Note
Go to the end to download the full example code
Preprocessing workflow with autoreject
and ICA#
This example demonstrates how to visualize data when preprocessing
with autoreject
and discusses decisions about when and which
other preprocessing steps to use in combination.
tldr: We recommend that you first highpass filter the data, then run autoreject (local) and supply the bad epochs detected by it to the ICA algorithm for a robust fit, and finally run autoreject (local) again.
# Author: Alex Rockhill <aprockhill@mailbox.org>
# Mainak Jas <mjas@mgh.harvard.edu>
# Apoorva Karekal <apoorvak@uoregon.edu>
#
# License: BSD-3-Clause
# sphinx_gallery_thumbnail_number = 9
Table of Contents
First, we download resting-state EEG data from a Parkinson’s patient
from OpenNeuro. We will do this using openneuro-py
which can be
installed with the command pip install openneuro-py
.
import os
import matplotlib.pyplot as plt
import openneuro
import mne
import autoreject
dataset = 'ds002778' # The id code on OpenNeuro for this example dataset
subject_id = 'pd14'
target_dir = os.path.join(
os.path.dirname(autoreject.__file__), '..', 'examples', dataset)
os.makedirs(target_dir, exist_ok=True)
openneuro.download(dataset=dataset, target_dir=target_dir,
include=[f'sub-{subject_id}/ses-off'])
Hello! This is openneuro-py 2022.4.0. Great to see you!
Please report problems and bugs at
https://github.com/hoechenberger/openneuro-py/issues
Preparing to download ds002778 ...
Traversing directories for ds002778: 0 entities [00:00, ? entities/s]
Traversing directories for ds002778: 7 entities [00:00, 14.58 entities/s]
Traversing directories for ds002778: 9 entities [00:01, 5.22 entities/s]
Traversing directories for ds002778: 10 entities [00:01, 4.14 entities/s]
Traversing directories for ds002778: 11 entities [00:02, 3.46 entities/s]
Traversing directories for ds002778: 12 entities [00:02, 2.97 entities/s]
Traversing directories for ds002778: 13 entities [00:03, 2.65 entities/s]
Traversing directories for ds002778: 14 entities [00:03, 2.48 entities/s]
Traversing directories for ds002778: 15 entities [00:04, 2.37 entities/s]
Traversing directories for ds002778: 16 entities [00:04, 2.31 entities/s]
Traversing directories for ds002778: 17 entities [00:05, 2.26 entities/s]
Traversing directories for ds002778: 18 entities [00:05, 2.23 entities/s]
Traversing directories for ds002778: 19 entities [00:06, 2.20 entities/s]
Traversing directories for ds002778: 20 entities [00:06, 2.18 entities/s]
Traversing directories for ds002778: 21 entities [00:07, 2.17 entities/s]
Traversing directories for ds002778: 22 entities [00:07, 2.16 entities/s]
Traversing directories for ds002778: 23 entities [00:08, 2.14 entities/s]
Traversing directories for ds002778: 24 entities [00:08, 2.52 entities/s]
Traversing directories for ds002778: 25 entities [00:08, 2.37 entities/s]
Traversing directories for ds002778: 26 entities [00:09, 2.69 entities/s]
Traversing directories for ds002778: 27 entities [00:09, 2.51 entities/s]
Traversing directories for ds002778: 28 entities [00:09, 2.86 entities/s]
Traversing directories for ds002778: 29 entities [00:10, 2.60 entities/s]
Traversing directories for ds002778: 30 entities [00:10, 2.65 entities/s]
Traversing directories for ds002778: 32 entities [00:10, 3.84 entities/s]
Traversing directories for ds002778: 36 entities [00:11, 6.70 entities/s]
Traversing directories for ds002778: 37 entities [00:11, 5.38 entities/s]
Traversing directories for ds002778: 39 entities [00:11, 6.11 entities/s]
Traversing directories for ds002778: 43 entities [00:12, 7.08 entities/s]
Traversing directories for ds002778: 44 entities [00:12, 6.46 entities/s]
Traversing directories for ds002778: 45 entities [00:12, 4.78 entities/s]
Traversing directories for ds002778: 46 entities [00:13, 4.67 entities/s]
Traversing directories for ds002778: 47 entities [00:13, 3.64 entities/s]
Traversing directories for ds002778: 48 entities [00:13, 3.78 entities/s]
Traversing directories for ds002778: 49 entities [00:14, 3.16 entities/s]
Traversing directories for ds002778: 50 entities [00:14, 3.36 entities/s]
Traversing directories for ds002778: 51 entities [00:15, 2.91 entities/s]
Traversing directories for ds002778: 52 entities [00:15, 3.22 entities/s]
Traversing directories for ds002778: 53 entities [00:15, 2.77 entities/s]
Traversing directories for ds002778: 54 entities [00:15, 3.09 entities/s]
Traversing directories for ds002778: 55 entities [00:16, 2.73 entities/s]
Traversing directories for ds002778: 56 entities [00:16, 3.03 entities/s]
Traversing directories for ds002778: 57 entities [00:17, 2.71 entities/s]
Traversing directories for ds002778: 58 entities [00:17, 3.04 entities/s]
Traversing directories for ds002778: 59 entities [00:17, 2.62 entities/s]
Traversing directories for ds002778: 60 entities [00:18, 2.98 entities/s]
Traversing directories for ds002778: 61 entities [00:18, 2.58 entities/s]
Traversing directories for ds002778: 62 entities [00:18, 2.86 entities/s]
Traversing directories for ds002778: 63 entities [00:19, 2.58 entities/s]
Traversing directories for ds002778: 64 entities [00:19, 2.90 entities/s]
Traversing directories for ds002778: 64 entities [00:19, 3.26 entities/s]
Retrieving up to 12 files (5 concurrent downloads).
Skipping participants.tsv: already downloaded.: 100%|###############################################################################################| 1.62k/1.62k [00:00<?, ?B/s]
Skipping participants.json: already downloaded.: 100%|##############################################################################################| 1.24k/1.24k [00:00<?, ?B/s]
Skipping sub-pd14_ses-off_scans.tsv: already downloaded.: 100%|#######################################################################################| 76.0/76.0 [00:00<?, ?B/s]
Skipping sub-pd14_ses-off_task-rest_beh.tsv: already downloaded.: 100%|###############################################################################| 10.0/10.0 [00:00<?, ?B/s]
Re-downloading README: file size mismatch.: 0.00B [00:00, ?B/s]
Skipping sub-pd14_ses-off_task-rest_channels.tsv: already downloaded.: 100%|########################################################################| 2.22k/2.22k [00:00<?, ?B/s]
Skipping sub-pd14_ses-off_task-rest_eeg.json: already downloaded.: 100%|################################################################################| 471/471 [00:00<?, ?B/s]
Skipping sub-pd14_ses-off_task-rest_eeg.bdf: already downloaded.: 100%|#############################################################################| 17.5M/17.5M [00:00<?, ?B/s]
Re-downloading dataset_description.json: file size mismatch.: 0.00B [00:00, ?B/s]
Re-downloading CHANGES: file size mismatch.: 0.00B [00:00, ?B/s]
Skipping sub-pd14_ses-off_task-rest_events.tsv: already downloaded.: 100%|############################################################################| 65.0/65.0 [00:00<?, ?B/s]
Re-downloading sub-pd14_ses-off_task-rest_beh.json: file size mismatch.: 0.00B [00:00, ?B/s]
Finished downloading ds002778.
Please enjoy your brains.
We will now load in the raw data from the bdf file downloaded from OpenNeuro and, since this is resting-state data without any events, make regularly spaced events with which to epoch the raw data. In the averaged plot, we can see that there may be some eyeblink artifact contamination but, overall, the data is typical of resting-state EEG.
raw_fname = os.path.join(target_dir, f'sub-{subject_id}', 'ses-off', 'eeg',
'sub-pd14_ses-off_task-rest_eeg.bdf')
raw = mne.io.read_raw_bdf(raw_fname, preload=True)
dig_montage = mne.channels.make_standard_montage('biosemi32')
raw.drop_channels([ch for ch in raw.ch_names
if ch not in dig_montage.ch_names])
raw.set_montage(dig_montage) # use the standard montage
epochs = mne.make_fixed_length_epochs(raw, duration=3, preload=True)
# plot the data
epochs.average().detrend().plot_joint()
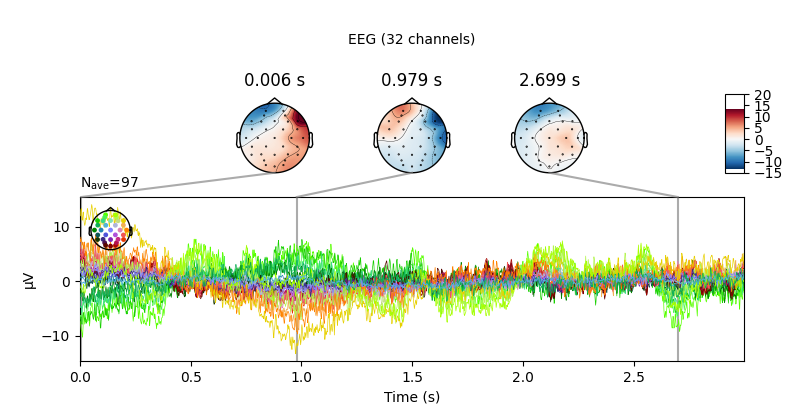
Extracting EDF parameters from c:\users\stefan\desktop\git-repos\autoreject\examples\ds002778\sub-pd14\ses-off\eeg\sub-pd14_ses-off_task-rest_eeg.bdf...
BDF file detected
Setting channel info structure...
Creating raw.info structure...
Reading 0 ... 149503 = 0.000 ... 291.998 secs...
Not setting metadata
97 matching events found
No baseline correction applied
0 projection items activated
Using data from preloaded Raw for 97 events and 1536 original time points ...
0 bad epochs dropped
NOTE: pick_channels() is a legacy function. New code should use inst.pick(...).
No projector specified for this dataset. Please consider the method self.add_proj.
NOTE: pick_channels() is a legacy function. New code should use inst.pick(...).
<Figure size 800x420 with 7 Axes>
Autoreject without any other preprocessing#
Now, we’ll naively apply autoreject as our first preprocessing step.
As we can see in the plot of the rejected epochs, there are many eyeblinks that caused the epoch to be dropped. This resulted in a lot of the data being lost.
The data looks fairly clean already and we don’t want to interpolate more than a few sensors since we only have 32 to start, so the number of channels to interpolate was set to check some low numbers
ar = autoreject.AutoReject(n_interpolate=[1, 2, 3, 4], random_state=11,
n_jobs=1, verbose=True)
ar.fit(epochs[:20]) # fit on a few epochs to save time
epochs_ar, reject_log = ar.transform(epochs, return_log=True)
Running autoreject on ch_type=eeg
0%| | Creating augmented epochs : 0/32 [00:00<?, ?it/s]
3%|###4 | Creating augmented epochs : 1/32 [00:00<00:00, 60.96it/s]
9%|##########4 | Creating augmented epochs : 3/32 [00:00<00:00, 75.36it/s]
12%|#############8 | Creating augmented epochs : 4/32 [00:00<00:00, 70.88it/s]
19%|####################8 | Creating augmented epochs : 6/32 [00:00<00:00, 75.01it/s]
25%|###########################7 | Creating augmented epochs : 8/32 [00:00<00:00, 77.47it/s]
31%|##################################3 | Creating augmented epochs : 10/32 [00:00<00:00, 78.57it/s]
38%|#########################################2 | Creating augmented epochs : 12/32 [00:00<00:00, 79.20it/s]
44%|################################################1 | Creating augmented epochs : 14/32 [00:00<00:00, 77.98it/s]
50%|####################################################### | Creating augmented epochs : 16/32 [00:00<00:00, 78.50it/s]
56%|#############################################################8 | Creating augmented epochs : 18/32 [00:00<00:00, 78.88it/s]
62%|####################################################################7 | Creating augmented epochs : 20/32 [00:00<00:00, 77.84it/s]
69%|###########################################################################6 | Creating augmented epochs : 22/32 [00:00<00:00, 78.42it/s]
75%|##################################################################################5 | Creating augmented epochs : 24/32 [00:00<00:00, 78.56it/s]
81%|#########################################################################################3 | Creating augmented epochs : 26/32 [00:00<00:00, 79.29it/s]
88%|################################################################################################2 | Creating augmented epochs : 28/32 [00:00<00:00, 79.80it/s]
94%|#######################################################################################################1 | Creating augmented epochs : 30/32 [00:00<00:00, 80.11it/s]
97%|##########################################################################################################5 | Creating augmented epochs : 31/32 [00:00<00:00, 79.05it/s]
100%|##############################################################################################################| Creating augmented epochs : 32/32 [00:00<00:00, 78.97it/s]
0%| | Computing thresholds ... : 0/32 [00:00<?, ?it/s]
3%|###5 | Computing thresholds ... : 1/32 [00:00<00:11, 2.63it/s]
6%|####### | Computing thresholds ... : 2/32 [00:00<00:10, 2.84it/s]
9%|##########5 | Computing thresholds ... : 3/32 [00:01<00:09, 2.96it/s]
12%|############## | Computing thresholds ... : 4/32 [00:01<00:09, 2.98it/s]
16%|#################5 | Computing thresholds ... : 5/32 [00:01<00:09, 3.00it/s]
19%|##################### | Computing thresholds ... : 6/32 [00:01<00:08, 3.01it/s]
22%|########################5 | Computing thresholds ... : 7/32 [00:02<00:08, 3.00it/s]
25%|############################ | Computing thresholds ... : 8/32 [00:02<00:07, 3.01it/s]
28%|###############################5 | Computing thresholds ... : 9/32 [00:02<00:07, 3.03it/s]
31%|##################################6 | Computing thresholds ... : 10/32 [00:03<00:07, 3.05it/s]
34%|######################################1 | Computing thresholds ... : 11/32 [00:03<00:06, 3.03it/s]
38%|#########################################6 | Computing thresholds ... : 12/32 [00:03<00:06, 3.04it/s]
41%|############################################# | Computing thresholds ... : 13/32 [00:04<00:06, 3.06it/s]
44%|################################################5 | Computing thresholds ... : 14/32 [00:04<00:05, 3.05it/s]
47%|#################################################### | Computing thresholds ... : 15/32 [00:04<00:05, 3.06it/s]
50%|#######################################################5 | Computing thresholds ... : 16/32 [00:05<00:05, 3.06it/s]
53%|##########################################################9 | Computing thresholds ... : 17/32 [00:05<00:04, 3.04it/s]
56%|##############################################################4 | Computing thresholds ... : 18/32 [00:05<00:04, 3.05it/s]
59%|#################################################################9 | Computing thresholds ... : 19/32 [00:06<00:04, 3.06it/s]
62%|#####################################################################3 | Computing thresholds ... : 20/32 [00:06<00:03, 3.05it/s]
66%|########################################################################8 | Computing thresholds ... : 21/32 [00:06<00:03, 3.05it/s]
69%|############################################################################3 | Computing thresholds ... : 22/32 [00:07<00:03, 3.06it/s]
72%|###############################################################################7 | Computing thresholds ... : 23/32 [00:07<00:02, 3.07it/s]
75%|###################################################################################2 | Computing thresholds ... : 24/32 [00:07<00:02, 3.07it/s]
78%|######################################################################################7 | Computing thresholds ... : 25/32 [00:08<00:02, 3.08it/s]
81%|##########################################################################################1 | Computing thresholds ... : 26/32 [00:08<00:01, 3.07it/s]
84%|#############################################################################################6 | Computing thresholds ... : 27/32 [00:08<00:01, 3.06it/s]
88%|#################################################################################################1 | Computing thresholds ... : 28/32 [00:09<00:01, 3.07it/s]
91%|####################################################################################################5 | Computing thresholds ... : 29/32 [00:09<00:00, 3.07it/s]
94%|######################################################################################################## | Computing thresholds ... : 30/32 [00:09<00:00, 3.07it/s]
97%|###########################################################################################################5 | Computing thresholds ... : 31/32 [00:10<00:00, 3.08it/s]
100%|###############################################################################################################| Computing thresholds ... : 32/32 [00:10<00:00, 3.09it/s]
100%|###############################################################################################################| Computing thresholds ... : 32/32 [00:10<00:00, 3.07it/s]
0%| | Repairing epochs : 0/20 [00:00<?, ?it/s]
35%|########################################## | Repairing epochs : 7/20 [00:00<00:00, 411.11it/s]
75%|#########################################################################################2 | Repairing epochs : 15/20 [00:00<00:00, 433.41it/s]
100%|#######################################################################################################################| Repairing epochs : 20/20 [00:00<00:00, 433.18it/s]
0%| | n_interp : 0/4 [00:00<?, ?it/s]
0%| | Repairing epochs : 0/20 [00:00<?, ?it/s]
15%|################## | Repairing epochs : 3/20 [00:00<00:00, 176.05it/s]
40%|################################################ | Repairing epochs : 8/20 [00:00<00:00, 229.44it/s]
60%|#######################################################################3 | Repairing epochs : 12/20 [00:00<00:00, 233.74it/s]
80%|###############################################################################################2 | Repairing epochs : 16/20 [00:00<00:00, 210.86it/s]
95%|################################################################################################################# | Repairing epochs : 19/20 [00:00<00:00, 202.57it/s]
100%|#######################################################################################################################| Repairing epochs : 20/20 [00:00<00:00, 203.75it/s]
0%| | Fold : 0/10 [00:00<?, ?it/s]
10%|#############2 | Fold : 1/10 [00:00<00:01, 8.47it/s]
20%|##########################4 | Fold : 2/10 [00:00<00:00, 8.45it/s]
30%|#######################################6 | Fold : 3/10 [00:00<00:00, 8.55it/s]
40%|####################################################8 | Fold : 4/10 [00:00<00:00, 8.64it/s]
50%|################################################################## | Fold : 5/10 [00:00<00:00, 8.65it/s]
60%|###############################################################################2 | Fold : 6/10 [00:00<00:00, 8.70it/s]
70%|############################################################################################3 | Fold : 7/10 [00:00<00:00, 8.74it/s]
80%|#########################################################################################################6 | Fold : 8/10 [00:00<00:00, 8.76it/s]
90%|######################################################################################################################8 | Fold : 9/10 [00:01<00:00, 8.72it/s]
100%|###################################################################################################################################| Fold : 10/10 [00:01<00:00, 8.61it/s]
100%|###################################################################################################################################| Fold : 10/10 [00:01<00:00, 8.62it/s]
25%|################################2 | n_interp : 1/4 [00:01<00:03, 1.27s/it]
0%| | Repairing epochs : 0/20 [00:00<?, ?it/s]
15%|################## | Repairing epochs : 3/20 [00:00<00:00, 181.37it/s]
35%|########################################## | Repairing epochs : 7/20 [00:00<00:00, 202.98it/s]
55%|#################################################################4 | Repairing epochs : 11/20 [00:00<00:00, 208.17it/s]
75%|#########################################################################################2 | Repairing epochs : 15/20 [00:00<00:00, 217.82it/s]
90%|###########################################################################################################1 | Repairing epochs : 18/20 [00:00<00:00, 196.16it/s]
100%|#######################################################################################################################| Repairing epochs : 20/20 [00:00<00:00, 207.78it/s]
0%| | Fold : 0/10 [00:00<?, ?it/s]
10%|#############2 | Fold : 1/10 [00:00<00:00, 9.12it/s]
20%|##########################4 | Fold : 2/10 [00:00<00:00, 9.00it/s]
30%|#######################################6 | Fold : 3/10 [00:00<00:00, 8.92it/s]
40%|####################################################8 | Fold : 4/10 [00:00<00:00, 8.80it/s]
50%|################################################################## | Fold : 5/10 [00:00<00:00, 8.76it/s]
60%|###############################################################################2 | Fold : 6/10 [00:00<00:00, 8.76it/s]
70%|############################################################################################3 | Fold : 7/10 [00:00<00:00, 8.74it/s]
80%|#########################################################################################################6 | Fold : 8/10 [00:00<00:00, 8.74it/s]
90%|######################################################################################################################8 | Fold : 9/10 [00:01<00:00, 8.56it/s]
100%|###################################################################################################################################| Fold : 10/10 [00:01<00:00, 8.57it/s]
100%|###################################################################################################################################| Fold : 10/10 [00:01<00:00, 8.61it/s]
50%|################################################################5 | n_interp : 2/4 [00:02<00:02, 1.27s/it]
0%| | Repairing epochs : 0/20 [00:00<?, ?it/s]
20%|######################## | Repairing epochs : 4/20 [00:00<00:00, 249.50it/s]
40%|################################################ | Repairing epochs : 8/20 [00:00<00:00, 245.66it/s]
60%|#######################################################################3 | Repairing epochs : 12/20 [00:00<00:00, 244.35it/s]
80%|###############################################################################################2 | Repairing epochs : 16/20 [00:00<00:00, 220.23it/s]
100%|#######################################################################################################################| Repairing epochs : 20/20 [00:00<00:00, 222.19it/s]
100%|#######################################################################################################################| Repairing epochs : 20/20 [00:00<00:00, 223.36it/s]
0%| | Fold : 0/10 [00:00<?, ?it/s]
10%|#############2 | Fold : 1/10 [00:00<00:01, 8.67it/s]
20%|##########################4 | Fold : 2/10 [00:00<00:00, 8.68it/s]
30%|#######################################6 | Fold : 3/10 [00:00<00:00, 8.69it/s]
40%|####################################################8 | Fold : 4/10 [00:00<00:00, 8.69it/s]
50%|################################################################## | Fold : 5/10 [00:00<00:00, 8.59it/s]
60%|###############################################################################2 | Fold : 6/10 [00:00<00:00, 8.57it/s]
70%|############################################################################################3 | Fold : 7/10 [00:00<00:00, 8.52it/s]
80%|#########################################################################################################6 | Fold : 8/10 [00:00<00:00, 8.55it/s]
90%|######################################################################################################################8 | Fold : 9/10 [00:01<00:00, 8.54it/s]
100%|###################################################################################################################################| Fold : 10/10 [00:01<00:00, 8.52it/s]
100%|###################################################################################################################################| Fold : 10/10 [00:01<00:00, 8.53it/s]
75%|################################################################################################7 | n_interp : 3/4 [00:03<00:01, 1.27s/it]
0%| | Repairing epochs : 0/20 [00:00<?, ?it/s]
20%|######################## | Repairing epochs : 4/20 [00:00<00:00, 234.67it/s]
40%|################################################ | Repairing epochs : 8/20 [00:00<00:00, 227.89it/s]
60%|#######################################################################3 | Repairing epochs : 12/20 [00:00<00:00, 223.62it/s]
75%|#########################################################################################2 | Repairing epochs : 15/20 [00:00<00:00, 214.55it/s]
90%|###########################################################################################################1 | Repairing epochs : 18/20 [00:00<00:00, 190.40it/s]
100%|#######################################################################################################################| Repairing epochs : 20/20 [00:00<00:00, 202.68it/s]
0%| | Fold : 0/10 [00:00<?, ?it/s]
10%|#############2 | Fold : 1/10 [00:00<00:00, 9.15it/s]
20%|##########################4 | Fold : 2/10 [00:00<00:00, 8.95it/s]
30%|#######################################6 | Fold : 3/10 [00:00<00:00, 8.45it/s]
40%|####################################################8 | Fold : 4/10 [00:00<00:00, 8.64it/s]
50%|################################################################## | Fold : 5/10 [00:00<00:00, 8.75it/s]
60%|###############################################################################2 | Fold : 6/10 [00:00<00:00, 8.62it/s]
70%|############################################################################################3 | Fold : 7/10 [00:00<00:00, 8.55it/s]
80%|#########################################################################################################6 | Fold : 8/10 [00:00<00:00, 8.39it/s]
90%|######################################################################################################################8 | Fold : 9/10 [00:01<00:00, 8.40it/s]
100%|###################################################################################################################################| Fold : 10/10 [00:01<00:00, 8.22it/s]
100%|###################################################################################################################################| Fold : 10/10 [00:01<00:00, 8.28it/s]
100%|#################################################################################################################################| n_interp : 4/4 [00:05<00:00, 1.28s/it]
100%|#################################################################################################################################| n_interp : 4/4 [00:05<00:00, 1.28s/it]
Estimated consensus=0.10 and n_interpolate=1
0%| | Repairing epochs : 0/97 [00:00<?, ?it/s]
3%|###7 | Repairing epochs : 3/97 [00:00<00:00, 176.14it/s]
7%|########6 | Repairing epochs : 7/97 [00:00<00:00, 157.91it/s]
10%|############2 | Repairing epochs : 10/97 [00:00<00:00, 145.95it/s]
15%|##################4 | Repairing epochs : 15/97 [00:00<00:00, 145.73it/s]
19%|###################### | Repairing epochs : 18/97 [00:00<00:00, 136.47it/s]
25%|#############################4 | Repairing epochs : 24/97 [00:00<00:00, 155.39it/s]
28%|#################################1 | Repairing epochs : 27/97 [00:00<00:00, 151.04it/s]
31%|####################################8 | Repairing epochs : 30/97 [00:00<00:00, 154.60it/s]
34%|########################################4 | Repairing epochs : 33/97 [00:00<00:00, 157.20it/s]
36%|##########################################9 | Repairing epochs : 35/97 [00:00<00:00, 150.15it/s]
40%|###############################################8 | Repairing epochs : 39/97 [00:00<00:00, 150.57it/s]
44%|####################################################7 | Repairing epochs : 43/97 [00:00<00:00, 152.87it/s]
48%|#########################################################6 | Repairing epochs : 47/97 [00:00<00:00, 153.59it/s]
53%|##############################################################5 | Repairing epochs : 51/97 [00:00<00:00, 157.47it/s]
56%|##################################################################2 | Repairing epochs : 54/97 [00:00<00:00, 158.10it/s]
60%|#######################################################################1 | Repairing epochs : 58/97 [00:00<00:00, 158.32it/s]
63%|##########################################################################8 | Repairing epochs : 61/97 [00:00<00:00, 159.72it/s]
66%|##############################################################################5 | Repairing epochs : 64/97 [00:00<00:00, 157.31it/s]
68%|################################################################################9 | Repairing epochs : 66/97 [00:00<00:00, 153.71it/s]
70%|###################################################################################4 | Repairing epochs : 68/97 [00:00<00:00, 150.94it/s]
72%|#####################################################################################8 | Repairing epochs : 70/97 [00:00<00:00, 147.87it/s]
74%|########################################################################################3 | Repairing epochs : 72/97 [00:00<00:00, 144.82it/s]
76%|##########################################################################################7 | Repairing epochs : 74/97 [00:00<00:00, 141.29it/s]
78%|#############################################################################################2 | Repairing epochs : 76/97 [00:00<00:00, 139.46it/s]
80%|###############################################################################################6 | Repairing epochs : 78/97 [00:00<00:00, 137.76it/s]
82%|##################################################################################################1 | Repairing epochs : 80/97 [00:00<00:00, 135.97it/s]
85%|####################################################################################################5 | Repairing epochs : 82/97 [00:00<00:00, 134.51it/s]
87%|####################################################################################################### | Repairing epochs : 84/97 [00:00<00:00, 133.35it/s]
89%|#########################################################################################################5 | Repairing epochs : 86/97 [00:00<00:00, 131.23it/s]
91%|###########################################################################################################9 | Repairing epochs : 88/97 [00:00<00:00, 130.28it/s]
93%|##############################################################################################################4 | Repairing epochs : 90/97 [00:00<00:00, 128.38it/s]
95%|################################################################################################################8 | Repairing epochs : 92/97 [00:00<00:00, 127.81it/s]
97%|###################################################################################################################3 | Repairing epochs : 94/97 [00:00<00:00, 127.26it/s]
99%|#####################################################################################################################7 | Repairing epochs : 96/97 [00:00<00:00, 125.59it/s]
100%|#######################################################################################################################| Repairing epochs : 97/97 [00:00<00:00, 134.78it/s]
Dropped 27 epochs: 54, 58, 62, 63, 64, 65, 66, 67, 68, 69, 70, 71, 72, 73, 74, 75, 76, 77, 78, 79, 80, 86, 89, 91, 92, 95, 96
visualize the dropped epochs
epochs[reject_log.bad_epochs].plot(scalings=dict(eeg=100e-6))
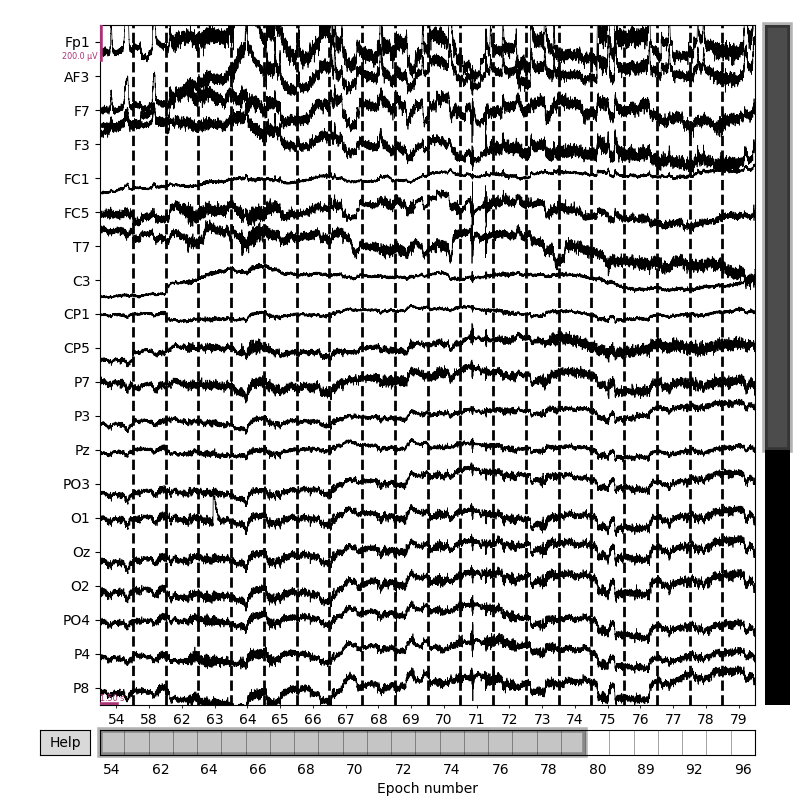
Using matplotlib as 2D backend.
<MNEBrowseFigure size 800x800 with 4 Axes>
and the reject log
reject_log.plot('horizontal')
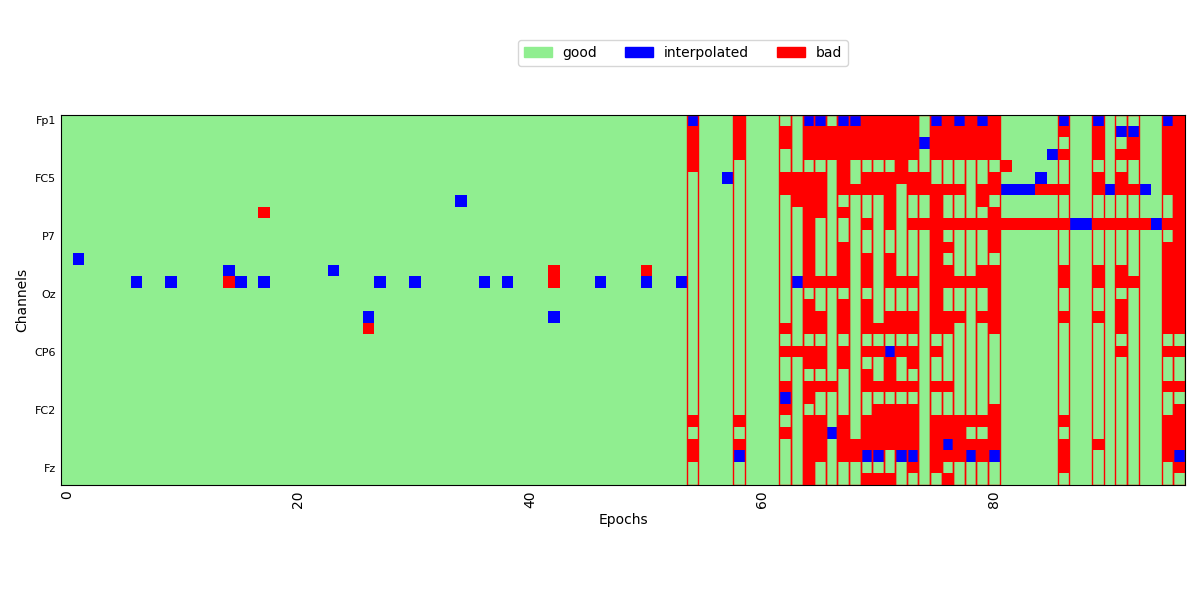
<Figure size 1200x600 with 1 Axes>
Autoreject with high-pass filter#
The data may be very valuable and the time for the experiment limited and so we may want to take steps to reduce the number of epochs dropped by first using other steps to preprocess the data. We will use a high-pass filter first to remove slow drift that could cause epochs to be dropped.
When making this decision to filter the data, we do want to be careful because filtering can spread sharp, high-frequency transients and distort the phase of the signal. Most evoked response potential analyses use filtering since the interest is in the time series, but if you are doing a frequency based analysis, filtering before the Fourier transform could potentially be avoided by detrending instead.
raw.filter(l_freq=1, h_freq=None)
epochs = mne.make_fixed_length_epochs(raw, duration=3, preload=True)
ar = autoreject.AutoReject(n_interpolate=[1, 2, 3, 4], random_state=11,
n_jobs=1, verbose=True)
ar.fit(epochs[:20]) # fit on a few epochs to save time
epochs_ar, reject_log = ar.transform(epochs, return_log=True)
Filtering raw data in 1 contiguous segment
Setting up high-pass filter at 1 Hz
FIR filter parameters
---------------------
Designing a one-pass, zero-phase, non-causal highpass filter:
- Windowed time-domain design (firwin) method
- Hamming window with 0.0194 passband ripple and 53 dB stopband attenuation
- Lower passband edge: 1.00
- Lower transition bandwidth: 1.00 Hz (-6 dB cutoff frequency: 0.50 Hz)
- Filter length: 1691 samples (3.303 s)
[Parallel(n_jobs=1)]: Using backend SequentialBackend with 1 concurrent workers.
[Parallel(n_jobs=1)]: Done 1 out of 1 | elapsed: 0.0s remaining: 0.0s
[Parallel(n_jobs=1)]: Done 2 out of 2 | elapsed: 0.0s remaining: 0.0s
[Parallel(n_jobs=1)]: Done 3 out of 3 | elapsed: 0.0s remaining: 0.0s
[Parallel(n_jobs=1)]: Done 4 out of 4 | elapsed: 0.0s remaining: 0.0s
[Parallel(n_jobs=1)]: Done 32 out of 32 | elapsed: 0.0s finished
Not setting metadata
97 matching events found
No baseline correction applied
0 projection items activated
Using data from preloaded Raw for 97 events and 1536 original time points ...
0 bad epochs dropped
Running autoreject on ch_type=eeg
0%| | Creating augmented epochs : 0/32 [00:00<?, ?it/s]
6%|######9 | Creating augmented epochs : 2/32 [00:00<00:00, 88.33it/s]
12%|#############8 | Creating augmented epochs : 4/32 [00:00<00:00, 84.85it/s]
19%|####################8 | Creating augmented epochs : 6/32 [00:00<00:00, 81.19it/s]
25%|###########################7 | Creating augmented epochs : 8/32 [00:00<00:00, 82.48it/s]
31%|##################################3 | Creating augmented epochs : 10/32 [00:00<00:00, 82.61it/s]
38%|#########################################2 | Creating augmented epochs : 12/32 [00:00<00:00, 82.02it/s]
44%|################################################1 | Creating augmented epochs : 14/32 [00:00<00:00, 81.12it/s]
50%|####################################################### | Creating augmented epochs : 16/32 [00:00<00:00, 81.17it/s]
53%|##########################################################4 | Creating augmented epochs : 17/32 [00:00<00:00, 79.03it/s]
59%|#################################################################3 | Creating augmented epochs : 19/32 [00:00<00:00, 79.14it/s]
66%|########################################################################1 | Creating augmented epochs : 21/32 [00:00<00:00, 79.78it/s]
72%|############################################################################### | Creating augmented epochs : 23/32 [00:00<00:00, 79.94it/s]
78%|#####################################################################################9 | Creating augmented epochs : 25/32 [00:00<00:00, 80.42it/s]
84%|############################################################################################8 | Creating augmented epochs : 27/32 [00:00<00:00, 80.70it/s]
91%|###################################################################################################6 | Creating augmented epochs : 29/32 [00:00<00:00, 80.88it/s]
97%|##########################################################################################################5 | Creating augmented epochs : 31/32 [00:00<00:00, 80.63it/s]
100%|##############################################################################################################| Creating augmented epochs : 32/32 [00:00<00:00, 80.84it/s]
0%| | Computing thresholds ... : 0/32 [00:00<?, ?it/s]
3%|###5 | Computing thresholds ... : 1/32 [00:00<00:12, 2.44it/s]
6%|####### | Computing thresholds ... : 2/32 [00:00<00:12, 2.45it/s]
9%|##########5 | Computing thresholds ... : 3/32 [00:01<00:11, 2.51it/s]
12%|############## | Computing thresholds ... : 4/32 [00:01<00:10, 2.56it/s]
16%|#################5 | Computing thresholds ... : 5/32 [00:01<00:10, 2.60it/s]
19%|##################### | Computing thresholds ... : 6/32 [00:02<00:09, 2.64it/s]
22%|########################5 | Computing thresholds ... : 7/32 [00:02<00:09, 2.65it/s]
25%|############################ | Computing thresholds ... : 8/32 [00:03<00:09, 2.66it/s]
28%|###############################5 | Computing thresholds ... : 9/32 [00:03<00:08, 2.68it/s]
31%|##################################6 | Computing thresholds ... : 10/32 [00:03<00:08, 2.68it/s]
34%|######################################1 | Computing thresholds ... : 11/32 [00:04<00:07, 2.69it/s]
38%|#########################################6 | Computing thresholds ... : 12/32 [00:04<00:07, 2.71it/s]
41%|############################################# | Computing thresholds ... : 13/32 [00:04<00:07, 2.70it/s]
44%|################################################5 | Computing thresholds ... : 14/32 [00:05<00:06, 2.72it/s]
47%|#################################################### | Computing thresholds ... : 15/32 [00:05<00:06, 2.73it/s]
50%|#######################################################5 | Computing thresholds ... : 16/32 [00:05<00:05, 2.72it/s]
53%|##########################################################9 | Computing thresholds ... : 17/32 [00:06<00:05, 2.74it/s]
56%|##############################################################4 | Computing thresholds ... : 18/32 [00:06<00:05, 2.75it/s]
59%|#################################################################9 | Computing thresholds ... : 19/32 [00:06<00:04, 2.74it/s]
62%|#####################################################################3 | Computing thresholds ... : 20/32 [00:07<00:04, 2.75it/s]
66%|########################################################################8 | Computing thresholds ... : 21/32 [00:07<00:04, 2.75it/s]
69%|############################################################################3 | Computing thresholds ... : 22/32 [00:08<00:03, 2.75it/s]
72%|###############################################################################7 | Computing thresholds ... : 23/32 [00:08<00:03, 2.76it/s]
75%|###################################################################################2 | Computing thresholds ... : 24/32 [00:08<00:02, 2.76it/s]
78%|######################################################################################7 | Computing thresholds ... : 25/32 [00:09<00:02, 2.75it/s]
81%|##########################################################################################1 | Computing thresholds ... : 26/32 [00:09<00:02, 2.76it/s]
84%|#############################################################################################6 | Computing thresholds ... : 27/32 [00:09<00:01, 2.76it/s]
88%|#################################################################################################1 | Computing thresholds ... : 28/32 [00:10<00:01, 2.76it/s]
91%|####################################################################################################5 | Computing thresholds ... : 29/32 [00:10<00:01, 2.77it/s]
94%|######################################################################################################## | Computing thresholds ... : 30/32 [00:10<00:00, 2.77it/s]
97%|###########################################################################################################5 | Computing thresholds ... : 31/32 [00:11<00:00, 2.77it/s]
100%|###############################################################################################################| Computing thresholds ... : 32/32 [00:11<00:00, 2.78it/s]
100%|###############################################################################################################| Computing thresholds ... : 32/32 [00:11<00:00, 2.75it/s]
0%| | Repairing epochs : 0/20 [00:00<?, ?it/s]
35%|########################################## | Repairing epochs : 7/20 [00:00<00:00, 436.67it/s]
75%|#########################################################################################2 | Repairing epochs : 15/20 [00:00<00:00, 440.31it/s]
100%|#######################################################################################################################| Repairing epochs : 20/20 [00:00<00:00, 429.33it/s]
0%| | n_interp : 0/4 [00:00<?, ?it/s]
0%| | Repairing epochs : 0/20 [00:00<?, ?it/s]
10%|############ | Repairing epochs : 2/20 [00:00<00:00, 108.07it/s]
25%|############################## | Repairing epochs : 5/20 [00:00<00:00, 130.26it/s]
35%|########################################## | Repairing epochs : 7/20 [00:00<00:00, 119.34it/s]
50%|###########################################################5 | Repairing epochs : 10/20 [00:00<00:00, 125.87it/s]
60%|#######################################################################3 | Repairing epochs : 12/20 [00:00<00:00, 124.94it/s]
75%|#########################################################################################2 | Repairing epochs : 15/20 [00:00<00:00, 123.74it/s]
85%|#####################################################################################################1 | Repairing epochs : 17/20 [00:00<00:00, 113.46it/s]
95%|################################################################################################################# | Repairing epochs : 19/20 [00:00<00:00, 109.25it/s]
100%|#######################################################################################################################| Repairing epochs : 20/20 [00:00<00:00, 110.69it/s]
0%| | Fold : 0/10 [00:00<?, ?it/s]
10%|#############2 | Fold : 1/10 [00:00<00:01, 8.39it/s]
20%|##########################4 | Fold : 2/10 [00:00<00:00, 8.34it/s]
30%|#######################################6 | Fold : 3/10 [00:00<00:00, 8.48it/s]
40%|####################################################8 | Fold : 4/10 [00:00<00:00, 8.54it/s]
50%|################################################################## | Fold : 5/10 [00:00<00:00, 8.60it/s]
60%|###############################################################################2 | Fold : 6/10 [00:00<00:00, 8.60it/s]
70%|############################################################################################3 | Fold : 7/10 [00:00<00:00, 8.63it/s]
80%|#########################################################################################################6 | Fold : 8/10 [00:00<00:00, 8.68it/s]
90%|######################################################################################################################8 | Fold : 9/10 [00:01<00:00, 8.53it/s]
100%|###################################################################################################################################| Fold : 10/10 [00:01<00:00, 8.53it/s]
100%|###################################################################################################################################| Fold : 10/10 [00:01<00:00, 8.54it/s]
25%|################################2 | n_interp : 1/4 [00:01<00:04, 1.37s/it]
0%| | Repairing epochs : 0/20 [00:00<?, ?it/s]
10%|############ | Repairing epochs : 2/20 [00:00<00:00, 86.71it/s]
25%|############################## | Repairing epochs : 5/20 [00:00<00:00, 110.31it/s]
35%|########################################## | Repairing epochs : 7/20 [00:00<00:00, 105.46it/s]
60%|#######################################################################3 | Repairing epochs : 12/20 [00:00<00:00, 132.43it/s]
75%|#########################################################################################2 | Repairing epochs : 15/20 [00:00<00:00, 130.05it/s]
85%|#####################################################################################################1 | Repairing epochs : 17/20 [00:00<00:00, 126.63it/s]
95%|################################################################################################################# | Repairing epochs : 19/20 [00:00<00:00, 125.44it/s]
100%|#######################################################################################################################| Repairing epochs : 20/20 [00:00<00:00, 122.64it/s]
0%| | Fold : 0/10 [00:00<?, ?it/s]
10%|#############2 | Fold : 1/10 [00:00<00:01, 8.09it/s]
20%|##########################4 | Fold : 2/10 [00:00<00:00, 8.31it/s]
30%|#######################################6 | Fold : 3/10 [00:00<00:00, 8.32it/s]
40%|####################################################8 | Fold : 4/10 [00:00<00:00, 8.36it/s]
50%|################################################################## | Fold : 5/10 [00:00<00:00, 8.36it/s]
60%|###############################################################################2 | Fold : 6/10 [00:00<00:00, 8.17it/s]
70%|############################################################################################3 | Fold : 7/10 [00:00<00:00, 8.12it/s]
80%|#########################################################################################################6 | Fold : 8/10 [00:00<00:00, 8.18it/s]
90%|######################################################################################################################8 | Fold : 9/10 [00:01<00:00, 8.19it/s]
100%|###################################################################################################################################| Fold : 10/10 [00:01<00:00, 8.24it/s]
100%|###################################################################################################################################| Fold : 10/10 [00:01<00:00, 8.23it/s]
50%|################################################################5 | n_interp : 2/4 [00:02<00:02, 1.39s/it]
0%| | Repairing epochs : 0/20 [00:00<?, ?it/s]
10%|############ | Repairing epochs : 2/20 [00:00<00:00, 98.11it/s]
25%|############################## | Repairing epochs : 5/20 [00:00<00:00, 121.34it/s]
35%|########################################## | Repairing epochs : 7/20 [00:00<00:00, 109.20it/s]
50%|###########################################################5 | Repairing epochs : 10/20 [00:00<00:00, 122.74it/s]
65%|#############################################################################3 | Repairing epochs : 13/20 [00:00<00:00, 134.37it/s]
75%|#########################################################################################2 | Repairing epochs : 15/20 [00:00<00:00, 121.96it/s]
85%|#####################################################################################################1 | Repairing epochs : 17/20 [00:00<00:00, 120.33it/s]
95%|################################################################################################################# | Repairing epochs : 19/20 [00:00<00:00, 116.43it/s]
100%|#######################################################################################################################| Repairing epochs : 20/20 [00:00<00:00, 115.19it/s]
0%| | Fold : 0/10 [00:00<?, ?it/s]
10%|#############2 | Fold : 1/10 [00:00<00:01, 8.87it/s]
20%|##########################4 | Fold : 2/10 [00:00<00:00, 8.76it/s]
30%|#######################################6 | Fold : 3/10 [00:00<00:00, 8.75it/s]
40%|####################################################8 | Fold : 4/10 [00:00<00:00, 8.69it/s]
50%|################################################################## | Fold : 5/10 [00:00<00:00, 8.77it/s]
60%|###############################################################################2 | Fold : 6/10 [00:00<00:00, 8.63it/s]
70%|############################################################################################3 | Fold : 7/10 [00:00<00:00, 8.43it/s]
80%|#########################################################################################################6 | Fold : 8/10 [00:00<00:00, 8.35it/s]
90%|######################################################################################################################8 | Fold : 9/10 [00:01<00:00, 8.42it/s]
100%|###################################################################################################################################| Fold : 10/10 [00:01<00:00, 8.45it/s]
100%|###################################################################################################################################| Fold : 10/10 [00:01<00:00, 8.46it/s]
75%|################################################################################################7 | n_interp : 3/4 [00:04<00:01, 1.39s/it]
0%| | Repairing epochs : 0/20 [00:00<?, ?it/s]
10%|############ | Repairing epochs : 2/20 [00:00<00:00, 100.44it/s]
25%|############################## | Repairing epochs : 5/20 [00:00<00:00, 124.22it/s]
35%|########################################## | Repairing epochs : 7/20 [00:00<00:00, 115.76it/s]
55%|#################################################################4 | Repairing epochs : 11/20 [00:00<00:00, 143.22it/s]
70%|###################################################################################3 | Repairing epochs : 14/20 [00:00<00:00, 139.27it/s]
80%|###############################################################################################2 | Repairing epochs : 16/20 [00:00<00:00, 128.34it/s]
90%|###########################################################################################################1 | Repairing epochs : 18/20 [00:00<00:00, 122.84it/s]
100%|#######################################################################################################################| Repairing epochs : 20/20 [00:00<00:00, 117.12it/s]
100%|#######################################################################################################################| Repairing epochs : 20/20 [00:00<00:00, 118.82it/s]
0%| | Fold : 0/10 [00:00<?, ?it/s]
10%|#############2 | Fold : 1/10 [00:00<00:00, 9.57it/s]
20%|##########################4 | Fold : 2/10 [00:00<00:00, 9.48it/s]
30%|#######################################6 | Fold : 3/10 [00:00<00:00, 9.43it/s]
40%|####################################################8 | Fold : 4/10 [00:00<00:00, 9.49it/s]
50%|################################################################## | Fold : 5/10 [00:00<00:00, 9.51it/s]
60%|###############################################################################2 | Fold : 6/10 [00:00<00:00, 9.52it/s]
70%|############################################################################################3 | Fold : 7/10 [00:00<00:00, 9.46it/s]
80%|#########################################################################################################6 | Fold : 8/10 [00:00<00:00, 9.40it/s]
90%|######################################################################################################################8 | Fold : 9/10 [00:00<00:00, 9.41it/s]
100%|###################################################################################################################################| Fold : 10/10 [00:01<00:00, 9.31it/s]
100%|###################################################################################################################################| Fold : 10/10 [00:01<00:00, 9.34it/s]
100%|#################################################################################################################################| n_interp : 4/4 [00:05<00:00, 1.35s/it]
100%|#################################################################################################################################| n_interp : 4/4 [00:05<00:00, 1.35s/it]
Estimated consensus=0.40 and n_interpolate=4
0%| | Repairing epochs : 0/97 [00:00<?, ?it/s]
2%|##4 | Repairing epochs : 2/97 [00:00<00:00, 107.92it/s]
5%|######1 | Repairing epochs : 5/97 [00:00<00:00, 131.97it/s]
7%|########6 | Repairing epochs : 7/97 [00:00<00:00, 116.13it/s]
11%|#############4 | Repairing epochs : 11/97 [00:00<00:00, 141.16it/s]
14%|#################1 | Repairing epochs : 14/97 [00:00<00:00, 136.19it/s]
16%|###################6 | Repairing epochs : 16/97 [00:00<00:00, 129.50it/s]
19%|###################### | Repairing epochs : 18/97 [00:00<00:00, 125.52it/s]
21%|########################5 | Repairing epochs : 20/97 [00:00<00:00, 122.60it/s]
23%|##########################9 | Repairing epochs : 22/97 [00:00<00:00, 120.37it/s]
25%|#############################4 | Repairing epochs : 24/97 [00:00<00:00, 118.56it/s]
27%|###############################8 | Repairing epochs : 26/97 [00:00<00:00, 116.64it/s]
29%|##################################3 | Repairing epochs : 28/97 [00:00<00:00, 115.21it/s]
31%|####################################8 | Repairing epochs : 30/97 [00:00<00:00, 113.03it/s]
33%|#######################################2 | Repairing epochs : 32/97 [00:00<00:00, 112.32it/s]
35%|#########################################7 | Repairing epochs : 34/97 [00:00<00:00, 111.14it/s]
37%|############################################1 | Repairing epochs : 36/97 [00:00<00:00, 110.27it/s]
39%|##############################################6 | Repairing epochs : 38/97 [00:00<00:00, 108.45it/s]
41%|################################################# | Repairing epochs : 40/97 [00:00<00:00, 107.64it/s]
43%|###################################################5 | Repairing epochs : 42/97 [00:00<00:00, 106.96it/s]
45%|#####################################################9 | Repairing epochs : 44/97 [00:00<00:00, 106.87it/s]
47%|########################################################4 | Repairing epochs : 46/97 [00:00<00:00, 106.26it/s]
49%|##########################################################8 | Repairing epochs : 48/97 [00:00<00:00, 106.42it/s]
52%|#############################################################3 | Repairing epochs : 50/97 [00:00<00:00, 103.68it/s]
54%|###############################################################7 | Repairing epochs : 52/97 [00:00<00:00, 102.33it/s]
56%|##################################################################2 | Repairing epochs : 54/97 [00:00<00:00, 102.00it/s]
58%|####################################################################7 | Repairing epochs : 56/97 [00:00<00:00, 102.36it/s]
60%|#######################################################################1 | Repairing epochs : 58/97 [00:00<00:00, 102.47it/s]
62%|#########################################################################6 | Repairing epochs : 60/97 [00:00<00:00, 102.30it/s]
64%|############################################################################ | Repairing epochs : 62/97 [00:00<00:00, 102.00it/s]
66%|##############################################################################5 | Repairing epochs : 64/97 [00:00<00:00, 100.59it/s]
68%|################################################################################9 | Repairing epochs : 66/97 [00:00<00:00, 100.69it/s]
70%|###################################################################################4 | Repairing epochs : 68/97 [00:00<00:00, 101.08it/s]
72%|#####################################################################################8 | Repairing epochs : 70/97 [00:00<00:00, 101.34it/s]
74%|########################################################################################3 | Repairing epochs : 72/97 [00:00<00:00, 101.25it/s]
76%|##########################################################################################7 | Repairing epochs : 74/97 [00:00<00:00, 101.07it/s]
78%|#############################################################################################2 | Repairing epochs : 76/97 [00:00<00:00, 101.33it/s]
80%|###############################################################################################6 | Repairing epochs : 78/97 [00:00<00:00, 101.54it/s]
82%|##################################################################################################1 | Repairing epochs : 80/97 [00:00<00:00, 101.74it/s]
85%|####################################################################################################5 | Repairing epochs : 82/97 [00:00<00:00, 102.66it/s]
87%|####################################################################################################### | Repairing epochs : 84/97 [00:00<00:00, 102.51it/s]
89%|#########################################################################################################5 | Repairing epochs : 86/97 [00:00<00:00, 102.53it/s]
91%|###########################################################################################################9 | Repairing epochs : 88/97 [00:00<00:00, 102.67it/s]
93%|##############################################################################################################4 | Repairing epochs : 90/97 [00:00<00:00, 103.25it/s]
95%|################################################################################################################8 | Repairing epochs : 92/97 [00:00<00:00, 103.05it/s]
97%|###################################################################################################################3 | Repairing epochs : 94/97 [00:00<00:00, 101.86it/s]
99%|#####################################################################################################################7 | Repairing epochs : 96/97 [00:00<00:00, 100.63it/s]
100%|#######################################################################################################################| Repairing epochs : 97/97 [00:00<00:00, 103.57it/s]
Dropped 23 epochs: 62, 63, 64, 65, 67, 68, 69, 70, 71, 72, 73, 75, 76, 77, 78, 79, 80, 86, 89, 91, 92, 95, 96
visualize the dropped epochs
epochs[reject_log.bad_epochs].plot(scalings=dict(eeg=100e-6))
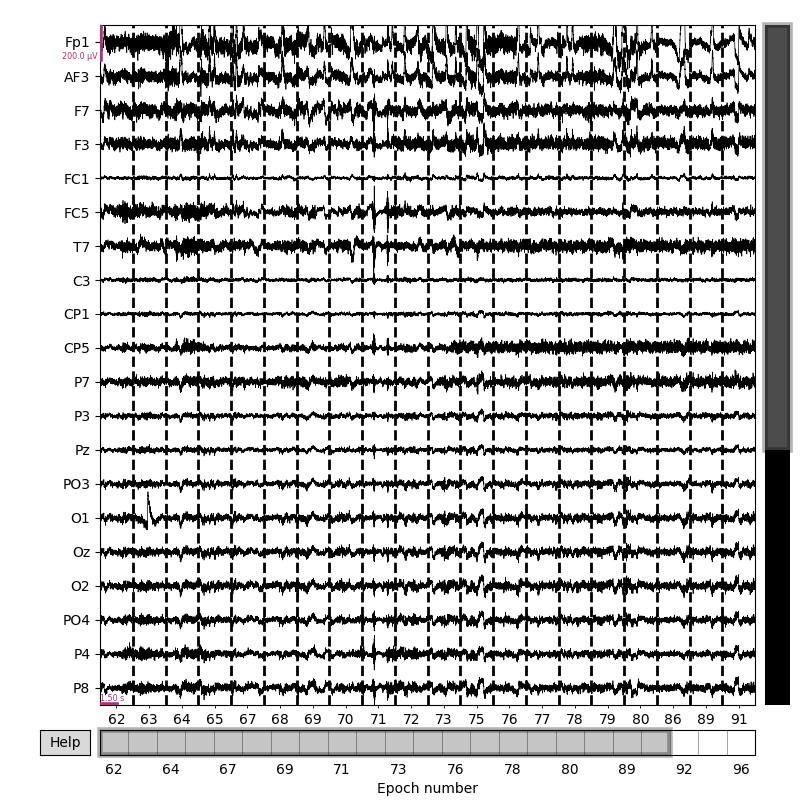
<MNEBrowseFigure size 800x800 with 4 Axes>
and the reject log. As we can see in the plot, high-pass filtering reduced the number of epochs marked as bad by autoreject substantially.
reject_log.plot('horizontal')
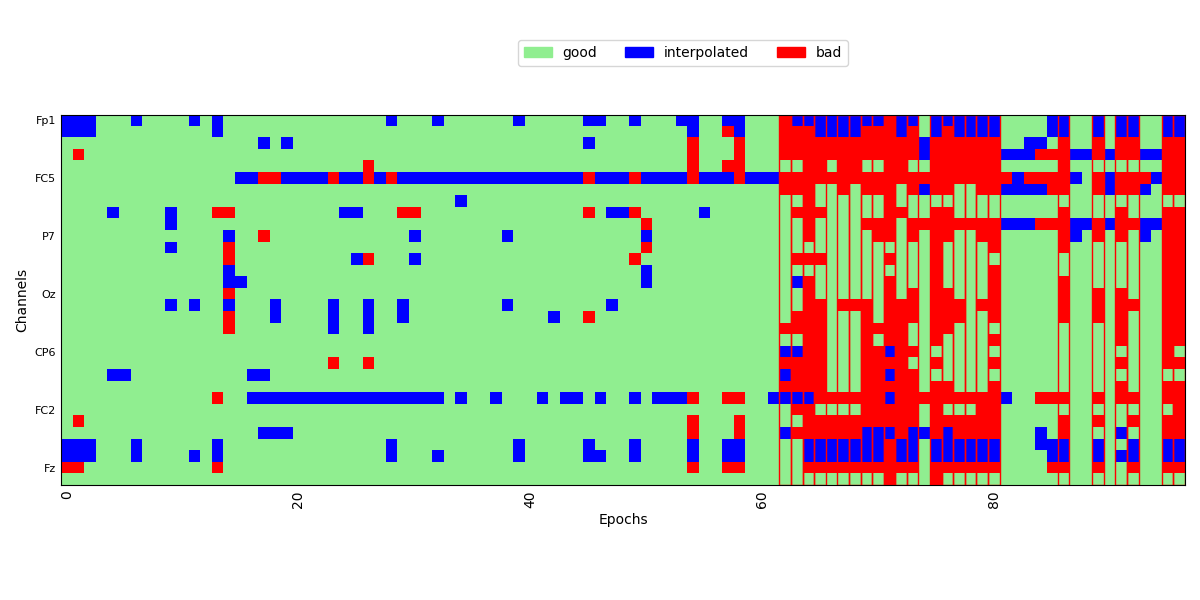
<Figure size 1200x600 with 1 Axes>
ICA#
Finally, we can apply independent components analysis (ICA) to remove eyeblinks from the data. If our analysis were to be very dependent on sensors at the front of the head, we could skip ICA and use the previous result. However, ICA can increase the amount of usable data by applying a spatial filter that downscales the data in sensors most affected by eyeblink artifacts.
Note that ICA works best if bad segments of the data are removed Hence, we will remove the bad segments from the previous run of autoreject for the benefit of the ICA algorithm.
# compute ICA
ica = mne.preprocessing.ICA(random_state=99)
ica.fit(epochs[~reject_log.bad_epochs])
Fitting ICA to data using 32 channels (please be patient, this may take a while)
Selecting by non-zero PCA components: 32 components
Fitting ICA took 5.6s.
We can see in the plots below that ICA effectively removed eyeblink artifact.
plot source components to see which is made up of blinks
exclude = [0, # blinks
2 # saccades
]
ica.plot_components(exclude)
ica.exclude = exclude
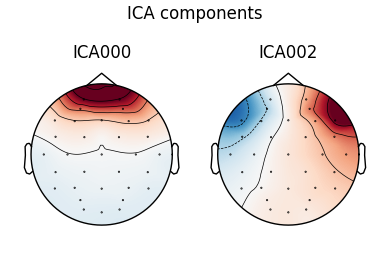
plot with and without eyeblink component
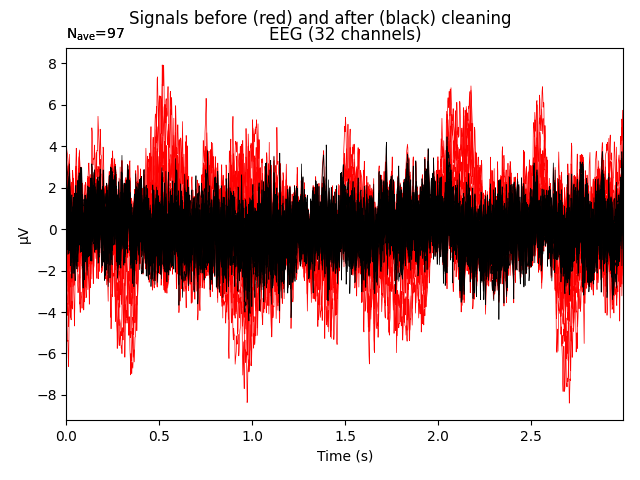
NOTE: pick_channels() is a legacy function. New code should use inst.pick(...).
NOTE: pick_channels() is a legacy function. New code should use inst.pick(...).
Applying ICA to Evoked instance
Transforming to ICA space (32 components)
Zeroing out 2 ICA components
Projecting back using 32 PCA components
Applying ICA to Epochs instance
Transforming to ICA space (32 components)
Zeroing out 2 ICA components
Projecting back using 32 PCA components
Autoreject with highpass filter and ICA#
We can see in this section that preprocessing, especially ICA, can be made to do a lot of the heavy lifting. There isn’t a huge difference when viewing the averaged data because the ICA effectively limited the number of epochs that had to be dropped. However, there are still artifacts such as non-stereotypical blinks that weren’t able to be removed by ICA, channel “pops” (sharp transients with exponential RC decay), muscle artifact such as jaw clenches and gross movement artifact that could still impact analyses.
These are the basic steps for a workflow with decisions that must be
made based on what the data is being used for. Following this may help
you optimize your use of autoreject
in preprocessing.
# compute channel-level rejections
ar = autoreject.AutoReject(n_interpolate=[1, 2, 3, 4], random_state=11,
n_jobs=1, verbose=True)
ar.fit(epochs[:20]) # fit on the first 20 epochs to save time
epochs_ar, reject_log = ar.transform(epochs, return_log=True)
epochs[reject_log.bad_epochs].plot(scalings=dict(eeg=100e-6))
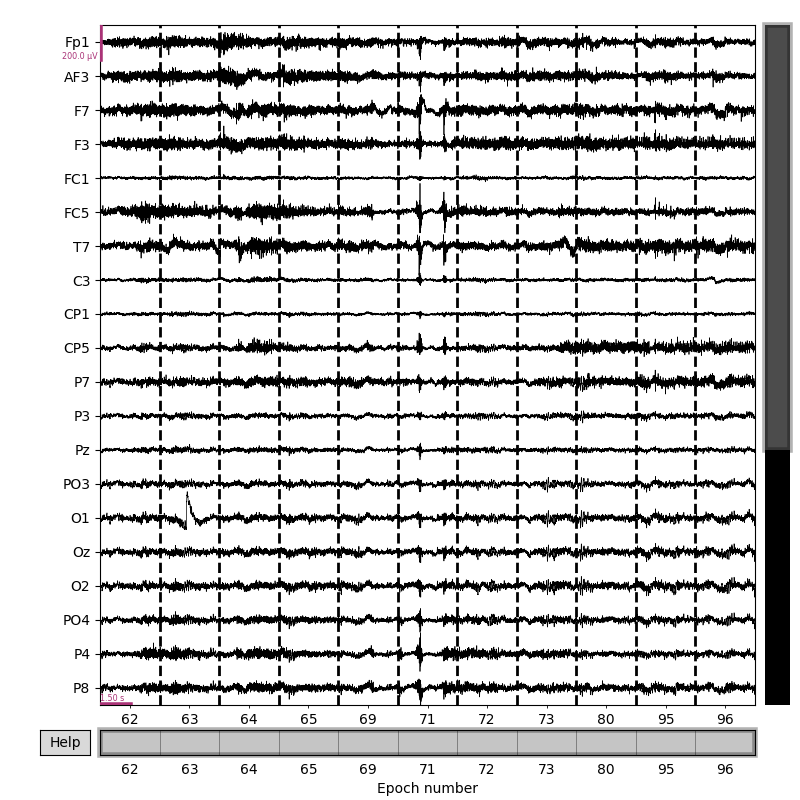
Running autoreject on ch_type=eeg
0%| | Creating augmented epochs : 0/32 [00:00<?, ?it/s]
6%|######9 | Creating augmented epochs : 2/32 [00:00<00:00, 86.90it/s]
12%|#############8 | Creating augmented epochs : 4/32 [00:00<00:00, 81.46it/s]
19%|####################8 | Creating augmented epochs : 6/32 [00:00<00:00, 80.90it/s]
25%|###########################7 | Creating augmented epochs : 8/32 [00:00<00:00, 83.34it/s]
31%|##################################3 | Creating augmented epochs : 10/32 [00:00<00:00, 82.57it/s]
38%|#########################################2 | Creating augmented epochs : 12/32 [00:00<00:00, 82.92it/s]
44%|################################################1 | Creating augmented epochs : 14/32 [00:00<00:00, 82.12it/s]
50%|####################################################### | Creating augmented epochs : 16/32 [00:00<00:00, 82.28it/s]
56%|#############################################################8 | Creating augmented epochs : 18/32 [00:00<00:00, 82.39it/s]
62%|####################################################################7 | Creating augmented epochs : 20/32 [00:00<00:00, 82.49it/s]
69%|###########################################################################6 | Creating augmented epochs : 22/32 [00:00<00:00, 81.78it/s]
75%|##################################################################################5 | Creating augmented epochs : 24/32 [00:00<00:00, 81.74it/s]
81%|#########################################################################################3 | Creating augmented epochs : 26/32 [00:00<00:00, 81.78it/s]
88%|################################################################################################2 | Creating augmented epochs : 28/32 [00:00<00:00, 82.25it/s]
94%|#######################################################################################################1 | Creating augmented epochs : 30/32 [00:00<00:00, 82.23it/s]
100%|##############################################################################################################| Creating augmented epochs : 32/32 [00:00<00:00, 82.24it/s]
100%|##############################################################################################################| Creating augmented epochs : 32/32 [00:00<00:00, 82.23it/s]
0%| | Computing thresholds ... : 0/32 [00:00<?, ?it/s]
3%|###5 | Computing thresholds ... : 1/32 [00:00<00:10, 2.84it/s]
6%|####### | Computing thresholds ... : 2/32 [00:00<00:10, 2.75it/s]
9%|##########5 | Computing thresholds ... : 3/32 [00:01<00:10, 2.80it/s]
12%|############## | Computing thresholds ... : 4/32 [00:01<00:09, 2.81it/s]
16%|#################5 | Computing thresholds ... : 5/32 [00:01<00:09, 2.79it/s]
19%|##################### | Computing thresholds ... : 6/32 [00:02<00:09, 2.79it/s]
22%|########################5 | Computing thresholds ... : 7/32 [00:02<00:08, 2.80it/s]
25%|############################ | Computing thresholds ... : 8/32 [00:02<00:08, 2.78it/s]
28%|###############################5 | Computing thresholds ... : 9/32 [00:03<00:08, 2.75it/s]
31%|##################################6 | Computing thresholds ... : 10/32 [00:03<00:08, 2.72it/s]
34%|######################################1 | Computing thresholds ... : 11/32 [00:04<00:07, 2.71it/s]
38%|#########################################6 | Computing thresholds ... : 12/32 [00:04<00:07, 2.64it/s]
41%|############################################# | Computing thresholds ... : 13/32 [00:04<00:07, 2.63it/s]
44%|################################################5 | Computing thresholds ... : 14/32 [00:05<00:06, 2.61it/s]
47%|#################################################### | Computing thresholds ... : 15/32 [00:05<00:06, 2.63it/s]
50%|#######################################################5 | Computing thresholds ... : 16/32 [00:06<00:06, 2.65it/s]
53%|##########################################################9 | Computing thresholds ... : 17/32 [00:06<00:05, 2.67it/s]
56%|##############################################################4 | Computing thresholds ... : 18/32 [00:06<00:05, 2.68it/s]
59%|#################################################################9 | Computing thresholds ... : 19/32 [00:07<00:04, 2.68it/s]
62%|#####################################################################3 | Computing thresholds ... : 20/32 [00:07<00:04, 2.69it/s]
66%|########################################################################8 | Computing thresholds ... : 21/32 [00:07<00:04, 2.70it/s]
69%|############################################################################3 | Computing thresholds ... : 22/32 [00:08<00:03, 2.70it/s]
72%|###############################################################################7 | Computing thresholds ... : 23/32 [00:08<00:03, 2.71it/s]
75%|###################################################################################2 | Computing thresholds ... : 24/32 [00:08<00:02, 2.72it/s]
78%|######################################################################################7 | Computing thresholds ... : 25/32 [00:09<00:02, 2.72it/s]
81%|##########################################################################################1 | Computing thresholds ... : 26/32 [00:09<00:02, 2.73it/s]
84%|#############################################################################################6 | Computing thresholds ... : 27/32 [00:09<00:01, 2.74it/s]
88%|#################################################################################################1 | Computing thresholds ... : 28/32 [00:10<00:01, 2.74it/s]
91%|####################################################################################################5 | Computing thresholds ... : 29/32 [00:10<00:01, 2.75it/s]
94%|######################################################################################################## | Computing thresholds ... : 30/32 [00:10<00:00, 2.75it/s]
97%|###########################################################################################################5 | Computing thresholds ... : 31/32 [00:11<00:00, 2.76it/s]
100%|###############################################################################################################| Computing thresholds ... : 32/32 [00:11<00:00, 2.76it/s]
100%|###############################################################################################################| Computing thresholds ... : 32/32 [00:11<00:00, 2.74it/s]
0%| | Repairing epochs : 0/20 [00:00<?, ?it/s]
40%|################################################ | Repairing epochs : 8/20 [00:00<00:00, 443.60it/s]
75%|#########################################################################################2 | Repairing epochs : 15/20 [00:00<00:00, 433.89it/s]
100%|#######################################################################################################################| Repairing epochs : 20/20 [00:00<00:00, 435.96it/s]
0%| | n_interp : 0/4 [00:00<?, ?it/s]
0%| | Repairing epochs : 0/20 [00:00<?, ?it/s]
25%|############################## | Repairing epochs : 5/20 [00:00<00:00, 278.49it/s]
45%|###################################################### | Repairing epochs : 9/20 [00:00<00:00, 256.54it/s]
60%|#######################################################################3 | Repairing epochs : 12/20 [00:00<00:00, 215.76it/s]
75%|#########################################################################################2 | Repairing epochs : 15/20 [00:00<00:00, 191.32it/s]
85%|#####################################################################################################1 | Repairing epochs : 17/20 [00:00<00:00, 168.65it/s]
95%|################################################################################################################# | Repairing epochs : 19/20 [00:00<00:00, 156.25it/s]
100%|#######################################################################################################################| Repairing epochs : 20/20 [00:00<00:00, 157.27it/s]
0%| | Fold : 0/10 [00:00<?, ?it/s]
10%|#############2 | Fold : 1/10 [00:00<00:01, 8.77it/s]
20%|##########################4 | Fold : 2/10 [00:00<00:00, 8.82it/s]
30%|#######################################6 | Fold : 3/10 [00:00<00:00, 8.98it/s]
40%|####################################################8 | Fold : 4/10 [00:00<00:00, 9.06it/s]
50%|################################################################## | Fold : 5/10 [00:00<00:00, 9.16it/s]
60%|###############################################################################2 | Fold : 6/10 [00:00<00:00, 9.23it/s]
70%|############################################################################################3 | Fold : 7/10 [00:00<00:00, 9.18it/s]
80%|#########################################################################################################6 | Fold : 8/10 [00:00<00:00, 9.20it/s]
90%|######################################################################################################################8 | Fold : 9/10 [00:00<00:00, 9.24it/s]
100%|###################################################################################################################################| Fold : 10/10 [00:01<00:00, 9.20it/s]
100%|###################################################################################################################################| Fold : 10/10 [00:01<00:00, 9.18it/s]
25%|################################2 | n_interp : 1/4 [00:01<00:03, 1.23s/it]
0%| | Repairing epochs : 0/20 [00:00<?, ?it/s]
25%|############################## | Repairing epochs : 5/20 [00:00<00:00, 216.81it/s]
45%|###################################################### | Repairing epochs : 9/20 [00:00<00:00, 216.71it/s]
60%|#######################################################################3 | Repairing epochs : 12/20 [00:00<00:00, 181.20it/s]
75%|#########################################################################################2 | Repairing epochs : 15/20 [00:00<00:00, 166.88it/s]
85%|#####################################################################################################1 | Repairing epochs : 17/20 [00:00<00:00, 154.03it/s]
95%|################################################################################################################# | Repairing epochs : 19/20 [00:00<00:00, 148.39it/s]
100%|#######################################################################################################################| Repairing epochs : 20/20 [00:00<00:00, 147.27it/s]
0%| | Fold : 0/10 [00:00<?, ?it/s]
10%|#############2 | Fold : 1/10 [00:00<00:01, 8.78it/s]
20%|##########################4 | Fold : 2/10 [00:00<00:00, 8.99it/s]
30%|#######################################6 | Fold : 3/10 [00:00<00:00, 9.10it/s]
40%|####################################################8 | Fold : 4/10 [00:00<00:00, 9.13it/s]
50%|################################################################## | Fold : 5/10 [00:00<00:00, 9.11it/s]
60%|###############################################################################2 | Fold : 6/10 [00:00<00:00, 9.10it/s]
70%|############################################################################################3 | Fold : 7/10 [00:00<00:00, 9.10it/s]
80%|#########################################################################################################6 | Fold : 8/10 [00:00<00:00, 9.11it/s]
90%|######################################################################################################################8 | Fold : 9/10 [00:00<00:00, 9.15it/s]
100%|###################################################################################################################################| Fold : 10/10 [00:01<00:00, 9.12it/s]
100%|###################################################################################################################################| Fold : 10/10 [00:01<00:00, 9.11it/s]
50%|################################################################5 | n_interp : 2/4 [00:02<00:02, 1.24s/it]
0%| | Repairing epochs : 0/20 [00:00<?, ?it/s]
25%|############################## | Repairing epochs : 5/20 [00:00<00:00, 195.54it/s]
40%|################################################ | Repairing epochs : 8/20 [00:00<00:00, 185.32it/s]
60%|#######################################################################3 | Repairing epochs : 12/20 [00:00<00:00, 178.66it/s]
75%|#########################################################################################2 | Repairing epochs : 15/20 [00:00<00:00, 164.32it/s]
85%|#####################################################################################################1 | Repairing epochs : 17/20 [00:00<00:00, 155.73it/s]
95%|################################################################################################################# | Repairing epochs : 19/20 [00:00<00:00, 146.63it/s]
100%|#######################################################################################################################| Repairing epochs : 20/20 [00:00<00:00, 145.90it/s]
0%| | Fold : 0/10 [00:00<?, ?it/s]
10%|#############2 | Fold : 1/10 [00:00<00:00, 9.28it/s]
20%|##########################4 | Fold : 2/10 [00:00<00:00, 9.18it/s]
30%|#######################################6 | Fold : 3/10 [00:00<00:00, 9.25it/s]
40%|####################################################8 | Fold : 4/10 [00:00<00:00, 9.30it/s]
50%|################################################################## | Fold : 5/10 [00:00<00:00, 9.34it/s]
60%|###############################################################################2 | Fold : 6/10 [00:00<00:00, 9.36it/s]
70%|############################################################################################3 | Fold : 7/10 [00:00<00:00, 9.38it/s]
80%|#########################################################################################################6 | Fold : 8/10 [00:00<00:00, 9.37it/s]
90%|######################################################################################################################8 | Fold : 9/10 [00:00<00:00, 9.31it/s]
100%|###################################################################################################################################| Fold : 10/10 [00:01<00:00, 9.30it/s]
100%|###################################################################################################################################| Fold : 10/10 [00:01<00:00, 9.30it/s]
75%|################################################################################################7 | n_interp : 3/4 [00:03<00:01, 1.24s/it]
0%| | Repairing epochs : 0/20 [00:00<?, ?it/s]
25%|############################## | Repairing epochs : 5/20 [00:00<00:00, 256.99it/s]
45%|###################################################### | Repairing epochs : 9/20 [00:00<00:00, 253.03it/s]
60%|#######################################################################3 | Repairing epochs : 12/20 [00:00<00:00, 206.07it/s]
75%|#########################################################################################2 | Repairing epochs : 15/20 [00:00<00:00, 184.03it/s]
85%|#####################################################################################################1 | Repairing epochs : 17/20 [00:00<00:00, 169.12it/s]
95%|################################################################################################################# | Repairing epochs : 19/20 [00:00<00:00, 154.41it/s]
100%|#######################################################################################################################| Repairing epochs : 20/20 [00:00<00:00, 154.74it/s]
0%| | Fold : 0/10 [00:00<?, ?it/s]
10%|#############2 | Fold : 1/10 [00:00<00:00, 10.11it/s]
20%|##########################4 | Fold : 2/10 [00:00<00:00, 10.08it/s]
30%|#######################################6 | Fold : 3/10 [00:00<00:00, 10.01it/s]
40%|####################################################8 | Fold : 4/10 [00:00<00:00, 10.01it/s]
50%|################################################################## | Fold : 5/10 [00:00<00:00, 9.99it/s]
60%|###############################################################################2 | Fold : 6/10 [00:00<00:00, 10.01it/s]
70%|############################################################################################3 | Fold : 7/10 [00:00<00:00, 10.05it/s]
80%|#########################################################################################################6 | Fold : 8/10 [00:00<00:00, 9.85it/s]
90%|######################################################################################################################8 | Fold : 9/10 [00:00<00:00, 9.65it/s]
100%|###################################################################################################################################| Fold : 10/10 [00:01<00:00, 9.69it/s]
100%|###################################################################################################################################| Fold : 10/10 [00:01<00:00, 9.74it/s]
100%|#################################################################################################################################| n_interp : 4/4 [00:04<00:00, 1.22s/it]
100%|#################################################################################################################################| n_interp : 4/4 [00:04<00:00, 1.22s/it]
Estimated consensus=0.40 and n_interpolate=2
0%| | Repairing epochs : 0/97 [00:00<?, ?it/s]
5%|######1 | Repairing epochs : 5/97 [00:00<00:00, 250.73it/s]
9%|###########1 | Repairing epochs : 9/97 [00:00<00:00, 243.51it/s]
12%|##############7 | Repairing epochs : 12/97 [00:00<00:00, 205.11it/s]
15%|##################4 | Repairing epochs : 15/97 [00:00<00:00, 183.21it/s]
18%|####################8 | Repairing epochs : 17/97 [00:00<00:00, 169.53it/s]
20%|#######################3 | Repairing epochs : 19/97 [00:00<00:00, 160.80it/s]
22%|#########################7 | Repairing epochs : 21/97 [00:00<00:00, 150.14it/s]
24%|############################2 | Repairing epochs : 23/97 [00:00<00:00, 142.61it/s]
26%|##############################6 | Repairing epochs : 25/97 [00:00<00:00, 139.34it/s]
28%|#################################1 | Repairing epochs : 27/97 [00:00<00:00, 135.28it/s]
30%|###################################5 | Repairing epochs : 29/97 [00:00<00:00, 131.07it/s]
32%|###################################### | Repairing epochs : 31/97 [00:00<00:00, 128.27it/s]
34%|########################################4 | Repairing epochs : 33/97 [00:00<00:00, 125.21it/s]
36%|##########################################9 | Repairing epochs : 35/97 [00:00<00:00, 122.03it/s]
38%|#############################################3 | Repairing epochs : 37/97 [00:00<00:00, 119.90it/s]
40%|###############################################8 | Repairing epochs : 39/97 [00:00<00:00, 119.15it/s]
43%|###################################################5 | Repairing epochs : 42/97 [00:00<00:00, 120.53it/s]
45%|#####################################################9 | Repairing epochs : 44/97 [00:00<00:00, 119.80it/s]
47%|########################################################4 | Repairing epochs : 46/97 [00:00<00:00, 118.90it/s]
49%|##########################################################8 | Repairing epochs : 48/97 [00:00<00:00, 118.32it/s]
53%|##############################################################5 | Repairing epochs : 51/97 [00:00<00:00, 120.31it/s]
55%|################################################################# | Repairing epochs : 53/97 [00:00<00:00, 119.67it/s]
57%|###################################################################4 | Repairing epochs : 55/97 [00:00<00:00, 118.55it/s]
60%|#######################################################################1 | Repairing epochs : 58/97 [00:00<00:00, 120.83it/s]
63%|##########################################################################8 | Repairing epochs : 61/97 [00:00<00:00, 121.52it/s]
66%|##############################################################################5 | Repairing epochs : 64/97 [00:00<00:00, 123.69it/s]
68%|################################################################################9 | Repairing epochs : 66/97 [00:00<00:00, 122.15it/s]
70%|###################################################################################4 | Repairing epochs : 68/97 [00:00<00:00, 121.02it/s]
72%|#####################################################################################8 | Repairing epochs : 70/97 [00:00<00:00, 120.01it/s]
74%|########################################################################################3 | Repairing epochs : 72/97 [00:00<00:00, 119.56it/s]
76%|##########################################################################################7 | Repairing epochs : 74/97 [00:00<00:00, 119.24it/s]
78%|#############################################################################################2 | Repairing epochs : 76/97 [00:00<00:00, 118.75it/s]
80%|###############################################################################################6 | Repairing epochs : 78/97 [00:00<00:00, 118.31it/s]
82%|##################################################################################################1 | Repairing epochs : 80/97 [00:00<00:00, 117.33it/s]
85%|####################################################################################################5 | Repairing epochs : 82/97 [00:00<00:00, 116.60it/s]
87%|####################################################################################################### | Repairing epochs : 84/97 [00:00<00:00, 115.82it/s]
89%|#########################################################################################################5 | Repairing epochs : 86/97 [00:00<00:00, 115.19it/s]
91%|###########################################################################################################9 | Repairing epochs : 88/97 [00:00<00:00, 112.55it/s]
93%|##############################################################################################################4 | Repairing epochs : 90/97 [00:00<00:00, 111.38it/s]
95%|################################################################################################################8 | Repairing epochs : 92/97 [00:00<00:00, 109.74it/s]
97%|###################################################################################################################3 | Repairing epochs : 94/97 [00:00<00:00, 109.12it/s]
99%|#####################################################################################################################7 | Repairing epochs : 96/97 [00:00<00:00, 107.66it/s]
100%|#######################################################################################################################| Repairing epochs : 97/97 [00:00<00:00, 116.03it/s]
Dropped 11 epochs: 62, 63, 64, 65, 69, 71, 72, 73, 80, 95, 96
<MNEBrowseFigure size 800x800 with 4 Axes>
We will do a few more visualizations to see that removing the bad epochs
found by autoreject
is still important even with preprocessing first.
This is especially important if your analyses include trial-level statistics
such as looking for bursting activity. We’ll visualize why autoreject
excluded these epochs and the effect that including these bad epochs would
have on the data.
First, we will visualize the reject log
reject_log.plot('horizontal')
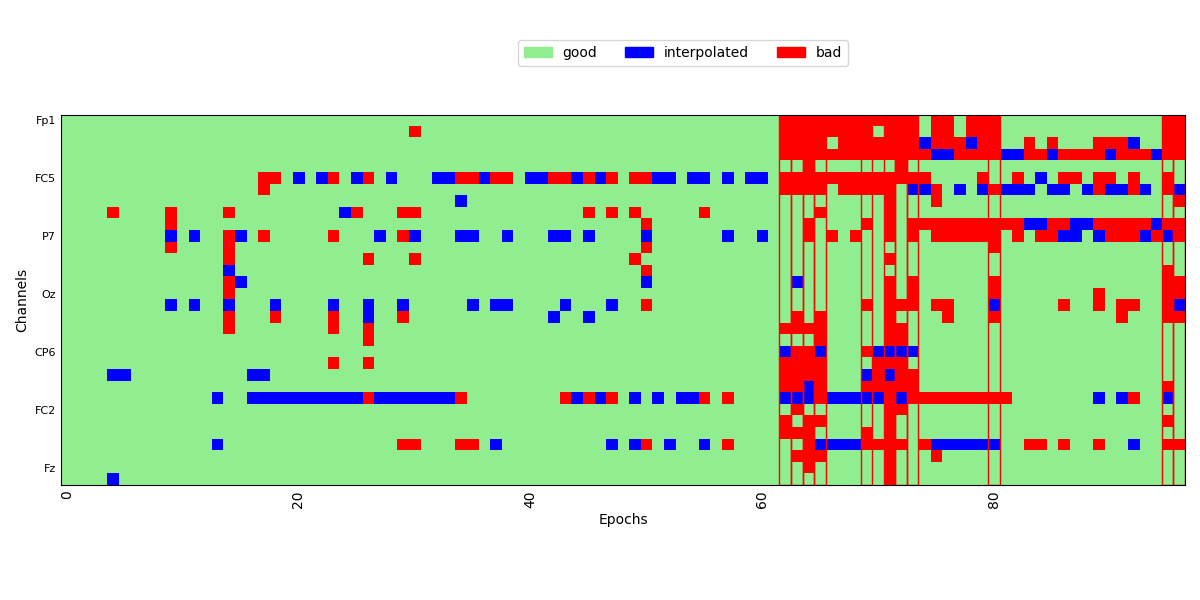
<Figure size 1200x600 with 1 Axes>
Next, we will visualize the cleaned average data and compare it against the bad segments.
evoked_bad = epochs[reject_log.bad_epochs].average()
plt.figure()
plt.plot(evoked_bad.times, evoked_bad.data.T * 1e6, 'r', zorder=-1)
epochs_ar.average().plot(axes=plt.gca())
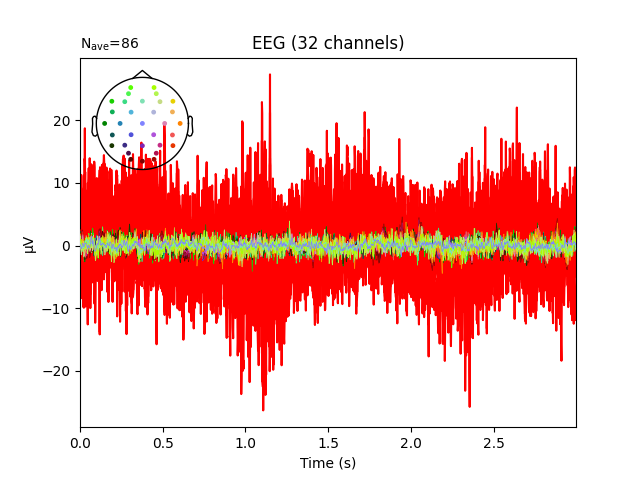
NOTE: pick_channels() is a legacy function. New code should use inst.pick(...).
NOTE: pick_channels() is a legacy function. New code should use inst.pick(...).
<Figure size 640x480 with 2 Axes>
As a last optional step, we can do inspect the reject_log and make manual corrections to the reject_log. For instance, if data is limited, we may not want to drop epochs but retain the list of bad epochs for quality assurance metrics.
reject_log = ar.get_reject_log(epochs)
bad_epochs = reject_log.bad_epochs.copy()
reject_log.bad_epochs[:] = False # no bad epochs
The modified reject log can be applied to the data as follows.
epochs_ar = ar.transform(epochs, reject_log=reject_log)
print(f'Number of epochs originally: {len(epochs)}, '
f'after autoreject: {len(epochs_ar)}')
0%| | Repairing epochs : 0/97 [00:00<?, ?it/s]
5%|######1 | Repairing epochs : 5/97 [00:00<00:00, 262.94it/s]
9%|###########1 | Repairing epochs : 9/97 [00:00<00:00, 249.23it/s]
12%|##############7 | Repairing epochs : 12/97 [00:00<00:00, 208.05it/s]
15%|##################4 | Repairing epochs : 15/97 [00:00<00:00, 189.03it/s]
18%|####################8 | Repairing epochs : 17/97 [00:00<00:00, 174.84it/s]
20%|#######################3 | Repairing epochs : 19/97 [00:00<00:00, 161.70it/s]
22%|#########################7 | Repairing epochs : 21/97 [00:00<00:00, 152.32it/s]
24%|############################2 | Repairing epochs : 23/97 [00:00<00:00, 144.20it/s]
26%|##############################6 | Repairing epochs : 25/97 [00:00<00:00, 138.94it/s]
28%|#################################1 | Repairing epochs : 27/97 [00:00<00:00, 134.72it/s]
30%|###################################5 | Repairing epochs : 29/97 [00:00<00:00, 129.87it/s]
32%|###################################### | Repairing epochs : 31/97 [00:00<00:00, 123.72it/s]
34%|########################################4 | Repairing epochs : 33/97 [00:00<00:00, 118.48it/s]
36%|##########################################9 | Repairing epochs : 35/97 [00:00<00:00, 113.48it/s]
38%|#############################################3 | Repairing epochs : 37/97 [00:00<00:00, 109.77it/s]
40%|###############################################8 | Repairing epochs : 39/97 [00:00<00:00, 109.15it/s]
43%|###################################################5 | Repairing epochs : 42/97 [00:00<00:00, 110.66it/s]
45%|#####################################################9 | Repairing epochs : 44/97 [00:00<00:00, 110.68it/s]
47%|########################################################4 | Repairing epochs : 46/97 [00:00<00:00, 110.70it/s]
49%|##########################################################8 | Repairing epochs : 48/97 [00:00<00:00, 110.93it/s]
53%|##############################################################5 | Repairing epochs : 51/97 [00:00<00:00, 113.58it/s]
55%|################################################################# | Repairing epochs : 53/97 [00:00<00:00, 112.98it/s]
57%|###################################################################4 | Repairing epochs : 55/97 [00:00<00:00, 112.45it/s]
60%|#######################################################################1 | Repairing epochs : 58/97 [00:00<00:00, 114.65it/s]
63%|##########################################################################8 | Repairing epochs : 61/97 [00:00<00:00, 115.86it/s]
66%|##############################################################################5 | Repairing epochs : 64/97 [00:00<00:00, 117.67it/s]
68%|################################################################################9 | Repairing epochs : 66/97 [00:00<00:00, 117.26it/s]
70%|###################################################################################4 | Repairing epochs : 68/97 [00:00<00:00, 116.71it/s]
72%|#####################################################################################8 | Repairing epochs : 70/97 [00:00<00:00, 116.01it/s]
74%|########################################################################################3 | Repairing epochs : 72/97 [00:00<00:00, 115.72it/s]
76%|##########################################################################################7 | Repairing epochs : 74/97 [00:00<00:00, 115.63it/s]
78%|#############################################################################################2 | Repairing epochs : 76/97 [00:00<00:00, 115.27it/s]
80%|###############################################################################################6 | Repairing epochs : 78/97 [00:00<00:00, 114.74it/s]
82%|##################################################################################################1 | Repairing epochs : 80/97 [00:00<00:00, 113.99it/s]
85%|####################################################################################################5 | Repairing epochs : 82/97 [00:00<00:00, 113.48it/s]
87%|####################################################################################################### | Repairing epochs : 84/97 [00:00<00:00, 112.31it/s]
89%|#########################################################################################################5 | Repairing epochs : 86/97 [00:00<00:00, 110.64it/s]
91%|###########################################################################################################9 | Repairing epochs : 88/97 [00:00<00:00, 110.33it/s]
93%|##############################################################################################################4 | Repairing epochs : 90/97 [00:00<00:00, 109.88it/s]
95%|################################################################################################################8 | Repairing epochs : 92/97 [00:00<00:00, 108.67it/s]
97%|###################################################################################################################3 | Repairing epochs : 94/97 [00:00<00:00, 108.29it/s]
99%|#####################################################################################################################7 | Repairing epochs : 96/97 [00:00<00:00, 106.59it/s]
100%|#######################################################################################################################| Repairing epochs : 97/97 [00:00<00:00, 113.00it/s]
No bad epochs were found for your data. Returning a copy of the data you wanted to clean. Interpolation may have been done.
Number of epochs originally: 97, after autoreject: 97
Finally, don’t forget that we are working with resting state data here. Here we used long epochs of 3 seconds so that frequency-domain analysis was possible with the epochs. However, this could also lead to longer segments of the data being rejected. If you want more fine-grained control over the artifacts, you can construct shorter epochs and use the autoreject log to mark annotations in MNE that can be used to reject the data during doing time-frequency analysis. We want to emphasize that there is no subsitute for visual inspection. Irrespective of the rejection method used, we highly recommend users to inspect their preprocessed data before further analyses.
Total running time of the script: ( 1 minutes 29.369 seconds)