Note
Go to the end to download the full example code
Plot channel-level thresholds#
This example demonstrates how to use autoreject
to find
channel-wise thresholds.
# Author: Mainak Jas <mainak.jas@telecom-paristech.fr>
# License: BSD-3-Clause
Let us first load the raw data using mne.io.read_raw_fif()
.
import mne
from mne import io
from mne.datasets import sample
data_path = sample.data_path()
meg_path = data_path / 'MEG' / 'sample'
raw_fname = meg_path / 'sample_audvis_filt-0-40_raw.fif'
raw = io.read_raw_fif(raw_fname, preload=True)
Opening raw data file C:\Users\stefan\mne_data\MNE-sample-data\MEG\sample\sample_audvis_filt-0-40_raw.fif...
Read a total of 4 projection items:
PCA-v1 (1 x 102) idle
PCA-v2 (1 x 102) idle
PCA-v3 (1 x 102) idle
Average EEG reference (1 x 60) idle
Range : 6450 ... 48149 = 42.956 ... 320.665 secs
Ready.
Reading 0 ... 41699 = 0.000 ... 277.709 secs...
We can extract the events (or triggers) for epoching our signal.
event_fname = meg_path / 'sample_audvis_filt-0-40_raw-eve.fif'
event_id = {'Auditory/Left': 1}
tmin, tmax = -0.2, 0.5
events = mne.read_events(event_fname)
Now that we have the events, we can extract the trials for the selection
of channels defined by picks
.
epochs = mne.Epochs(raw, events, event_id, tmin, tmax,
baseline=(None, 0),
reject=None, verbose=False, preload=True)
picks = mne.pick_types(epochs.info, meg='grad', eeg=False, stim=False,
eog=False, exclude='bads')
Now, we compute the channel-level thresholds using
autoreject.compute_thresholds()
. The method parameter will determine
how we will search for thresholds over a range of potential candidates.
import numpy as np # noqa
from autoreject import compute_thresholds # noqa
# Get a dictionary of rejection thresholds
threshes = compute_thresholds(epochs, picks=picks, method='random_search',
random_state=42, augment=False,
verbose=True)
c:\users\stefan\desktop\git-repos\autoreject\autoreject\utils.py:66: UserWarning: 2 channels are marked as bad. These will be ignored. If you want them to be considered by autoreject please remove them from epochs.info["bads"].
warnings.warn(
0%| | Computing thresholds ... : 0/203 [00:00<?, ?it/s]
0%|5 | Computing thresholds ... : 1/203 [00:00<00:18, 10.81it/s]
1%|#1 | Computing thresholds ... : 2/203 [00:00<00:18, 11.00it/s]
1%|#6 | Computing thresholds ... : 3/203 [00:00<00:17, 11.30it/s]
2%|##2 | Computing thresholds ... : 4/203 [00:00<00:17, 11.11it/s]
2%|##7 | Computing thresholds ... : 5/203 [00:00<00:18, 11.00it/s]
3%|###3 | Computing thresholds ... : 6/203 [00:00<00:18, 10.92it/s]
3%|###8 | Computing thresholds ... : 7/203 [00:00<00:18, 10.87it/s]
4%|####4 | Computing thresholds ... : 8/203 [00:00<00:17, 10.84it/s]
4%|##### | Computing thresholds ... : 9/203 [00:00<00:17, 11.05it/s]
5%|#####5 | Computing thresholds ... : 10/203 [00:00<00:17, 10.99it/s]
5%|###### | Computing thresholds ... : 11/203 [00:01<00:17, 10.95it/s]
6%|######6 | Computing thresholds ... : 12/203 [00:01<00:17, 11.11it/s]
6%|#######1 | Computing thresholds ... : 13/203 [00:01<00:17, 11.06it/s]
7%|#######7 | Computing thresholds ... : 14/203 [00:01<00:17, 11.01it/s]
7%|########2 | Computing thresholds ... : 15/203 [00:01<00:16, 11.16it/s]
8%|########8 | Computing thresholds ... : 16/203 [00:01<00:16, 11.11it/s]
8%|#########3 | Computing thresholds ... : 17/203 [00:01<00:16, 11.07it/s]
9%|#########9 | Computing thresholds ... : 18/203 [00:01<00:16, 11.04it/s]
9%|##########4 | Computing thresholds ... : 19/203 [00:01<00:16, 11.09it/s]
10%|########### | Computing thresholds ... : 20/203 [00:01<00:16, 11.01it/s]
10%|###########5 | Computing thresholds ... : 21/203 [00:01<00:16, 11.00it/s]
11%|############1 | Computing thresholds ... : 22/203 [00:01<00:16, 10.99it/s]
11%|############6 | Computing thresholds ... : 23/203 [00:02<00:16, 10.99it/s]
12%|#############2 | Computing thresholds ... : 24/203 [00:02<00:16, 10.90it/s]
12%|#############7 | Computing thresholds ... : 25/203 [00:02<00:16, 10.85it/s]
13%|##############3 | Computing thresholds ... : 26/203 [00:02<00:16, 10.79it/s]
13%|##############8 | Computing thresholds ... : 27/203 [00:02<00:16, 10.76it/s]
14%|###############4 | Computing thresholds ... : 28/203 [00:02<00:16, 10.70it/s]
14%|################ | Computing thresholds ... : 29/203 [00:02<00:16, 10.64it/s]
15%|################5 | Computing thresholds ... : 30/203 [00:02<00:16, 10.72it/s]
15%|#################1 | Computing thresholds ... : 31/203 [00:02<00:16, 10.68it/s]
16%|#################6 | Computing thresholds ... : 32/203 [00:02<00:15, 10.77it/s]
16%|##################2 | Computing thresholds ... : 33/203 [00:03<00:15, 10.77it/s]
17%|##################7 | Computing thresholds ... : 34/203 [00:03<00:15, 10.80it/s]
17%|###################3 | Computing thresholds ... : 35/203 [00:03<00:15, 10.77it/s]
18%|###################8 | Computing thresholds ... : 36/203 [00:03<00:15, 10.89it/s]
18%|####################4 | Computing thresholds ... : 37/203 [00:03<00:15, 10.80it/s]
19%|####################9 | Computing thresholds ... : 38/203 [00:03<00:15, 10.82it/s]
19%|#####################5 | Computing thresholds ... : 39/203 [00:03<00:15, 10.83it/s]
20%|###################### | Computing thresholds ... : 40/203 [00:03<00:14, 10.90it/s]
20%|######################6 | Computing thresholds ... : 41/203 [00:03<00:14, 10.88it/s]
21%|#######################1 | Computing thresholds ... : 42/203 [00:03<00:14, 10.86it/s]
21%|#######################7 | Computing thresholds ... : 43/203 [00:03<00:14, 10.85it/s]
22%|########################2 | Computing thresholds ... : 44/203 [00:04<00:14, 10.86it/s]
22%|########################8 | Computing thresholds ... : 45/203 [00:04<00:14, 10.90it/s]
23%|#########################3 | Computing thresholds ... : 46/203 [00:04<00:14, 10.92it/s]
23%|#########################9 | Computing thresholds ... : 47/203 [00:04<00:14, 10.93it/s]
24%|##########################4 | Computing thresholds ... : 48/203 [00:04<00:14, 10.95it/s]
24%|########################### | Computing thresholds ... : 49/203 [00:04<00:14, 10.97it/s]
25%|###########################5 | Computing thresholds ... : 50/203 [00:04<00:13, 10.96it/s]
25%|############################1 | Computing thresholds ... : 51/203 [00:04<00:13, 10.97it/s]
26%|############################6 | Computing thresholds ... : 52/203 [00:04<00:13, 10.96it/s]
26%|#############################2 | Computing thresholds ... : 53/203 [00:04<00:13, 10.97it/s]
27%|#############################7 | Computing thresholds ... : 54/203 [00:04<00:13, 10.98it/s]
27%|##############################3 | Computing thresholds ... : 55/203 [00:05<00:13, 10.97it/s]
28%|##############################8 | Computing thresholds ... : 56/203 [00:05<00:13, 10.98it/s]
28%|###############################4 | Computing thresholds ... : 57/203 [00:05<00:13, 10.99it/s]
29%|################################ | Computing thresholds ... : 58/203 [00:05<00:13, 11.00it/s]
29%|################################5 | Computing thresholds ... : 59/203 [00:05<00:13, 11.01it/s]
30%|#################################1 | Computing thresholds ... : 60/203 [00:05<00:12, 11.01it/s]
30%|#################################6 | Computing thresholds ... : 61/203 [00:05<00:12, 11.02it/s]
31%|##################################2 | Computing thresholds ... : 62/203 [00:05<00:12, 11.10it/s]
31%|##################################7 | Computing thresholds ... : 63/203 [00:05<00:12, 11.07it/s]
32%|###################################3 | Computing thresholds ... : 64/203 [00:05<00:12, 11.05it/s]
32%|###################################8 | Computing thresholds ... : 65/203 [00:05<00:12, 11.12it/s]
33%|####################################4 | Computing thresholds ... : 66/203 [00:06<00:12, 11.10it/s]
33%|####################################9 | Computing thresholds ... : 67/203 [00:06<00:12, 11.09it/s]
33%|#####################################5 | Computing thresholds ... : 68/203 [00:06<00:12, 11.06it/s]
34%|###################################### | Computing thresholds ... : 69/203 [00:06<00:12, 11.14it/s]
34%|######################################6 | Computing thresholds ... : 70/203 [00:06<00:11, 11.11it/s]
35%|#######################################1 | Computing thresholds ... : 71/203 [00:06<00:11, 11.18it/s]
35%|#######################################7 | Computing thresholds ... : 72/203 [00:06<00:11, 11.09it/s]
36%|########################################2 | Computing thresholds ... : 73/203 [00:06<00:11, 11.11it/s]
36%|########################################8 | Computing thresholds ... : 74/203 [00:06<00:11, 11.18it/s]
37%|#########################################3 | Computing thresholds ... : 75/203 [00:06<00:11, 11.15it/s]
37%|#########################################9 | Computing thresholds ... : 76/203 [00:06<00:11, 11.12it/s]
38%|##########################################4 | Computing thresholds ... : 77/203 [00:07<00:11, 10.99it/s]
38%|########################################### | Computing thresholds ... : 78/203 [00:07<00:11, 11.00it/s]
39%|###########################################5 | Computing thresholds ... : 79/203 [00:07<00:11, 11.06it/s]
39%|############################################1 | Computing thresholds ... : 80/203 [00:07<00:11, 11.04it/s]
40%|############################################6 | Computing thresholds ... : 81/203 [00:07<00:10, 11.11it/s]
40%|#############################################2 | Computing thresholds ... : 82/203 [00:07<00:10, 11.08it/s]
41%|#############################################7 | Computing thresholds ... : 83/203 [00:07<00:10, 11.06it/s]
41%|##############################################3 | Computing thresholds ... : 84/203 [00:07<00:10, 11.06it/s]
42%|##############################################8 | Computing thresholds ... : 85/203 [00:07<00:10, 11.12it/s]
42%|###############################################4 | Computing thresholds ... : 86/203 [00:07<00:10, 11.10it/s]
43%|################################################ | Computing thresholds ... : 87/203 [00:07<00:10, 11.07it/s]
43%|################################################5 | Computing thresholds ... : 88/203 [00:08<00:10, 11.05it/s]
44%|#################################################1 | Computing thresholds ... : 89/203 [00:08<00:10, 11.13it/s]
44%|#################################################6 | Computing thresholds ... : 90/203 [00:08<00:10, 11.05it/s]
45%|##################################################2 | Computing thresholds ... : 91/203 [00:08<00:10, 11.08it/s]
45%|##################################################7 | Computing thresholds ... : 92/203 [00:08<00:09, 11.14it/s]
46%|###################################################3 | Computing thresholds ... : 93/203 [00:08<00:09, 11.07it/s]
46%|###################################################8 | Computing thresholds ... : 94/203 [00:08<00:09, 11.10it/s]
47%|####################################################4 | Computing thresholds ... : 95/203 [00:08<00:09, 11.17it/s]
47%|####################################################9 | Computing thresholds ... : 96/203 [00:08<00:09, 11.10it/s]
48%|#####################################################5 | Computing thresholds ... : 97/203 [00:08<00:09, 11.11it/s]
48%|###################################################### | Computing thresholds ... : 98/203 [00:08<00:09, 11.13it/s]
49%|######################################################6 | Computing thresholds ... : 99/203 [00:08<00:09, 11.17it/s]
49%|######################################################6 | Computing thresholds ... : 100/203 [00:09<00:09, 11.10it/s]
50%|#######################################################2 | Computing thresholds ... : 101/203 [00:09<00:09, 11.10it/s]
50%|#######################################################7 | Computing thresholds ... : 102/203 [00:09<00:09, 11.10it/s]
51%|########################################################3 | Computing thresholds ... : 103/203 [00:09<00:08, 11.16it/s]
51%|########################################################8 | Computing thresholds ... : 104/203 [00:09<00:08, 11.13it/s]
52%|#########################################################4 | Computing thresholds ... : 105/203 [00:09<00:08, 11.20it/s]
52%|#########################################################9 | Computing thresholds ... : 106/203 [00:09<00:08, 11.17it/s]
53%|##########################################################5 | Computing thresholds ... : 107/203 [00:09<00:08, 11.14it/s]
53%|########################################################### | Computing thresholds ... : 108/203 [00:09<00:08, 11.13it/s]
54%|###########################################################6 | Computing thresholds ... : 109/203 [00:09<00:08, 11.18it/s]
54%|############################################################1 | Computing thresholds ... : 110/203 [00:09<00:08, 11.16it/s]
55%|############################################################6 | Computing thresholds ... : 111/203 [00:10<00:08, 11.13it/s]
55%|#############################################################2 | Computing thresholds ... : 112/203 [00:10<00:08, 11.10it/s]
56%|#############################################################7 | Computing thresholds ... : 113/203 [00:10<00:08, 11.18it/s]
56%|##############################################################3 | Computing thresholds ... : 114/203 [00:10<00:07, 11.15it/s]
57%|##############################################################8 | Computing thresholds ... : 115/203 [00:10<00:07, 11.13it/s]
57%|###############################################################4 | Computing thresholds ... : 116/203 [00:10<00:07, 11.13it/s]
58%|###############################################################9 | Computing thresholds ... : 117/203 [00:10<00:07, 11.13it/s]
58%|################################################################5 | Computing thresholds ... : 118/203 [00:10<00:07, 11.20it/s]
59%|################################################################# | Computing thresholds ... : 119/203 [00:10<00:07, 11.14it/s]
59%|#################################################################6 | Computing thresholds ... : 120/203 [00:10<00:07, 11.15it/s]
60%|##################################################################1 | Computing thresholds ... : 121/203 [00:10<00:07, 11.15it/s]
60%|##################################################################7 | Computing thresholds ... : 122/203 [00:11<00:07, 11.14it/s]
61%|###################################################################2 | Computing thresholds ... : 123/203 [00:11<00:07, 11.13it/s]
61%|###################################################################8 | Computing thresholds ... : 124/203 [00:11<00:07, 11.13it/s]
62%|####################################################################3 | Computing thresholds ... : 125/203 [00:11<00:07, 11.11it/s]
62%|####################################################################8 | Computing thresholds ... : 126/203 [00:11<00:06, 11.11it/s]
63%|#####################################################################4 | Computing thresholds ... : 127/203 [00:11<00:06, 11.10it/s]
63%|#####################################################################9 | Computing thresholds ... : 128/203 [00:11<00:06, 11.10it/s]
64%|######################################################################5 | Computing thresholds ... : 129/203 [00:11<00:06, 11.10it/s]
64%|####################################################################### | Computing thresholds ... : 130/203 [00:11<00:06, 11.10it/s]
65%|#######################################################################6 | Computing thresholds ... : 131/203 [00:11<00:06, 11.10it/s]
65%|########################################################################1 | Computing thresholds ... : 132/203 [00:11<00:06, 11.08it/s]
66%|########################################################################7 | Computing thresholds ... : 133/203 [00:12<00:06, 11.05it/s]
66%|#########################################################################2 | Computing thresholds ... : 134/203 [00:12<00:06, 11.04it/s]
67%|#########################################################################8 | Computing thresholds ... : 135/203 [00:12<00:06, 11.04it/s]
67%|##########################################################################3 | Computing thresholds ... : 136/203 [00:12<00:06, 11.03it/s]
67%|##########################################################################9 | Computing thresholds ... : 137/203 [00:12<00:06, 11.00it/s]
68%|###########################################################################4 | Computing thresholds ... : 138/203 [00:12<00:05, 10.97it/s]
68%|############################################################################ | Computing thresholds ... : 139/203 [00:12<00:05, 10.98it/s]
69%|############################################################################5 | Computing thresholds ... : 140/203 [00:12<00:05, 10.97it/s]
69%|############################################################################# | Computing thresholds ... : 141/203 [00:12<00:05, 11.05it/s]
70%|#############################################################################6 | Computing thresholds ... : 142/203 [00:12<00:05, 11.03it/s]
70%|##############################################################################1 | Computing thresholds ... : 143/203 [00:12<00:05, 11.01it/s]
71%|##############################################################################7 | Computing thresholds ... : 144/203 [00:13<00:05, 11.08it/s]
71%|###############################################################################2 | Computing thresholds ... : 145/203 [00:13<00:05, 11.06it/s]
72%|###############################################################################8 | Computing thresholds ... : 146/203 [00:13<00:05, 11.04it/s]
72%|################################################################################3 | Computing thresholds ... : 147/203 [00:13<00:05, 11.11it/s]
73%|################################################################################9 | Computing thresholds ... : 148/203 [00:13<00:04, 11.09it/s]
73%|#################################################################################4 | Computing thresholds ... : 149/203 [00:13<00:04, 11.06it/s]
74%|################################################################################## | Computing thresholds ... : 150/203 [00:13<00:04, 11.04it/s]
74%|##################################################################################5 | Computing thresholds ... : 151/203 [00:13<00:04, 11.02it/s]
75%|###################################################################################1 | Computing thresholds ... : 152/203 [00:13<00:04, 11.09it/s]
75%|###################################################################################6 | Computing thresholds ... : 153/203 [00:13<00:04, 11.07it/s]
76%|####################################################################################2 | Computing thresholds ... : 154/203 [00:13<00:04, 11.02it/s]
76%|####################################################################################7 | Computing thresholds ... : 155/203 [00:14<00:04, 11.03it/s]
77%|#####################################################################################3 | Computing thresholds ... : 156/203 [00:14<00:04, 11.10it/s]
77%|#####################################################################################8 | Computing thresholds ... : 157/203 [00:14<00:04, 11.08it/s]
78%|######################################################################################3 | Computing thresholds ... : 158/203 [00:14<00:04, 11.05it/s]
78%|######################################################################################9 | Computing thresholds ... : 159/203 [00:14<00:03, 11.03it/s]
79%|#######################################################################################4 | Computing thresholds ... : 160/203 [00:14<00:03, 11.01it/s]
79%|######################################################################################## | Computing thresholds ... : 161/203 [00:14<00:03, 10.99it/s]
80%|########################################################################################5 | Computing thresholds ... : 162/203 [00:14<00:03, 11.07it/s]
80%|#########################################################################################1 | Computing thresholds ... : 163/203 [00:14<00:03, 11.04it/s]
81%|#########################################################################################6 | Computing thresholds ... : 164/203 [00:14<00:03, 11.02it/s]
81%|##########################################################################################2 | Computing thresholds ... : 165/203 [00:14<00:03, 10.98it/s]
82%|##########################################################################################7 | Computing thresholds ... : 166/203 [00:15<00:03, 11.08it/s]
82%|###########################################################################################3 | Computing thresholds ... : 167/203 [00:15<00:03, 11.06it/s]
83%|###########################################################################################8 | Computing thresholds ... : 168/203 [00:15<00:03, 11.04it/s]
83%|############################################################################################4 | Computing thresholds ... : 169/203 [00:15<00:03, 11.11it/s]
84%|############################################################################################9 | Computing thresholds ... : 170/203 [00:15<00:02, 11.08it/s]
84%|#############################################################################################5 | Computing thresholds ... : 171/203 [00:15<00:02, 10.96it/s]
85%|############################################################################################## | Computing thresholds ... : 172/203 [00:15<00:02, 11.04it/s]
85%|##############################################################################################5 | Computing thresholds ... : 173/203 [00:15<00:02, 11.02it/s]
86%|###############################################################################################1 | Computing thresholds ... : 174/203 [00:15<00:02, 11.00it/s]
86%|###############################################################################################6 | Computing thresholds ... : 175/203 [00:15<00:02, 11.01it/s]
87%|################################################################################################2 | Computing thresholds ... : 176/203 [00:15<00:02, 11.06it/s]
87%|################################################################################################7 | Computing thresholds ... : 177/203 [00:16<00:02, 11.03it/s]
88%|#################################################################################################3 | Computing thresholds ... : 178/203 [00:16<00:02, 11.11it/s]
88%|#################################################################################################8 | Computing thresholds ... : 179/203 [00:16<00:02, 11.08it/s]
89%|##################################################################################################4 | Computing thresholds ... : 180/203 [00:16<00:02, 11.06it/s]
89%|##################################################################################################9 | Computing thresholds ... : 181/203 [00:16<00:01, 11.13it/s]
90%|###################################################################################################5 | Computing thresholds ... : 182/203 [00:16<00:01, 11.10it/s]
90%|#################################################################################################### | Computing thresholds ... : 183/203 [00:16<00:01, 11.08it/s]
91%|####################################################################################################6 | Computing thresholds ... : 184/203 [00:16<00:01, 11.05it/s]
91%|#####################################################################################################1 | Computing thresholds ... : 185/203 [00:16<00:01, 11.13it/s]
92%|#####################################################################################################7 | Computing thresholds ... : 186/203 [00:16<00:01, 11.10it/s]
92%|######################################################################################################2 | Computing thresholds ... : 187/203 [00:16<00:01, 11.08it/s]
93%|######################################################################################################7 | Computing thresholds ... : 188/203 [00:17<00:01, 11.15it/s]
93%|#######################################################################################################3 | Computing thresholds ... : 189/203 [00:17<00:01, 11.12it/s]
94%|#######################################################################################################8 | Computing thresholds ... : 190/203 [00:17<00:01, 11.10it/s]
94%|########################################################################################################4 | Computing thresholds ... : 191/203 [00:17<00:01, 11.17it/s]
95%|########################################################################################################9 | Computing thresholds ... : 192/203 [00:17<00:00, 11.14it/s]
95%|#########################################################################################################5 | Computing thresholds ... : 193/203 [00:17<00:00, 11.11it/s]
96%|########################################################################################################## | Computing thresholds ... : 194/203 [00:17<00:00, 11.18it/s]
96%|##########################################################################################################6 | Computing thresholds ... : 195/203 [00:17<00:00, 10.99it/s]
97%|###########################################################################################################1 | Computing thresholds ... : 196/203 [00:17<00:00, 10.93it/s]
97%|###########################################################################################################7 | Computing thresholds ... : 197/203 [00:17<00:00, 11.02it/s]
98%|############################################################################################################2 | Computing thresholds ... : 198/203 [00:17<00:00, 11.00it/s]
98%|############################################################################################################8 | Computing thresholds ... : 199/203 [00:18<00:00, 10.98it/s]
99%|#############################################################################################################3 | Computing thresholds ... : 200/203 [00:18<00:00, 10.96it/s]
99%|#############################################################################################################9 | Computing thresholds ... : 201/203 [00:18<00:00, 11.04it/s]
100%|##############################################################################################################4| Computing thresholds ... : 202/203 [00:18<00:00, 11.02it/s]
100%|###############################################################################################################| Computing thresholds ... : 203/203 [00:18<00:00, 11.09it/s]
100%|###############################################################################################################| Computing thresholds ... : 203/203 [00:18<00:00, 11.05it/s]
Finally, let us plot a histogram of the channel-level thresholds to verify that the thresholds are indeed different for different sensors.
import matplotlib.pyplot as plt # noqa
from autoreject import set_matplotlib_defaults # noqa
set_matplotlib_defaults(plt)
unit = r'fT/cm'
scaling = 1e13
plt.figure(figsize=(6, 5))
plt.tick_params(axis='x', which='both', bottom='off', top='off')
plt.tick_params(axis='y', which='both', left='off', right='off')
plt.hist(scaling * np.array(list(threshes.values())), 30,
color='g', alpha=0.4)
plt.xlabel('Threshold (%s)' % unit)
plt.ylabel('Number of sensors')
plt.xlim((100, 950))
plt.tight_layout()
plt.show()
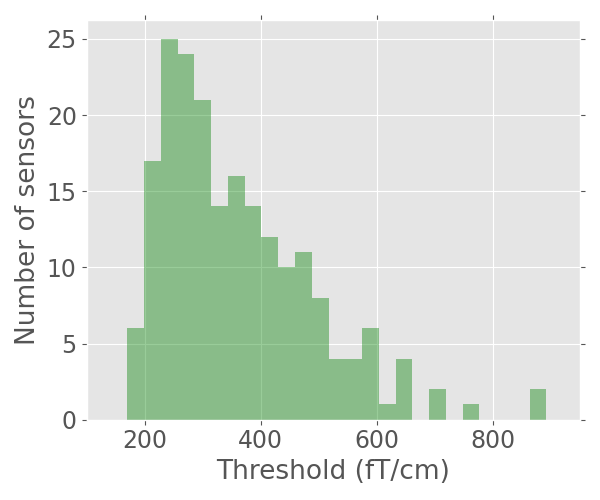
Total running time of the script: ( 0 minutes 18.849 seconds)