################5 | Repairing epochs : 102/197 [00:01<00:01, 80.05it/s]
53%|#############################################################7 | Repairing epochs : 104/197 [00:01<00:01, 80.94it/s]
54%|##############################################################9 | Repairing epochs : 106/197 [00:01<00:01, 80.26it/s]
55%|################################################################7 | Repairing epochs : 109/197 [00:01<00:01, 81.23it/s]
56%|#################################################################9 | Repairing epochs : 111/197 [00:01<00:01, 81.38it/s]
57%|##################################################################5 | Repairing epochs : 112/197 [00:01<00:01, 80.71it/s]
57%|###################################################################1 | Repairing epochs : 113/197 [00:01<00:01, 80.05it/s]
58%|####################################################################2 | Repairing epochs : 115/197 [00:01<00:01, 80.12it/s]
59%|#####################################################################4 | Repairing epochs : 117/197 [00:01<00:00, 81.64it/s]
60%|###################################################################### | Repairing epochs : 118/197 [00:01<00:00, 80.88it/s]
61%|#######################################################################2 | Repairing epochs : 120/197 [00:01<00:00, 80.36it/s]
62%|########################################################################4 | Repairing epochs : 122/197 [00:01<00:00, 79.80it/s]
63%|#########################################################################6 | Repairing epochs : 124/197 [00:01<00:00, 79.97it/s]
63%|##########################################################################2 | Repairing epochs : 125/197 [00:01<00:00, 79.32it/s]
65%|############################################################################ | Repairing epochs : 128/197 [00:01<00:00, 82.49it/s]
65%|############################################################################6 | Repairing epochs : 129/197 [00:01<00:00, 81.75it/s]
66%|#############################################################################8 | Repairing epochs : 131/197 [00:01<00:00, 81.87it/s]
68%|##############################################################################9 | Repairing epochs : 133/197 [00:01<00:00, 81.19it/s]
69%|################################################################################1 | Repairing epochs : 135/197 [00:01<00:00, 81.39it/s]
70%|#################################################################################3 | Repairing epochs : 137/197 [00:01<00:00, 80.69it/s]
71%|##################################################################################5 | Repairing epochs : 139/197 [00:01<00:00, 80.64it/s]
72%|###################################################################################7 | Repairing epochs : 141/197 [00:01<00:00, 80.33it/s]
73%|####################################################################################9 | Repairing epochs : 143/197 [00:01<00:00, 79.74it/s]
74%|######################################################################################1 | Repairing epochs : 145/197 [00:01<00:00, 79.92it/s]
74%|######################################################################################7 | Repairing epochs : 146/197 [00:01<00:00, 79.13it/s]
75%|#######################################################################################8 | Repairing epochs : 148/197 [00:01<00:00, 80.30it/s]
76%|######################################################################################### | Repairing epochs : 150/197 [00:01<00:00, 79.74it/s]
77%|##########################################################################################2 | Repairing epochs : 152/197 [00:01<00:00, 80.62it/s]
78%|###########################################################################################4 | Repairing epochs : 154/197 [00:01<00:00, 81.40it/s]
80%|#############################################################################################2 | Repairing epochs : 157/197 [00:01<00:00, 82.33it/s]
81%|##############################################################################################4 | Repairing epochs : 159/197 [00:01<00:00, 81.67it/s]
82%|###############################################################################################6 | Repairing epochs : 161/197 [00:02<00:00, 81.04it/s]
84%|#################################################################################################9 | Repairing epochs : 165/197 [00:02<00:00, 85.37it/s]
84%|##################################################################################################5 | Repairing epochs : 166/197 [00:02<00:00, 84.54it/s]
85%|###################################################################################################7 | Repairing epochs : 168/197 [00:02<00:00, 83.76it/s]
86%|####################################################################################################9 | Repairing epochs : 170/197 [00:02<00:00, 83.09it/s]
87%|######################################################################################################1 | Repairing epochs : 172/197 [00:02<00:00, 82.40it/s]
88%|#######################################################################################################3 | Repairing epochs : 174/197 [00:02<00:00, 81.73it/s]
89%|########################################################################################################5 | Repairing epochs : 176/197 [00:02<00:00, 80.49it/s]
90%|#########################################################################################################7 | Repairing epochs : 178/197 [00:02<00:00, 79.98it/s]
91%|##########################################################################################################9 | Repairing epochs : 180/197 [00:02<00:00, 79.49it/s]
93%|############################################################################################################6 | Repairing epochs : 183/197 [00:02<00:00, 81.06it/s]
93%|#############################################################################################################2 | Repairing epochs : 184/197 [00:02<00:00, 80.45it/s]
94%|##############################################################################################################4 | Repairing epochs : 186/197 [00:02<00:00, 80.57it/s]
95%|###############################################################################################################6 | Repairing epochs : 188/197 [00:02<00:00, 79.88it/s]
97%|#################################################################################################################4 | Repairing epochs : 191/197 [00:02<00:00, 80.99it/s]
98%|##################################################################################################################6 | Repairing epochs : 193/197 [00:02<00:00, 81.09it/s]
98%|###################################################################################################################2 | Repairing epochs : 194/197 [00:02<00:00, 80.48it/s]
99%|###################################################################################################################8 | Repairing epochs : 195/197 [00:02<00:00, 79.88it/s]
100%|#####################################################################################################################| Repairing epochs : 197/197 [00:02<00:00, 79.37it/s]
100%|#####################################################################################################################| Repairing epochs : 197/197 [00:02<00:00, 79.81it/s]
0%| | Fold : 0/10 [00:00<?, ?it/s]
10%|#############2 | Fold : 1/10 [00:00<00:03, 2.74it/s]
20%|##########################4 | Fold : 2/10 [00:00<00:02, 2.70it/s]
30%|#######################################6 | Fold : 3/10 [00:01<00:02, 2.72it/s]
40%|####################################################8 | Fold : 4/10 [00:01<00:02, 2.72it/s]
50%|################################################################## | Fold : 5/10 [00:01<00:01, 2.71it/s]
60%|###############################################################################2 | Fold : 6/10 [00:02<00:01, 2.72it/s]
70%|############################################################################################3 | Fold : 7/10 [00:02<00:01, 2.69it/s]
80%|#########################################################################################################6 | Fold : 8/10 [00:02<00:00, 2.69it/s]
90%|######################################################################################################################8 | Fold : 9/10 [00:03<00:00, 2.70it/s]
100%|###################################################################################################################################| Fold : 10/10 [00:03<00:00, 2.69it/s]
100%|###################################################################################################################################| Fold : 10/10 [00:03<00:00, 2.69it/s]
33%|########################################### | n_interp : 1/3 [00:06<00:12, 6.48s/it]
0%| | Repairing epochs : 0/197 [00:00<?, ?it/s]
1%|#2 | Repairing epochs : 2/197 [00:00<00:02, 81.08it/s]
2%|#8 | Repairing epochs : 3/197 [00:00<00:02, 70.78it/s]
3%|### | Repairing epochs : 5/197 [00:00<00:02, 77.11it/s]
3%|###6 | Repairing epochs : 6/197 [00:00<00:02, 73.96it/s]
4%|####2 | Repairing epochs : 7/197 [00:00<00:02, 71.81it/s]
5%|#####4 | Repairing epochs : 9/197 [00:00<00:02, 74.33it/s]
5%|#####9 | Repairing epochs : 10/197 [00:00<00:02, 71.77it/s]
6%|#######1 | Repairing epochs : 12/197 [00:00<00:02, 74.38it/s]
7%|#######7 | Repairing epochs : 13/197 [00:00<00:02, 72.89it/s]
8%|########9 | Repairing epochs : 15/197 [00:00<00:02, 72.39it/s]
9%|##########1 | Repairing epochs : 17/197 [00:00<00:02, 75.66it/s]
9%|##########7 | Repairing epochs : 18/197 [00:00<00:02, 73.30it/s]
10%|###########9 | Repairing epochs : 20/197 [00:00<00:02, 75.45it/s]
11%|############5 | Repairing epochs : 21/197 [00:00<00:02, 72.59it/s]
12%|#############7 | Repairing epochs : 23/197 [00:00<00:02, 72.83it/s]
13%|##############9 | Repairing epochs : 25/197 [00:00<00:02, 73.10it/s]
14%|################1 | Repairing epochs : 27/197 [00:00<00:02, 73.47it/s]
15%|#################3 | Repairing epochs : 29/197 [00:00<00:02, 73.51it/s]
16%|##################5 | Repairing epochs : 31/197 [00:00<00:02, 74.31it/s]
16%|###################1 | Repairing epochs : 32/197 [00:00<00:02, 73.63it/s]
17%|####################3 | Repairing epochs : 34/197 [00:00<00:02, 74.45it/s]
18%|####################9 | Repairing epochs : 35/197 [00:00<00:02, 73.70it/s]
19%|######################1 | Repairing epochs : 37/197 [00:00<00:02, 73.96it/s]
20%|#######################3 | Repairing epochs : 39/197 [00:00<00:02, 74.14it/s]
21%|########################5 | Repairing epochs : 41/197 [00:00<00:02, 74.12it/s]
22%|#########################7 | Repairing epochs : 43/197 [00:00<00:02, 74.63it/s]
23%|##########################9 | Repairing epochs : 45/197 [00:00<00:02, 74.14it/s]
24%|############################1 | Repairing epochs : 47/197 [00:00<00:01, 75.88it/s]
25%|#############################3 | Repairing epochs : 49/197 [00:00<00:01, 76.47it/s]
25%|#############################9 | Repairing epochs : 50/197 [00:00<00:01, 75.73it/s]
27%|###############################7 | Repairing epochs : 53/197 [00:00<00:01, 77.22it/s]
28%|################################9 | Repairing epochs : 55/197 [00:00<00:01, 77.19it/s]
29%|##################################1 | Repairing epochs : 57/197 [00:00<00:01, 76.05it/s]
30%|###################################3 | Repairing epochs : 59/197 [00:00<00:01, 75.43it/s]
31%|####################################5 | Repairing epochs : 61/197 [00:00<00:01, 76.65it/s]
32%|######################################3 | Repairing epochs : 64/197 [00:00<00:01, 78.64it/s]
34%|#######################################5 | Repairing epochs : 66/197 [00:00<00:01, 78.02it/s]
35%|########################################7 | Repairing epochs : 68/197 [00:00<00:01, 77.69it/s]
37%|###########################################1 | Repairing epochs : 72/197 [00:00<00:01, 81.21it/s]
38%|############################################3 | Repairing epochs : 74/197 [00:00<00:01, 80.93it/s]
39%|#############################################5 | Repairing epochs : 76/197 [00:00<00:01, 80.05it/s]
40%|##############################################7 | Repairing epochs : 78/197 [00:01<00:01, 80.31it/s]
40%|###############################################3 | Repairing epochs : 79/197 [00:01<00:01, 79.65it/s]
41%|################################################5 | Repairing epochs : 81/197 [00:01<00:01, 79.31it/s]
42%|#################################################7 | Repairing epochs : 83/197 [00:01<00:01, 79.88it/s]
43%|##################################################3 | Repairing epochs : 84/197 [00:01<00:01, 79.24it/s]
43%|##################################################9 | Repairing epochs : 85/197 [00:01<00:01, 78.62it/s]
44%|####################################################1 | Repairing epochs : 87/197 [00:01<00:01, 78.95it/s]
45%|#####################################################3 | Repairing epochs : 89/197 [00:01<00:01, 80.28it/s]
46%|######################################################5 | Repairing epochs : 91/197 [00:01<00:01, 80.91it/s]
47%|#######################################################7 | Repairing epochs : 93/197 [00:01<00:01, 80.99it/s]
48%|########################################################9 | Repairing epochs : 95/197 [00:01<00:01, 80.19it/s]
49%|##########################################################1 | Repairing epochs : 97/197 [00:01<00:01, 80.89it/s]
50%|###########################################################2 | Repairing epochs : 99/197 [00:01<00:01, 79.99it/s]
51%|###########################################################9 | Repairing epochs : 101/197 [00:01<00:01, 80.24it/s]
52%|############################################################5 | Repairing epochs : 102/197 [00:01<00:01, 79.44it/s]
53%|#############################################################7 | Repairing epochs : 104/197 [00:01<00:01, 81.10it/s]
53%|##############################################################3 | Repairing epochs : 105/197 [00:01<00:01, 80.35it/s]
54%|###############################################################5 | Repairing epochs : 107/197 [00:01<00:01, 82.01it/s]
55%|################################################################1 | Repairing epochs : 108/197 [00:01<00:01, 81.23it/s]
56%|#################################################################3 | Repairing epochs : 110/197 [00:01<00:01, 81.15it/s]
57%|##################################################################5 | Repairing epochs : 112/197 [00:01<00:01, 81.45it/s]
57%|###################################################################1 | Repairing epochs : 113/197 [00:01<00:01, 79.96it/s]
58%|####################################################################2 | Repairing epochs : 115/197 [00:01<00:01, 79.40it/s]
59%|#####################################################################4 | Repairing epochs : 117/197 [00:01<00:00, 81.00it/s]
60%|######################################################################6 | Repairing epochs : 119/197 [00:01<00:00, 81.02it/s]
61%|#######################################################################2 | Repairing epochs : 120/197 [00:01<00:00, 80.32it/s]
62%|########################################################################4 | Repairing epochs : 122/197 [00:01<00:00, 80.39it/s]
63%|#########################################################################6 | Repairing epochs : 124/197 [00:01<00:00, 79.67it/s]
64%|##########################################################################8 | Repairing epochs : 126/197 [00:01<00:00, 80.80it/s]
65%|############################################################################ | Repairing epochs : 128/197 [00:01<00:00, 82.36it/s]
66%|#############################################################################2 | Repairing epochs : 130/197 [00:01<00:00, 81.55it/s]
67%|##############################################################################3 | Repairing epochs : 132/197 [00:01<00:00, 82.21it/s]
68%|###############################################################################5 | Repairing epochs : 134/197 [00:01<00:00, 80.76it/s]
69%|################################################################################7 | Repairing epochs : 136/197 [00:01<00:00, 81.11it/s]
70%|#################################################################################3 | Repairing epochs : 137/197 [00:01<00:00, 79.70it/s]
71%|##################################################################################5 | Repairing epochs : 139/197 [00:01<00:00, 79.17it/s]
72%|###################################################################################7 | Repairing epochs : 141/197 [00:01<00:00, 79.20it/s]
72%|####################################################################################3 | Repairing epochs : 142/197 [00:01<00:00, 78.54it/s]
73%|#####################################################################################5 | Repairing epochs : 144/197 [00:01<00:00, 78.87it/s]
74%|######################################################################################7 | Repairing epochs : 146/197 [00:01<00:00, 79.10it/s]
75%|#######################################################################################8 | Repairing epochs : 148/197 [00:01<00:00, 80.66it/s]
76%|######################################################################################### | Repairing epochs : 150/197 [00:01<00:00, 79.32it/s]
77%|##########################################################################################2 | Repairing epochs : 152/197 [00:01<00:00, 80.94it/s]
78%|###########################################################################################4 | Repairing epochs : 154/197 [00:01<00:00, 82.54it/s]
79%|############################################################################################ | Repairing epochs : 155/197 [00:01<00:00, 81.79it/s]
80%|#############################################################################################2 | Repairing epochs : 157/197 [00:01<00:00, 83.39it/s]
80%|#############################################################################################8 | Repairing epochs : 158/197 [00:01<00:00, 82.59it/s]
81%|############################################################################################### | Repairing epochs : 160/197 [00:02<00:00, 82.62it/s]
82%|###############################################################################################6 | Repairing epochs : 161/197 [00:02<00:00, 81.85it/s]
84%|#################################################################################################9 | Repairing epochs : 165/197 [00:02<00:00, 86.64it/s]
85%|###################################################################################################1 | Repairing epochs : 167/197 [00:02<00:00, 86.44it/s]
85%|###################################################################################################7 | Repairing epochs : 168/197 [00:02<00:00, 85.22it/s]
86%|####################################################################################################9 | Repairing epochs : 170/197 [00:02<00:00, 85.39it/s]
87%|#####################################################################################################5 | Repairing epochs : 171/197 [00:02<00:00, 84.51it/s]
88%|######################################################################################################7 | Repairing epochs : 173/197 [00:02<00:00, 84.23it/s]
89%|#######################################################################################################9 | Repairing epochs : 175/197 [00:02<00:00, 84.21it/s]
89%|########################################################################################################5 | Repairing epochs : 176/197 [00:02<00:00, 82.79it/s]
90%|#########################################################################################################7 | Repairing epochs : 178/197 [00:02<00:00, 83.40it/s]
91%|##########################################################################################################9 | Repairing epochs : 180/197 [00:02<00:00, 81.80it/s]
92%|############################################################################################################ | Repairing epochs : 182/197 [00:02<00:00, 83.33it/s]
93%|#############################################################################################################2 | Repairing epochs : 184/197 [00:02<00:00, 82.92it/s]
94%|##############################################################################################################4 | Repairing epochs : 186/197 [00:02<00:00, 83.49it/s]
95%|############################################################################################################### | Repairing epochs : 187/197 [00:02<00:00, 81.67it/s]
96%|################################################################################################################2 | Repairing epochs : 189/197 [00:02<00:00, 82.64it/s]
97%|#################################################################################################################4 | Repairing epochs : 191/197 [00:02<00:00, 82.76it/s]
97%|################################################################################################################## | Repairing epochs : 192/197 [00:02<00:00, 81.95it/s]
98%|###################################################################################################################2 | Repairing epochs : 194/197 [00:02<00:00, 81.59it/s]
99%|####################################################################################################################4| Repairing epochs : 196/197 [00:02<00:00, 81.29it/s]
100%|#####################################################################################################################| Repairing epochs : 197/197 [00:02<00:00, 80.13it/s]
0%| | Fold : 0/10 [00:00<?, ?it/s]
10%|#############2 | Fold : 1/10 [00:00<00:03, 2.78it/s]
20%|##########################4 | Fold : 2/10 [00:00<00:02, 2.78it/s]
30%|#######################################6 | Fold : 3/10 [00:01<00:02, 2.79it/s]
40%|####################################################8 | Fold : 4/10 [00:01<00:02, 2.78it/s]
50%|################################################################## | Fold : 5/10 [00:01<00:01, 2.78it/s]
60%|###############################################################################2 | Fold : 6/10 [00:02<00:01, 2.78it/s]
70%|############################################################################################3 | Fold : 7/10 [00:02<00:01, 2.78it/s]
80%|#########################################################################################################6 | Fold : 8/10 [00:02<00:00, 2.79it/s]
90%|######################################################################################################################8 | Fold : 9/10 [00:03<00:00, 2.79it/s]
100%|###################################################################################################################################| Fold : 10/10 [00:03<00:00, 2.78it/s]
100%|###################################################################################################################################| Fold : 10/10 [00:03<00:00, 2.78it/s]
67%|###################################################################################### | n_interp : 2/3 [00:12<00:06, 6.39s/it]
0%| | Repairing epochs : 0/197 [00:00<?, ?it/s]
1%|#2 | Repairing epochs : 2/197 [00:00<00:02, 82.41it/s]
2%|##4 | Repairing epochs : 4/197 [00:00<00:02, 83.66it/s]
3%|### | Repairing epochs : 5/197 [00:00<00:02, 78.01it/s]
4%|####2 | Repairing epochs : 7/197 [00:00<00:02, 79.41it/s]
4%|####8 | Repairing epochs : 8/197 [00:00<00:02, 76.50it/s]
5%|#####9 | Repairing epochs : 10/197 [00:00<00:02, 77.57it/s]
6%|#######1 | Repairing epochs : 12/197 [00:00<00:02, 76.55it/s]
7%|########3 | Repairing epochs : 14/197 [00:00<00:02, 77.08it/s]
8%|#########5 | Repairing epochs : 16/197 [00:00<00:02, 78.40it/s]
9%|##########7 | Repairing epochs : 18/197 [00:00<00:02, 79.23it/s]
10%|###########9 | Repairing epochs : 20/197 [00:00<00:02, 78.13it/s]
11%|#############1 | Repairing epochs : 22/197 [00:00<00:02, 79.56it/s]
12%|##############3 | Repairing epochs : 24/197 [00:00<00:02, 79.04it/s]
13%|###############5 | Repairing epochs : 26/197 [00:00<00:02, 79.44it/s]
14%|################7 | Repairing epochs : 28/197 [00:00<00:02, 78.61it/s]
15%|#################9 | Repairing epochs : 30/197 [00:00<00:02, 79.01it/s]
16%|###################1 | Repairing epochs : 32/197 [00:00<00:02, 79.08it/s]
17%|####################3 | Repairing epochs : 34/197 [00:00<00:02, 78.67it/s]
18%|#####################5 | Repairing epochs : 36/197 [00:00<00:02, 79.03it/s]
19%|######################7 | Repairing epochs : 38/197 [00:00<00:02, 78.29it/s]
20%|#######################9 | Repairing epochs : 40/197 [00:00<00:01, 78.65it/s]
21%|#########################1 | Repairing epochs : 42/197 [00:00<00:01, 78.75it/s]
22%|##########################3 | Repairing epochs : 44/197 [00:00<00:01, 78.42it/s]
23%|###########################5 | Repairing epochs : 46/197 [00:00<00:01, 79.77it/s]
24%|############################7 | Repairing epochs : 48/197 [00:00<00:01, 80.00it/s]
25%|#############################9 | Repairing epochs : 50/197 [00:00<00:01, 79.32it/s]
26%|###############################1 | Repairing epochs : 52/197 [00:00<00:01, 80.78it/s]
27%|################################3 | Repairing epochs : 54/197 [00:00<00:01, 81.49it/s]
28%|################################9 | Repairing epochs : 55/197 [00:00<00:01, 80.60it/s]
29%|##################################1 | Repairing epochs : 57/197 [00:00<00:01, 81.76it/s]
29%|##################################7 | Repairing epochs : 58/197 [00:00<00:01, 80.91it/s]
30%|###################################9 | Repairing epochs : 60/197 [00:00<00:01, 82.77it/s]
31%|#####################################1 | Repairing epochs : 62/197 [00:00<00:01, 84.41it/s]
32%|######################################3 | Repairing epochs : 64/197 [00:00<00:01, 83.72it/s]
34%|#######################################5 | Repairing epochs : 66/197 [00:00<00:01, 83.13it/s]
35%|########################################7 | Repairing epochs : 68/197 [00:00<00:01, 82.74it/s]
35%|#########################################3 | Repairing epochs : 69/197 [00:00<00:01, 81.89it/s]
37%|###########################################1 | Repairing epochs : 72/197 [00:00<00:01, 84.65it/s]
38%|############################################3 | Repairing epochs : 74/197 [00:00<00:01, 84.56it/s]
38%|############################################9 | Repairing epochs : 75/197 [00:00<00:01, 83.64it/s]
39%|##############################################1 | Repairing epochs : 77/197 [00:00<00:01, 83.59it/s]
40%|###############################################3 | Repairing epochs : 79/197 [00:00<00:01, 82.82it/s]
41%|################################################5 | Repairing epochs : 81/197 [00:00<00:01, 82.84it/s]
42%|#################################################7 | Repairing epochs : 83/197 [00:01<00:01, 82.85it/s]
43%|##################################################3 | Repairing epochs : 84/197 [00:01<00:01, 82.01it/s]
44%|###################################################5 | Repairing epochs : 86/197 [00:01<00:01, 81.97it/s]
45%|####################################################7 | Repairing epochs : 88/197 [00:01<00:01, 81.35it/s]
46%|######################################################5 | Repairing epochs : 91/197 [00:01<00:01, 84.75it/s]
47%|#######################################################7 | Repairing epochs : 93/197 [00:01<00:01, 83.85it/s]
48%|########################################################9 | Repairing epochs : 95/197 [00:01<00:01, 83.81it/s]
49%|##########################################################1 | Repairing epochs : 97/197 [00:01<00:01, 83.77it/s]
50%|###########################################################2 | Repairing epochs : 99/197 [00:01<00:01, 83.44it/s]
51%|###########################################################9 | Repairing epochs : 101/197 [00:01<00:01, 82.95it/s]
52%|#############################################################1 | Repairing epochs : 103/197 [00:01<00:01, 84.51it/s]
53%|#############################################################7 | Repairing epochs : 104/197 [00:01<00:01, 83.59it/s]
54%|##############################################################9 | Repairing epochs : 106/197 [00:01<00:01, 83.19it/s]
55%|################################################################1 | Repairing epochs : 108/197 [00:01<00:01, 84.26it/s]
56%|#################################################################3 | Repairing epochs : 110/197 [00:01<00:01, 83.41it/s]
57%|##################################################################5 | Repairing epochs : 112/197 [00:01<00:01, 82.64it/s]
58%|###################################################################7 | Repairing epochs : 114/197 [00:01<00:01, 81.91it/s]
59%|####################################################################8 | Repairing epochs : 116/197 [00:01<00:00, 81.80it/s]
60%|###################################################################### | Repairing epochs : 118/197 [00:01<00:00, 83.31it/s]
61%|#######################################################################2 | Repairing epochs : 120/197 [00:01<00:00, 82.56it/s]
62%|########################################################################4 | Repairing epochs : 122/197 [00:01<00:00, 82.58it/s]
63%|#########################################################################6 | Repairing epochs : 124/197 [00:01<00:00, 82.61it/s]
64%|##########################################################################8 | Repairing epochs : 126/197 [00:01<00:00, 83.46it/s]
65%|############################################################################ | Repairing epochs : 128/197 [00:01<00:00, 84.86it/s]
66%|#############################################################################2 | Repairing epochs : 130/197 [00:01<00:00, 84.76it/s]
67%|##############################################################################3 | Repairing epochs : 132/197 [00:01<00:00, 84.74it/s]
68%|##############################################################################9 | Repairing epochs : 133/197 [00:01<00:00, 83.93it/s]
69%|################################################################################1 | Repairing epochs : 135/197 [00:01<00:00, 83.87it/s]
70%|#################################################################################3 | Repairing epochs : 137/197 [00:01<00:00, 83.93it/s]
70%|#################################################################################9 | Repairing epochs : 138/197 [00:01<00:00, 83.13it/s]
71%|###################################################################################1 | Repairing epochs : 140/197 [00:01<00:00, 83.13it/s]
72%|####################################################################################3 | Repairing epochs : 142/197 [00:01<00:00, 83.14it/s]
73%|####################################################################################9 | Repairing epochs : 143/197 [00:01<00:00, 82.37it/s]
74%|######################################################################################1 | Repairing epochs : 145/197 [00:01<00:00, 82.38it/s]
75%|#######################################################################################8 | Repairing epochs : 148/197 [00:01<00:00, 83.74it/s]
76%|######################################################################################### | Repairing epochs : 150/197 [00:01<00:00, 83.24it/s]
77%|##########################################################################################2 | Repairing epochs : 152/197 [00:01<00:00, 84.65it/s]
78%|###########################################################################################4 | Repairing epochs : 154/197 [00:01<00:00, 85.14it/s]
80%|#############################################################################################2 | Repairing epochs : 157/197 [00:01<00:00, 86.79it/s]
80%|#############################################################################################8 | Repairing epochs : 158/197 [00:01<00:00, 85.80it/s]
81%|############################################################################################### | Repairing epochs : 160/197 [00:01<00:00, 85.76it/s]
82%|###############################################################################################6 | Repairing epochs : 161/197 [00:01<00:00, 84.89it/s]
84%|#################################################################################################9 | Repairing epochs : 165/197 [00:01<00:00, 89.35it/s]
85%|###################################################################################################1 | Repairing epochs : 167/197 [00:01<00:00, 88.98it/s]
86%|####################################################################################################3 | Repairing epochs : 169/197 [00:02<00:00, 87.92it/s]
87%|#####################################################################################################5 | Repairing epochs : 171/197 [00:02<00:00, 86.90it/s]
88%|######################################################################################################7 | Repairing epochs : 173/197 [00:02<00:00, 86.23it/s]
89%|#######################################################################################################9 | Repairing epochs : 175/197 [00:02<00:00, 85.83it/s]
90%|#########################################################################################################1 | Repairing epochs : 177/197 [00:02<00:00, 84.95it/s]
91%|##########################################################################################################3 | Repairing epochs : 179/197 [00:02<00:00, 84.84it/s]
92%|###########################################################################################################4 | Repairing epochs : 181/197 [00:02<00:00, 84.02it/s]
93%|#############################################################################################################2 | Repairing epochs : 184/197 [00:02<00:00, 85.45it/s]
94%|##############################################################################################################4 | Repairing epochs : 186/197 [00:02<00:00, 85.33it/s]
95%|###############################################################################################################6 | Repairing epochs : 188/197 [00:02<00:00, 85.13it/s]
96%|################################################################################################################8 | Repairing epochs : 190/197 [00:02<00:00, 85.66it/s]
97%|################################################################################################################## | Repairing epochs : 192/197 [00:02<00:00, 85.48it/s]
98%|###################################################################################################################2 | Repairing epochs : 194/197 [00:02<00:00, 85.36it/s]
99%|####################################################################################################################4| Repairing epochs : 196/197 [00:02<00:00, 85.33it/s]
100%|#####################################################################################################################| Repairing epochs : 197/197 [00:02<00:00, 84.54it/s]
100%|#####################################################################################################################| Repairing epochs : 197/197 [00:02<00:00, 83.47it/s]
0%| | Fold : 0/10 [00:00<?, ?it/s]
10%|#############2 | Fold : 1/10 [00:00<00:02, 4.46it/s]
20%|##########################4 | Fold : 2/10 [00:00<00:01, 4.40it/s]
30%|#######################################6 | Fold : 3/10 [00:00<00:01, 4.38it/s]
40%|####################################################8 | Fold : 4/10 [00:00<00:01, 4.41it/s]
50%|################################################################## | Fold : 5/10 [00:01<00:01, 4.42it/s]
60%|###############################################################################2 | Fold : 6/10 [00:01<00:00, 4.43it/s]
70%|############################################################################################3 | Fold : 7/10 [00:01<00:00, 4.42it/s]
80%|#########################################################################################################6 | Fold : 8/10 [00:01<00:00, 4.43it/s]
90%|######################################################################################################################8 | Fold : 9/10 [00:02<00:00, 4.43it/s]
100%|###################################################################################################################################| Fold : 10/10 [00:02<00:00, 4.44it/s]
100%|###################################################################################################################################| Fold : 10/10 [00:02<00:00, 4.43it/s]
100%|#################################################################################################################################| n_interp : 3/3 [00:17<00:00, 5.80s/it]
100%|#################################################################################################################################| n_interp : 3/3 [00:17<00:00, 5.83s/it]
Estimated consensus=1.00 and n_interpolate=32
0%| | Repairing epochs : 0/197 [00:00<?, ?it/s]
1%|6 | Repairing epochs : 1/197 [00:00<00:03, 62.39it/s]
2%|#8 | Repairing epochs : 3/197 [00:00<00:02, 74.96it/s]
2%|##4 | Repairing epochs : 4/197 [00:00<00:02, 71.18it/s]
3%|###6 | Repairing epochs : 6/197 [00:00<00:02, 74.00it/s]
4%|####8 | Repairing epochs : 8/197 [00:00<00:02, 74.05it/s]
5%|#####9 | Repairing epochs : 10/197 [00:00<00:02, 73.69it/s]
6%|#######1 | Repairing epochs : 12/197 [00:00<00:02, 75.06it/s]
7%|########3 | Repairing epochs : 14/197 [00:00<00:02, 76.03it/s]
8%|#########5 | Repairing epochs : 16/197 [00:00<00:02, 77.01it/s]
9%|##########7 | Repairing epochs : 18/197 [00:00<00:02, 77.75it/s]
10%|###########9 | Repairing epochs : 20/197 [00:00<00:02, 76.87it/s]
11%|#############1 | Repairing epochs : 22/197 [00:00<00:02, 78.07it/s]
12%|##############3 | Repairing epochs : 24/197 [00:00<00:02, 78.09it/s]
13%|###############5 | Repairing epochs : 26/197 [00:00<00:02, 78.58it/s]
14%|################7 | Repairing epochs : 28/197 [00:00<00:02, 77.94it/s]
15%|#################9 | Repairing epochs : 30/197 [00:00<00:02, 78.39it/s]
16%|###################1 | Repairing epochs : 32/197 [00:00<00:02, 78.80it/s]
17%|####################3 | Repairing epochs : 34/197 [00:00<00:02, 79.02it/s]
18%|#####################5 | Repairing epochs : 36/197 [00:00<00:02, 78.44it/s]
19%|######################7 | Repairing epochs : 38/197 [00:00<00:02, 78.55it/s]
20%|#######################9 | Repairing epochs : 40/197 [00:00<00:02, 78.17it/s]
21%|#########################1 | Repairing epochs : 42/197 [00:00<00:01, 78.53it/s]
22%|##########################3 | Repairing epochs : 44/197 [00:00<00:01, 77.98it/s]
23%|###########################5 | Repairing epochs : 46/197 [00:00<00:01, 79.91it/s]
24%|############################7 | Repairing epochs : 48/197 [00:00<00:01, 80.36it/s]
25%|#############################3 | Repairing epochs : 49/197 [00:00<00:01, 79.53it/s]
26%|###############################1 | Repairing epochs : 52/197 [00:00<00:01, 81.67it/s]
27%|################################3 | Repairing epochs : 54/197 [00:00<00:01, 80.66it/s]
28%|#################################5 | Repairing epochs : 56/197 [00:00<00:01, 80.51it/s]
29%|##################################7 | Repairing epochs : 58/197 [00:00<00:01, 80.67it/s]
30%|###################################9 | Repairing epochs : 60/197 [00:00<00:01, 81.77it/s]
31%|#####################################1 | Repairing epochs : 62/197 [00:00<00:01, 83.58it/s]
32%|#####################################7 | Repairing epochs : 63/197 [00:00<00:01, 82.67it/s]
33%|######################################9 | Repairing epochs : 65/197 [00:00<00:01, 82.17it/s]
34%|########################################1 | Repairing epochs : 67/197 [00:00<00:01, 81.91it/s]
35%|#########################################3 | Repairing epochs : 69/197 [00:00<00:01, 82.82it/s]
37%|###########################################1 | Repairing epochs : 72/197 [00:00<00:01, 85.27it/s]
38%|############################################3 | Repairing epochs : 74/197 [00:00<00:01, 84.75it/s]
39%|#############################################5 | Repairing epochs : 76/197 [00:00<00:01, 84.29it/s]
39%|##############################################1 | Repairing epochs : 77/197 [00:00<00:01, 83.22it/s]
40%|###############################################3 | Repairing epochs : 79/197 [00:00<00:01, 82.70it/s]
41%|################################################5 | Repairing epochs : 81/197 [00:00<00:01, 82.72it/s]
42%|#################################################7 | Repairing epochs : 83/197 [00:01<00:01, 82.75it/s]
43%|##################################################9 | Repairing epochs : 85/197 [00:01<00:01, 82.74it/s]
44%|###################################################5 | Repairing epochs : 86/197 [00:01<00:01, 81.86it/s]
45%|####################################################7 | Repairing epochs : 88/197 [00:01<00:01, 81.82it/s]
46%|######################################################5 | Repairing epochs : 91/197 [00:01<00:01, 84.26it/s]
47%|#######################################################7 | Repairing epochs : 93/197 [00:01<00:01, 84.19it/s]
48%|########################################################9 | Repairing epochs : 95/197 [00:01<00:01, 83.92it/s]
49%|##########################################################1 | Repairing epochs : 97/197 [00:01<00:01, 83.33it/s]
50%|###########################################################2 | Repairing epochs : 99/197 [00:01<00:01, 83.32it/s]
51%|###########################################################3 | Repairing epochs : 100/197 [00:01<00:01, 82.54it/s]
52%|############################################################5 | Repairing epochs : 102/197 [00:01<00:01, 82.66it/s]
53%|#############################################################7 | Repairing epochs : 104/197 [00:01<00:01, 83.40it/s]
54%|##############################################################9 | Repairing epochs : 106/197 [00:01<00:01, 82.72it/s]
55%|################################################################1 | Repairing epochs : 108/197 [00:01<00:01, 84.24it/s]
55%|################################################################7 | Repairing epochs : 109/197 [00:01<00:01, 83.42it/s]
56%|#################################################################3 | Repairing epochs : 110/197 [00:01<00:01, 82.61it/s]
57%|##################################################################5 | Repairing epochs : 112/197 [00:01<00:01, 81.96it/s]
58%|###################################################################7 | Repairing epochs : 114/197 [00:01<00:01, 81.15it/s]
59%|####################################################################8 | Repairing epochs : 116/197 [00:01<00:00, 81.35it/s]
60%|###################################################################### | Repairing epochs : 118/197 [00:01<00:00, 81.95it/s]
61%|#######################################################################2 | Repairing epochs : 120/197 [00:01<00:00, 81.54it/s]
62%|########################################################################4 | Repairing epochs : 122/197 [00:01<00:00, 81.16it/s]
63%|#########################################################################6 | Repairing epochs : 124/197 [00:01<00:00, 81.00it/s]
64%|##########################################################################8 | Repairing epochs : 126/197 [00:01<00:00, 82.40it/s]
65%|############################################################################6 | Repairing epochs : 129/197 [00:01<00:00, 83.46it/s]
66%|#############################################################################8 | Repairing epochs : 131/197 [00:01<00:00, 83.35it/s]
68%|##############################################################################9 | Repairing epochs : 133/197 [00:01<00:00, 82.70it/s]
69%|################################################################################1 | Repairing epochs : 135/197 [00:01<00:00, 82.72it/s]
70%|#################################################################################3 | Repairing epochs : 137/197 [00:01<00:00, 82.82it/s]
71%|##################################################################################5 | Repairing epochs : 139/197 [00:01<00:00, 82.75it/s]
71%|###################################################################################1 | Repairing epochs : 140/197 [00:01<00:00, 82.03it/s]
72%|####################################################################################3 | Repairing epochs : 142/197 [00:01<00:00, 82.07it/s]
73%|#####################################################################################5 | Repairing epochs : 144/197 [00:01<00:00, 81.59it/s]
74%|######################################################################################7 | Repairing epochs : 146/197 [00:01<00:00, 81.53it/s]
75%|#######################################################################################8 | Repairing epochs : 148/197 [00:01<00:00, 83.05it/s]
76%|######################################################################################### | Repairing epochs : 150/197 [00:01<00:00, 82.87it/s]
78%|##########################################################################################8 | Repairing epochs : 153/197 [00:01<00:00, 85.32it/s]
79%|############################################################################################ | Repairing epochs : 155/197 [00:01<00:00, 85.18it/s]
80%|#############################################################################################2 | Repairing epochs : 157/197 [00:01<00:00, 86.38it/s]
81%|##############################################################################################4 | Repairing epochs : 159/197 [00:01<00:00, 85.65it/s]
82%|###############################################################################################6 | Repairing epochs : 161/197 [00:01<00:00, 84.87it/s]
84%|#################################################################################################9 | Repairing epochs : 165/197 [00:01<00:00, 88.33it/s]
85%|###################################################################################################1 | Repairing epochs : 167/197 [00:02<00:00, 87.96it/s]
86%|####################################################################################################3 | Repairing epochs : 169/197 [00:02<00:00, 87.07it/s]
86%|####################################################################################################9 | Repairing epochs : 170/197 [00:02<00:00, 86.21it/s]
87%|######################################################################################################1 | Repairing epochs : 172/197 [00:02<00:00, 85.31it/s]
88%|#######################################################################################################3 | Repairing epochs : 174/197 [00:02<00:00, 85.20it/s]
89%|########################################################################################################5 | Repairing epochs : 176/197 [00:02<00:00, 84.34it/s]
90%|#########################################################################################################7 | Repairing epochs : 178/197 [00:02<00:00, 84.15it/s]
91%|##########################################################################################################9 | Repairing epochs : 180/197 [00:02<00:00, 83.65it/s]
92%|############################################################################################################ | Repairing epochs : 182/197 [00:02<00:00, 84.96it/s]
93%|#############################################################################################################2 | Repairing epochs : 184/197 [00:02<00:00, 84.95it/s]
94%|##############################################################################################################4 | Repairing epochs : 186/197 [00:02<00:00, 84.86it/s]
95%|###############################################################################################################6 | Repairing epochs : 188/197 [00:02<00:00, 84.32it/s]
96%|################################################################################################################8 | Repairing epochs : 190/197 [00:02<00:00, 85.43it/s]
97%|################################################################################################################## | Repairing epochs : 192/197 [00:02<00:00, 85.14it/s]
98%|###################################################################################################################2 | Repairing epochs : 194/197 [00:02<00:00, 85.18it/s]
99%|####################################################################################################################4| Repairing epochs : 196/197 [00:02<00:00, 85.13it/s]
100%|#####################################################################################################################| Repairing epochs : 197/197 [00:02<00:00, 84.34it/s]
100%|#####################################################################################################################| Repairing epochs : 197/197 [00:02<00:00, 82.96it/s]
No bad epochs were found for your data. Returning a copy of the data you wanted to clean. Interpolation may have been done.
We can visualize the cross validation curve over two variables
import numpy as np # noqa
import matplotlib.pyplot as plt # noqa
import matplotlib.patches as patches # noqa
from autoreject import set_matplotlib_defaults # noqa
set_matplotlib_defaults(plt, style='seaborn-white')
loss = ar.loss_['eeg'].mean(axis=-1) # losses are stored by channel type.
plt.matshow(loss.T * 1e6, cmap=plt.get_cmap('viridis'))
plt.xticks(range(len(ar.consensus)), ['%.1f' % c for c in ar.consensus])
plt.yticks(range(len(ar.n_interpolate)), ar.n_interpolate)
# Draw rectangle at location of best parameters
ax = plt.gca()
idx, jdx = np.unravel_index(loss.argmin(), loss.shape)
rect = patches.Rectangle((idx - 0.5, jdx - 0.5), 1, 1, linewidth=2,
edgecolor='r', facecolor='none')
ax.add_patch(rect)
ax.xaxis.set_ticks_position('bottom')
plt.xlabel(r'Consensus percentage $\kappa$')
plt.ylabel(r'Max sensors interpolated $\rho$')
plt.title('Mean cross validation error (x 1e6)')
plt.colorbar()
plt.show()
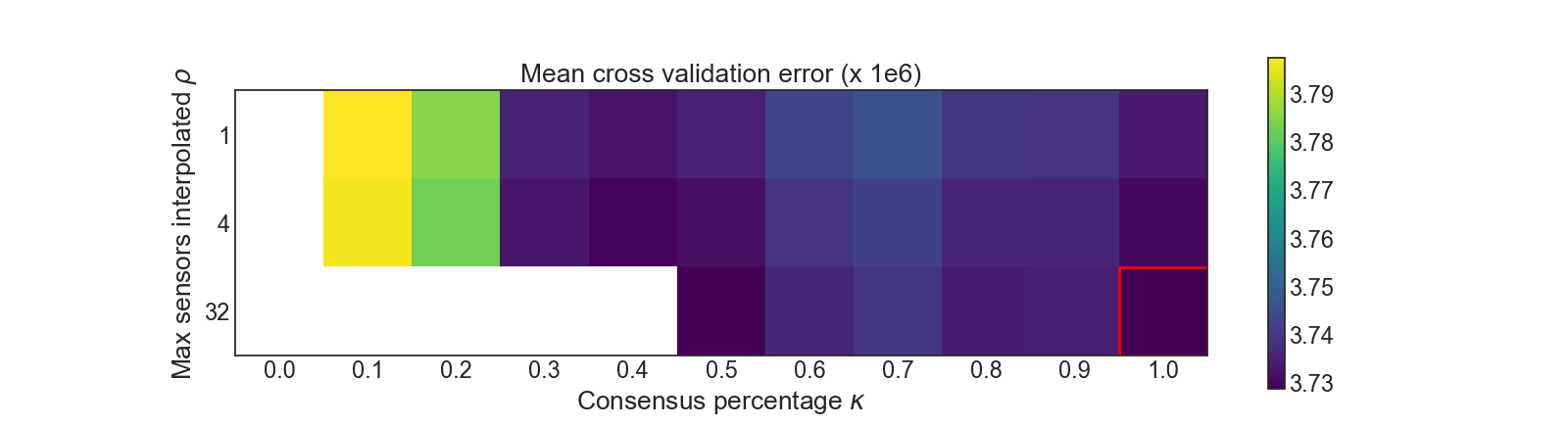
… and visualize the bad epochs and sensors. Bad sensors which have been interpolated are in blue. Bad sensors which are not interpolated are in red. Bad trials are also in red.
scalings = dict(eeg=40e-6)
reject_log.plot_epochs(this_epoch, scalings=scalings)
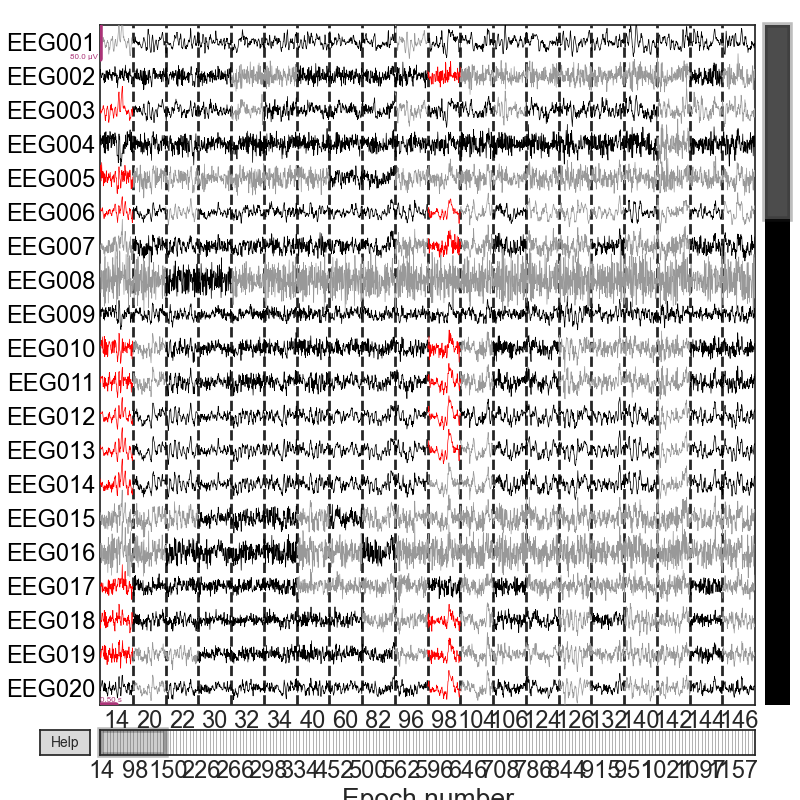
<MNEBrowseFigure size 800x800 with 4 Axes>
… and the epochs after cleaning with autoreject
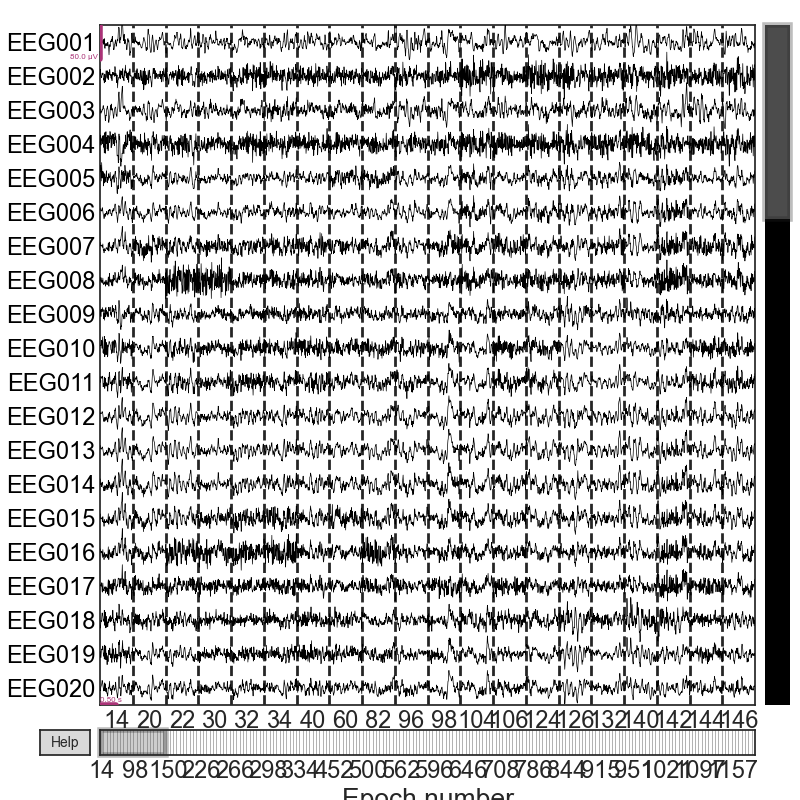
<MNEBrowseFigure size 800x800 with 4 Axes>
The epochs dropped by autoreject are also stored in epochs.drop_log
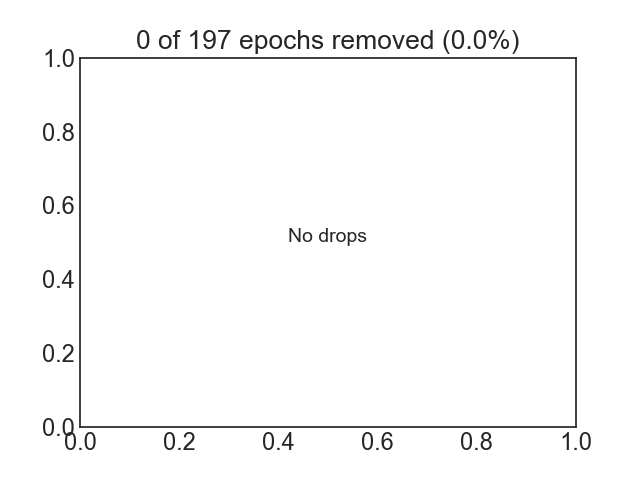
<Figure size 640x480 with 1 Axes>
Finally, the evoked before and after autoreject, for sanity check. We use
the spatial_colors
argument from MNE as it allows us to see that
the eyeblinks have not yet been cleaned but the bad channels have been
repaired.
ylim = dict(eeg=(-15, 15))
epochs.average().plot(ylim=ylim, spatial_colors=True)
epochs_ar.average().plot(ylim=ylim, spatial_colors=True)
NOTE: pick_channels() is a legacy function. New code should use inst.pick(...).
NOTE: pick_channels() is a legacy function. New code should use inst.pick(...).
<Figure size 640x300 with 2 Axes>
Total running time of the script: ( 1 minutes 58.656 seconds)