Note
Go to the end to download the full example code
Automatically repair epochs#
This example demonstrates how to use autoreject
to automatically
repair epochs.
# Author: Mainak Jas <mainak.jas@telecom-paristech.fr>
# Denis A. Engemann <denis.engemann@gmail.com>
# License: BSD-3-Clause
Let us first define the parameters. n_interpolates are the \(\rho\)
values that we would like autoreject
to try and consensus_percs
are the \(\kappa\) values that autoreject
will try (see the
autoreject paper) for
more information on these parameters).
Epochs with more than \(\kappa * N\) sensors (\(N\) total sensors) bad are dropped. For the rest of the epochs, the worst \(\rho\) bad sensors (as determined by channel-level thresholds) are interpolated. The exact values of these parameters are not preselected but learned from the data. If the number of bad sensors for a particular trial is less than \(\rho\), all the bad sensors are interpolated.
import numpy as np
n_interpolates = np.array([1, 4, 32])
consensus_percs = np.linspace(0, 1.0, 11)
For the purposes of this example, we shall use the MNE sample dataset. Therefore, let us make some MNE related imports.
import mne # noqa
from mne.utils import check_random_state # noqa
from mne.datasets import sample # noqa
Now, we can import the class required for rejecting and repairing bad
epochs. autoreject.compute_thresholds()
is a callable which must be
provided to the autoreject.AutoReject
class for computing
the channel-level thresholds.
from autoreject import (AutoReject, set_matplotlib_defaults) # noqa
Let us now read in the raw fif file for MNE sample dataset.
check_random_state(42)
data_path = sample.data_path()
meg_path = data_path / 'MEG' / 'sample'
raw_fname = meg_path / 'sample_audvis_filt-0-40_raw.fif'
raw = mne.io.read_raw_fif(raw_fname, preload=True)
Opening raw data file C:\Users\stefan\mne_data\MNE-sample-data\MEG\sample\sample_audvis_filt-0-40_raw.fif...
Read a total of 4 projection items:
PCA-v1 (1 x 102) idle
PCA-v2 (1 x 102) idle
PCA-v3 (1 x 102) idle
Average EEG reference (1 x 60) idle
Range : 6450 ... 48149 = 42.956 ... 320.665 secs
Ready.
Reading 0 ... 41699 = 0.000 ... 277.709 secs...
We can then read in the events
event_fname = meg_path / 'sample_audvis_filt-0-40_raw-eve.fif'
event_id = {'Auditory/Left': 1, 'Auditory/Right': 2}
tmin, tmax = -0.2, 0.5
events = mne.read_events(event_fname)
And pick MEG channels for repairing. Currently, autoreject
can repair
only one channel type at a time.
raw.info['bads'] = []
picks = mne.pick_types(raw.info, meg='grad', eeg=False, stim=False, eog=False,
include=[], exclude=[])
Now, we can create epochs. The reject
params will be set to None
because we do not want epochs to be dropped when instantiating
mne.Epochs
.
raw.del_proj() # remove proj, don't proj while interpolating
epochs = mne.Epochs(raw, events, event_id, tmin, tmax,
baseline=(None, 0), reject=None,
verbose=False, detrend=0, preload=True)
autoreject.AutoReject
internally does cross-validation to
determine the optimal values \(\rho^{*}\) and \(\kappa^{*}\)
Note that autoreject.AutoReject
by design supports
multiple channels.
If no picks are passed, separate solutions will be computed for each channel
type and internally combined. This then readily supports cleaning
unseen epochs from the different channel types used during fit.
Here we only use a subset of channels to save time.
ar = AutoReject(n_interpolates, consensus_percs, picks=picks,
thresh_method='random_search', random_state=42)
# Note that fitting and transforming can be done on different compatible
# portions of data if needed.
ar.fit(epochs['Auditory/Left'])
epochs_clean = ar.transform(epochs['Auditory/Left'])
evoked_clean = epochs_clean.average()
evoked = epochs['Auditory/Left'].average()
Running autoreject on ch_type=grad
0%| | Creating augmented epochs : 0/204 [00:00<?, ?it/s]
0%|5 | Creating augmented epochs : 1/204 [00:00<00:28, 7.07it/s]
1%|# | Creating augmented epochs : 2/204 [00:00<00:26, 7.51it/s]
1%|#6 | Creating augmented epochs : 3/204 [00:00<00:26, 7.66it/s]
2%|##1 | Creating augmented epochs : 4/204 [00:00<00:25, 7.75it/s]
2%|##7 | Creating augmented epochs : 5/204 [00:00<00:25, 7.79it/s]
3%|###2 | Creating augmented epochs : 6/204 [00:00<00:25, 7.83it/s]
3%|###8 | Creating augmented epochs : 7/204 [00:00<00:24, 8.02it/s]
4%|####3 | Creating augmented epochs : 8/204 [00:01<00:24, 8.01it/s]
4%|####9 | Creating augmented epochs : 9/204 [00:01<00:24, 7.87it/s]
5%|#####4 | Creating augmented epochs : 10/204 [00:01<00:24, 7.88it/s]
5%|#####9 | Creating augmented epochs : 11/204 [00:01<00:24, 8.01it/s]
6%|######5 | Creating augmented epochs : 12/204 [00:01<00:23, 8.00it/s]
6%|####### | Creating augmented epochs : 13/204 [00:01<00:23, 8.00it/s]
7%|#######6 | Creating augmented epochs : 14/204 [00:01<00:23, 8.09it/s]
7%|########1 | Creating augmented epochs : 15/204 [00:01<00:23, 8.08it/s]
8%|########7 | Creating augmented epochs : 16/204 [00:01<00:23, 8.07it/s]
8%|#########2 | Creating augmented epochs : 17/204 [00:02<00:23, 8.06it/s]
9%|#########7 | Creating augmented epochs : 18/204 [00:02<00:23, 8.05it/s]
9%|##########3 | Creating augmented epochs : 19/204 [00:02<00:22, 8.05it/s]
10%|##########8 | Creating augmented epochs : 20/204 [00:02<00:22, 8.04it/s]
10%|###########4 | Creating augmented epochs : 21/204 [00:02<00:22, 8.04it/s]
11%|###########9 | Creating augmented epochs : 22/204 [00:02<00:22, 8.11it/s]
11%|############5 | Creating augmented epochs : 23/204 [00:02<00:22, 8.10it/s]
12%|############# | Creating augmented epochs : 24/204 [00:02<00:22, 8.09it/s]
12%|#############6 | Creating augmented epochs : 25/204 [00:03<00:22, 8.08it/s]
13%|##############1 | Creating augmented epochs : 26/204 [00:03<00:22, 8.07it/s]
13%|##############6 | Creating augmented epochs : 27/204 [00:03<00:21, 8.06it/s]
14%|###############2 | Creating augmented epochs : 28/204 [00:03<00:21, 8.06it/s]
14%|###############7 | Creating augmented epochs : 29/204 [00:03<00:21, 8.05it/s]
15%|################3 | Creating augmented epochs : 30/204 [00:03<00:21, 8.05it/s]
15%|################8 | Creating augmented epochs : 31/204 [00:03<00:21, 8.10it/s]
16%|#################4 | Creating augmented epochs : 32/204 [00:03<00:21, 8.10it/s]
16%|#################9 | Creating augmented epochs : 33/204 [00:04<00:21, 8.09it/s]
17%|##################5 | Creating augmented epochs : 34/204 [00:04<00:20, 8.15it/s]
17%|################### | Creating augmented epochs : 35/204 [00:04<00:20, 8.07it/s]
18%|###################5 | Creating augmented epochs : 36/204 [00:04<00:20, 8.13it/s]
18%|####################1 | Creating augmented epochs : 37/204 [00:04<00:20, 8.12it/s]
19%|####################6 | Creating augmented epochs : 38/204 [00:04<00:20, 8.11it/s]
19%|#####################2 | Creating augmented epochs : 39/204 [00:04<00:20, 8.10it/s]
20%|#####################7 | Creating augmented epochs : 40/204 [00:04<00:20, 8.09it/s]
20%|######################3 | Creating augmented epochs : 41/204 [00:05<00:20, 8.15it/s]
21%|######################8 | Creating augmented epochs : 42/204 [00:05<00:19, 8.14it/s]
21%|#######################3 | Creating augmented epochs : 43/204 [00:05<00:19, 8.07it/s]
22%|#######################9 | Creating augmented epochs : 44/204 [00:05<00:19, 8.06it/s]
22%|########################4 | Creating augmented epochs : 45/204 [00:05<00:19, 8.11it/s]
23%|######################### | Creating augmented epochs : 46/204 [00:05<00:19, 8.11it/s]
23%|#########################5 | Creating augmented epochs : 47/204 [00:05<00:19, 8.16it/s]
24%|##########################1 | Creating augmented epochs : 48/204 [00:05<00:19, 8.15it/s]
24%|##########################6 | Creating augmented epochs : 49/204 [00:06<00:18, 8.16it/s]
25%|###########################2 | Creating augmented epochs : 50/204 [00:06<00:18, 8.18it/s]
25%|###########################7 | Creating augmented epochs : 51/204 [00:06<00:18, 8.17it/s]
25%|############################2 | Creating augmented epochs : 52/204 [00:06<00:18, 8.10it/s]
26%|############################8 | Creating augmented epochs : 53/204 [00:06<00:18, 8.12it/s]
26%|#############################3 | Creating augmented epochs : 54/204 [00:06<00:18, 8.14it/s]
27%|#############################9 | Creating augmented epochs : 55/204 [00:06<00:18, 8.19it/s]
27%|##############################4 | Creating augmented epochs : 56/204 [00:06<00:18, 8.18it/s]
28%|############################### | Creating augmented epochs : 57/204 [00:07<00:17, 8.22it/s]
28%|###############################5 | Creating augmented epochs : 58/204 [00:07<00:17, 8.21it/s]
29%|################################1 | Creating augmented epochs : 59/204 [00:07<00:17, 8.24it/s]
29%|################################6 | Creating augmented epochs : 60/204 [00:07<00:17, 8.24it/s]
30%|#################################1 | Creating augmented epochs : 61/204 [00:07<00:17, 8.17it/s]
30%|#################################7 | Creating augmented epochs : 62/204 [00:07<00:17, 8.16it/s]
31%|##################################2 | Creating augmented epochs : 63/204 [00:07<00:17, 8.19it/s]
31%|##################################8 | Creating augmented epochs : 64/204 [00:07<00:17, 8.19it/s]
32%|###################################3 | Creating augmented epochs : 65/204 [00:07<00:16, 8.23it/s]
32%|###################################9 | Creating augmented epochs : 66/204 [00:08<00:16, 8.22it/s]
33%|####################################4 | Creating augmented epochs : 67/204 [00:08<00:16, 8.26it/s]
33%|##################################### | Creating augmented epochs : 68/204 [00:08<00:16, 8.25it/s]
34%|#####################################5 | Creating augmented epochs : 69/204 [00:08<00:16, 8.28it/s]
34%|###################################### | Creating augmented epochs : 70/204 [00:08<00:16, 8.27it/s]
35%|######################################6 | Creating augmented epochs : 71/204 [00:08<00:16, 8.25it/s]
35%|#######################################1 | Creating augmented epochs : 72/204 [00:08<00:16, 8.24it/s]
36%|#######################################7 | Creating augmented epochs : 73/204 [00:08<00:15, 8.28it/s]
36%|########################################2 | Creating augmented epochs : 74/204 [00:09<00:15, 8.26it/s]
37%|########################################8 | Creating augmented epochs : 75/204 [00:09<00:15, 8.30it/s]
37%|#########################################3 | Creating augmented epochs : 76/204 [00:09<00:15, 8.28it/s]
38%|#########################################8 | Creating augmented epochs : 77/204 [00:09<00:15, 8.27it/s]
38%|##########################################4 | Creating augmented epochs : 78/204 [00:09<00:15, 8.31it/s]
39%|##########################################9 | Creating augmented epochs : 79/204 [00:09<00:15, 8.29it/s]
39%|###########################################5 | Creating augmented epochs : 80/204 [00:09<00:14, 8.33it/s]
40%|############################################ | Creating augmented epochs : 81/204 [00:09<00:14, 8.31it/s]
40%|############################################6 | Creating augmented epochs : 82/204 [00:10<00:14, 8.35it/s]
41%|#############################################1 | Creating augmented epochs : 83/204 [00:10<00:14, 8.33it/s]
41%|#############################################7 | Creating augmented epochs : 84/204 [00:10<00:14, 8.36it/s]
42%|##############################################2 | Creating augmented epochs : 85/204 [00:10<00:14, 8.34it/s]
42%|##############################################7 | Creating augmented epochs : 86/204 [00:10<00:14, 8.32it/s]
43%|###############################################3 | Creating augmented epochs : 87/204 [00:10<00:13, 8.36it/s]
43%|###############################################8 | Creating augmented epochs : 88/204 [00:10<00:13, 8.34it/s]
44%|################################################4 | Creating augmented epochs : 89/204 [00:10<00:13, 8.37it/s]
44%|################################################9 | Creating augmented epochs : 90/204 [00:10<00:13, 8.35it/s]
45%|#################################################5 | Creating augmented epochs : 91/204 [00:11<00:13, 8.39it/s]
45%|################################################## | Creating augmented epochs : 92/204 [00:11<00:13, 8.36it/s]
46%|##################################################6 | Creating augmented epochs : 93/204 [00:11<00:13, 8.40it/s]
46%|###################################################1 | Creating augmented epochs : 94/204 [00:11<00:13, 8.38it/s]
47%|###################################################6 | Creating augmented epochs : 95/204 [00:11<00:13, 8.36it/s]
47%|####################################################2 | Creating augmented epochs : 96/204 [00:11<00:12, 8.39it/s]
48%|####################################################7 | Creating augmented epochs : 97/204 [00:11<00:12, 8.42it/s]
48%|#####################################################3 | Creating augmented epochs : 98/204 [00:11<00:12, 8.40it/s]
49%|#####################################################8 | Creating augmented epochs : 99/204 [00:12<00:12, 8.43it/s]
49%|#####################################################9 | Creating augmented epochs : 100/204 [00:12<00:12, 8.46it/s]
50%|######################################################4 | Creating augmented epochs : 101/204 [00:12<00:12, 8.44it/s]
50%|####################################################### | Creating augmented epochs : 102/204 [00:12<00:12, 8.41it/s]
50%|#######################################################5 | Creating augmented epochs : 103/204 [00:12<00:11, 8.45it/s]
51%|######################################################## | Creating augmented epochs : 104/204 [00:12<00:11, 8.36it/s]
51%|########################################################6 | Creating augmented epochs : 105/204 [00:12<00:11, 8.31it/s]
52%|#########################################################1 | Creating augmented epochs : 106/204 [00:12<00:11, 8.33it/s]
52%|#########################################################6 | Creating augmented epochs : 107/204 [00:13<00:11, 8.31it/s]
53%|##########################################################2 | Creating augmented epochs : 108/204 [00:13<00:11, 8.34it/s]
53%|##########################################################7 | Creating augmented epochs : 109/204 [00:13<00:11, 8.32it/s]
54%|###########################################################3 | Creating augmented epochs : 110/204 [00:13<00:11, 8.36it/s]
54%|###########################################################8 | Creating augmented epochs : 111/204 [00:13<00:11, 8.37it/s]
55%|############################################################3 | Creating augmented epochs : 112/204 [00:13<00:10, 8.37it/s]
55%|############################################################9 | Creating augmented epochs : 113/204 [00:13<00:10, 8.41it/s]
56%|#############################################################4 | Creating augmented epochs : 114/204 [00:13<00:10, 8.33it/s]
56%|############################################################## | Creating augmented epochs : 115/204 [00:13<00:10, 8.31it/s]
57%|##############################################################5 | Creating augmented epochs : 116/204 [00:14<00:10, 8.35it/s]
57%|############################################################### | Creating augmented epochs : 117/204 [00:14<00:10, 8.33it/s]
58%|###############################################################6 | Creating augmented epochs : 118/204 [00:14<00:10, 8.31it/s]
58%|################################################################1 | Creating augmented epochs : 119/204 [00:14<00:10, 8.35it/s]
59%|################################################################7 | Creating augmented epochs : 120/204 [00:14<00:10, 8.33it/s]
59%|#################################################################2 | Creating augmented epochs : 121/204 [00:14<00:09, 8.36it/s]
60%|#################################################################7 | Creating augmented epochs : 122/204 [00:14<00:09, 8.40it/s]
60%|##################################################################3 | Creating augmented epochs : 123/204 [00:14<00:09, 8.32it/s]
61%|##################################################################8 | Creating augmented epochs : 124/204 [00:15<00:09, 8.30it/s]
61%|###################################################################4 | Creating augmented epochs : 125/204 [00:15<00:09, 8.28it/s]
62%|###################################################################9 | Creating augmented epochs : 126/204 [00:15<00:09, 8.32it/s]
62%|####################################################################4 | Creating augmented epochs : 127/204 [00:15<00:09, 8.30it/s]
63%|##################################################################### | Creating augmented epochs : 128/204 [00:15<00:09, 8.34it/s]
63%|#####################################################################5 | Creating augmented epochs : 129/204 [00:15<00:09, 8.32it/s]
64%|###################################################################### | Creating augmented epochs : 130/204 [00:15<00:08, 8.36it/s]
64%|######################################################################6 | Creating augmented epochs : 131/204 [00:15<00:08, 8.34it/s]
65%|#######################################################################1 | Creating augmented epochs : 132/204 [00:15<00:08, 8.32it/s]
65%|#######################################################################7 | Creating augmented epochs : 133/204 [00:16<00:08, 8.35it/s]
66%|########################################################################2 | Creating augmented epochs : 134/204 [00:16<00:08, 8.33it/s]
66%|########################################################################7 | Creating augmented epochs : 135/204 [00:16<00:08, 8.37it/s]
67%|#########################################################################3 | Creating augmented epochs : 136/204 [00:16<00:08, 8.35it/s]
67%|#########################################################################8 | Creating augmented epochs : 137/204 [00:16<00:08, 8.27it/s]
68%|##########################################################################4 | Creating augmented epochs : 138/204 [00:16<00:07, 8.31it/s]
68%|##########################################################################9 | Creating augmented epochs : 139/204 [00:16<00:07, 8.35it/s]
69%|###########################################################################4 | Creating augmented epochs : 140/204 [00:16<00:07, 8.33it/s]
69%|############################################################################ | Creating augmented epochs : 141/204 [00:17<00:07, 8.31it/s]
70%|############################################################################5 | Creating augmented epochs : 142/204 [00:17<00:07, 8.35it/s]
70%|#############################################################################1 | Creating augmented epochs : 143/204 [00:17<00:07, 8.33it/s]
71%|#############################################################################6 | Creating augmented epochs : 144/204 [00:17<00:07, 8.36it/s]
71%|##############################################################################1 | Creating augmented epochs : 145/204 [00:17<00:07, 8.40it/s]
72%|##############################################################################7 | Creating augmented epochs : 146/204 [00:17<00:06, 8.43it/s]
72%|###############################################################################2 | Creating augmented epochs : 147/204 [00:17<00:06, 8.40it/s]
73%|###############################################################################8 | Creating augmented epochs : 148/204 [00:17<00:06, 8.44it/s]
73%|################################################################################3 | Creating augmented epochs : 149/204 [00:18<00:06, 8.41it/s]
74%|################################################################################8 | Creating augmented epochs : 150/204 [00:18<00:06, 8.39it/s]
74%|#################################################################################4 | Creating augmented epochs : 151/204 [00:18<00:06, 8.37it/s]
75%|#################################################################################9 | Creating augmented epochs : 152/204 [00:18<00:06, 8.38it/s]
75%|##################################################################################5 | Creating augmented epochs : 153/204 [00:18<00:06, 8.44it/s]
75%|################################################################################### | Creating augmented epochs : 154/204 [00:18<00:05, 8.41it/s]
76%|###################################################################################5 | Creating augmented epochs : 155/204 [00:18<00:05, 8.39it/s]
76%|####################################################################################1 | Creating augmented epochs : 156/204 [00:18<00:05, 8.37it/s]
77%|####################################################################################6 | Creating augmented epochs : 157/204 [00:18<00:05, 8.40it/s]
77%|#####################################################################################1 | Creating augmented epochs : 158/204 [00:19<00:05, 8.38it/s]
78%|#####################################################################################7 | Creating augmented epochs : 159/204 [00:19<00:05, 8.41it/s]
78%|######################################################################################2 | Creating augmented epochs : 160/204 [00:19<00:05, 8.39it/s]
79%|######################################################################################8 | Creating augmented epochs : 161/204 [00:19<00:05, 8.42it/s]
79%|#######################################################################################3 | Creating augmented epochs : 162/204 [00:19<00:05, 8.40it/s]
80%|#######################################################################################8 | Creating augmented epochs : 163/204 [00:19<00:04, 8.38it/s]
80%|########################################################################################4 | Creating augmented epochs : 164/204 [00:19<00:04, 8.35it/s]
81%|########################################################################################9 | Creating augmented epochs : 165/204 [00:19<00:04, 8.34it/s]
81%|#########################################################################################5 | Creating augmented epochs : 166/204 [00:20<00:04, 8.32it/s]
82%|########################################################################################## | Creating augmented epochs : 167/204 [00:20<00:04, 8.30it/s]
82%|##########################################################################################5 | Creating augmented epochs : 168/204 [00:20<00:04, 8.33it/s]
83%|###########################################################################################1 | Creating augmented epochs : 169/204 [00:20<00:04, 8.32it/s]
83%|###########################################################################################6 | Creating augmented epochs : 170/204 [00:20<00:04, 8.35it/s]
84%|############################################################################################2 | Creating augmented epochs : 171/204 [00:20<00:03, 8.33it/s]
84%|############################################################################################7 | Creating augmented epochs : 172/204 [00:20<00:03, 8.37it/s]
85%|#############################################################################################2 | Creating augmented epochs : 173/204 [00:20<00:03, 8.39it/s]
85%|#############################################################################################8 | Creating augmented epochs : 174/204 [00:20<00:03, 8.38it/s]
86%|##############################################################################################3 | Creating augmented epochs : 175/204 [00:21<00:03, 8.41it/s]
86%|##############################################################################################9 | Creating augmented epochs : 176/204 [00:21<00:03, 8.45it/s]
87%|###############################################################################################4 | Creating augmented epochs : 177/204 [00:21<00:03, 8.48it/s]
87%|###############################################################################################9 | Creating augmented epochs : 178/204 [00:21<00:03, 8.51it/s]
88%|################################################################################################5 | Creating augmented epochs : 179/204 [00:21<00:02, 8.44it/s]
88%|################################################################################################# | Creating augmented epochs : 180/204 [00:21<00:02, 8.45it/s]
89%|#################################################################################################5 | Creating augmented epochs : 181/204 [00:21<00:02, 8.47it/s]
89%|##################################################################################################1 | Creating augmented epochs : 182/204 [00:21<00:02, 8.48it/s]
90%|##################################################################################################6 | Creating augmented epochs : 183/204 [00:22<00:02, 8.48it/s]
90%|###################################################################################################2 | Creating augmented epochs : 184/204 [00:22<00:02, 8.48it/s]
91%|###################################################################################################7 | Creating augmented epochs : 185/204 [00:22<00:02, 8.49it/s]
91%|####################################################################################################2 | Creating augmented epochs : 186/204 [00:22<00:02, 8.50it/s]
92%|####################################################################################################8 | Creating augmented epochs : 187/204 [00:22<00:01, 8.51it/s]
92%|#####################################################################################################3 | Creating augmented epochs : 188/204 [00:22<00:01, 8.52it/s]
93%|#####################################################################################################9 | Creating augmented epochs : 189/204 [00:22<00:01, 8.52it/s]
93%|######################################################################################################4 | Creating augmented epochs : 190/204 [00:22<00:01, 8.54it/s]
94%|######################################################################################################9 | Creating augmented epochs : 191/204 [00:22<00:01, 8.54it/s]
94%|#######################################################################################################5 | Creating augmented epochs : 192/204 [00:23<00:01, 8.51it/s]
95%|######################################################################################################## | Creating augmented epochs : 193/204 [00:23<00:01, 8.51it/s]
95%|########################################################################################################6 | Creating augmented epochs : 194/204 [00:23<00:01, 8.50it/s]
96%|#########################################################################################################1 | Creating augmented epochs : 195/204 [00:23<00:01, 8.51it/s]
96%|#########################################################################################################6 | Creating augmented epochs : 196/204 [00:23<00:00, 8.54it/s]
97%|##########################################################################################################2 | Creating augmented epochs : 197/204 [00:23<00:00, 8.57it/s]
97%|##########################################################################################################7 | Creating augmented epochs : 198/204 [00:23<00:00, 8.59it/s]
98%|###########################################################################################################3 | Creating augmented epochs : 199/204 [00:23<00:00, 8.61it/s]
98%|###########################################################################################################8 | Creating augmented epochs : 200/204 [00:23<00:00, 8.62it/s]
99%|############################################################################################################3 | Creating augmented epochs : 201/204 [00:24<00:00, 8.58it/s]
99%|############################################################################################################9 | Creating augmented epochs : 202/204 [00:24<00:00, 8.61it/s]
100%|#############################################################################################################4| Creating augmented epochs : 203/204 [00:24<00:00, 8.63it/s]
100%|##############################################################################################################| Creating augmented epochs : 204/204 [00:24<00:00, 8.60it/s]
100%|##############################################################################################################| Creating augmented epochs : 204/204 [00:24<00:00, 8.34it/s]
0%| | Computing thresholds ... : 0/204 [00:00<?, ?it/s]
0%|5 | Computing thresholds ... : 1/204 [00:00<00:22, 9.11it/s]
1%|#1 | Computing thresholds ... : 2/204 [00:00<00:20, 9.83it/s]
1%|#6 | Computing thresholds ... : 3/204 [00:00<00:21, 9.56it/s]
2%|##2 | Computing thresholds ... : 4/204 [00:00<00:20, 9.83it/s]
2%|##7 | Computing thresholds ... : 5/204 [00:00<00:19, 9.99it/s]
3%|###3 | Computing thresholds ... : 6/204 [00:00<00:20, 9.70it/s]
3%|###8 | Computing thresholds ... : 7/204 [00:00<00:20, 9.74it/s]
4%|####4 | Computing thresholds ... : 8/204 [00:00<00:20, 9.78it/s]
4%|####9 | Computing thresholds ... : 9/204 [00:00<00:20, 9.71it/s]
5%|#####4 | Computing thresholds ... : 10/204 [00:01<00:19, 9.82it/s]
5%|###### | Computing thresholds ... : 11/204 [00:01<00:19, 9.85it/s]
6%|######5 | Computing thresholds ... : 12/204 [00:01<00:19, 9.81it/s]
6%|#######1 | Computing thresholds ... : 13/204 [00:01<00:19, 9.88it/s]
7%|#######6 | Computing thresholds ... : 14/204 [00:01<00:19, 9.89it/s]
7%|########2 | Computing thresholds ... : 15/204 [00:01<00:19, 9.88it/s]
8%|########7 | Computing thresholds ... : 16/204 [00:01<00:18, 9.90it/s]
8%|#########3 | Computing thresholds ... : 17/204 [00:01<00:18, 9.86it/s]
9%|#########8 | Computing thresholds ... : 18/204 [00:01<00:18, 9.92it/s]
9%|##########4 | Computing thresholds ... : 19/204 [00:01<00:18, 9.92it/s]
10%|##########9 | Computing thresholds ... : 20/204 [00:02<00:18, 9.90it/s]
10%|###########5 | Computing thresholds ... : 21/204 [00:02<00:18, 9.93it/s]
11%|############ | Computing thresholds ... : 22/204 [00:02<00:18, 9.89it/s]
11%|############6 | Computing thresholds ... : 23/204 [00:02<00:18, 9.94it/s]
12%|#############1 | Computing thresholds ... : 24/204 [00:02<00:18, 9.87it/s]
12%|#############7 | Computing thresholds ... : 25/204 [00:02<00:18, 9.92it/s]
13%|##############2 | Computing thresholds ... : 26/204 [00:02<00:17, 9.96it/s]
13%|##############8 | Computing thresholds ... : 27/204 [00:02<00:17, 9.90it/s]
14%|###############3 | Computing thresholds ... : 28/204 [00:02<00:17, 9.94it/s]
14%|###############9 | Computing thresholds ... : 29/204 [00:02<00:17, 9.97it/s]
15%|################4 | Computing thresholds ... : 30/204 [00:03<00:17, 9.92it/s]
15%|################# | Computing thresholds ... : 31/204 [00:03<00:17, 9.96it/s]
16%|#################5 | Computing thresholds ... : 32/204 [00:03<00:17, 9.97it/s]
16%|##################1 | Computing thresholds ... : 33/204 [00:03<00:17, 9.94it/s]
17%|##################6 | Computing thresholds ... : 34/204 [00:03<00:17, 9.97it/s]
17%|###################2 | Computing thresholds ... : 35/204 [00:03<00:16, 9.97it/s]
18%|###################7 | Computing thresholds ... : 36/204 [00:03<00:16, 9.97it/s]
18%|####################3 | Computing thresholds ... : 37/204 [00:03<00:16, 9.97it/s]
19%|####################8 | Computing thresholds ... : 38/204 [00:03<00:16, 9.97it/s]
19%|#####################4 | Computing thresholds ... : 39/204 [00:03<00:16, 10.06it/s]
20%|#####################9 | Computing thresholds ... : 40/204 [00:04<00:16, 9.97it/s]
20%|######################5 | Computing thresholds ... : 41/204 [00:04<00:16, 9.97it/s]
21%|####################### | Computing thresholds ... : 42/204 [00:04<00:16, 9.97it/s]
21%|#######################6 | Computing thresholds ... : 43/204 [00:04<00:16, 9.97it/s]
22%|########################1 | Computing thresholds ... : 44/204 [00:04<00:15, 10.04it/s]
22%|########################7 | Computing thresholds ... : 45/204 [00:04<00:15, 9.99it/s]
23%|#########################2 | Computing thresholds ... : 46/204 [00:04<00:15, 10.02it/s]
23%|#########################8 | Computing thresholds ... : 47/204 [00:04<00:15, 10.05it/s]
24%|##########################3 | Computing thresholds ... : 48/204 [00:04<00:15, 9.99it/s]
24%|##########################9 | Computing thresholds ... : 49/204 [00:04<00:15, 10.02it/s]
25%|###########################4 | Computing thresholds ... : 50/204 [00:05<00:15, 10.05it/s]
25%|############################ | Computing thresholds ... : 51/204 [00:05<00:15, 10.00it/s]
25%|############################5 | Computing thresholds ... : 52/204 [00:05<00:15, 9.86it/s]
26%|############################# | Computing thresholds ... : 53/204 [00:05<00:15, 9.90it/s]
26%|#############################6 | Computing thresholds ... : 54/204 [00:05<00:15, 9.85it/s]
27%|##############################1 | Computing thresholds ... : 55/204 [00:05<00:15, 9.89it/s]
27%|##############################7 | Computing thresholds ... : 56/204 [00:05<00:14, 9.93it/s]
28%|###############################2 | Computing thresholds ... : 57/204 [00:05<00:14, 9.88it/s]
28%|###############################8 | Computing thresholds ... : 58/204 [00:05<00:14, 9.92it/s]
29%|################################3 | Computing thresholds ... : 59/204 [00:05<00:14, 9.87it/s]
29%|################################9 | Computing thresholds ... : 60/204 [00:06<00:14, 9.91it/s]
30%|#################################4 | Computing thresholds ... : 61/204 [00:06<00:14, 9.94it/s]
30%|################################## | Computing thresholds ... : 62/204 [00:06<00:14, 9.74it/s]
31%|##################################5 | Computing thresholds ... : 63/204 [00:06<00:14, 9.70it/s]
31%|###################################1 | Computing thresholds ... : 64/204 [00:06<00:14, 9.67it/s]
32%|###################################6 | Computing thresholds ... : 65/204 [00:06<00:14, 9.72it/s]
32%|####################################2 | Computing thresholds ... : 66/204 [00:06<00:14, 9.68it/s]
33%|####################################7 | Computing thresholds ... : 67/204 [00:06<00:14, 9.73it/s]
33%|#####################################3 | Computing thresholds ... : 68/204 [00:06<00:14, 9.69it/s]
34%|#####################################8 | Computing thresholds ... : 69/204 [00:07<00:13, 9.74it/s]
34%|######################################4 | Computing thresholds ... : 70/204 [00:07<00:13, 9.78it/s]
35%|######################################9 | Computing thresholds ... : 71/204 [00:07<00:13, 9.74it/s]
35%|#######################################5 | Computing thresholds ... : 72/204 [00:07<00:13, 9.71it/s]
36%|######################################## | Computing thresholds ... : 73/204 [00:07<00:13, 9.68it/s]
36%|########################################6 | Computing thresholds ... : 74/204 [00:07<00:13, 9.72it/s]
37%|#########################################1 | Computing thresholds ... : 75/204 [00:07<00:13, 9.69it/s]
37%|#########################################7 | Computing thresholds ... : 76/204 [00:07<00:13, 9.65it/s]
38%|##########################################2 | Computing thresholds ... : 77/204 [00:07<00:13, 9.53it/s]
38%|##########################################8 | Computing thresholds ... : 78/204 [00:07<00:13, 9.43it/s]
39%|###########################################3 | Computing thresholds ... : 79/204 [00:08<00:13, 9.38it/s]
39%|###########################################9 | Computing thresholds ... : 80/204 [00:08<00:13, 9.35it/s]
40%|############################################4 | Computing thresholds ... : 81/204 [00:08<00:13, 9.37it/s]
40%|############################################# | Computing thresholds ... : 82/204 [00:08<00:12, 9.39it/s]
41%|#############################################5 | Computing thresholds ... : 83/204 [00:08<00:12, 9.41it/s]
41%|##############################################1 | Computing thresholds ... : 84/204 [00:08<00:12, 9.44it/s]
42%|##############################################6 | Computing thresholds ... : 85/204 [00:08<00:12, 9.46it/s]
42%|###############################################2 | Computing thresholds ... : 86/204 [00:08<00:12, 9.49it/s]
43%|###############################################7 | Computing thresholds ... : 87/204 [00:08<00:12, 9.50it/s]
43%|################################################3 | Computing thresholds ... : 88/204 [00:09<00:12, 9.53it/s]
44%|################################################8 | Computing thresholds ... : 89/204 [00:09<00:12, 9.55it/s]
44%|#################################################4 | Computing thresholds ... : 90/204 [00:09<00:11, 9.55it/s]
45%|#################################################9 | Computing thresholds ... : 91/204 [00:09<00:11, 9.58it/s]
45%|##################################################5 | Computing thresholds ... : 92/204 [00:09<00:11, 9.58it/s]
46%|################################################### | Computing thresholds ... : 93/204 [00:09<00:11, 9.60it/s]
46%|###################################################6 | Computing thresholds ... : 94/204 [00:09<00:11, 9.62it/s]
47%|####################################################1 | Computing thresholds ... : 95/204 [00:09<00:11, 9.64it/s]
47%|####################################################7 | Computing thresholds ... : 96/204 [00:09<00:11, 9.64it/s]
48%|#####################################################2 | Computing thresholds ... : 97/204 [00:09<00:11, 9.66it/s]
48%|#####################################################8 | Computing thresholds ... : 98/204 [00:10<00:10, 9.64it/s]
49%|######################################################3 | Computing thresholds ... : 99/204 [00:10<00:10, 9.65it/s]
49%|######################################################4 | Computing thresholds ... : 100/204 [00:10<00:10, 9.64it/s]
50%|######################################################9 | Computing thresholds ... : 101/204 [00:10<00:10, 9.65it/s]
50%|#######################################################5 | Computing thresholds ... : 102/204 [00:10<00:10, 9.62it/s]
50%|######################################################## | Computing thresholds ... : 103/204 [00:10<00:10, 9.63it/s]
51%|########################################################5 | Computing thresholds ... : 104/204 [00:10<00:10, 9.65it/s]
51%|#########################################################1 | Computing thresholds ... : 105/204 [00:10<00:10, 9.65it/s]
52%|#########################################################6 | Computing thresholds ... : 106/204 [00:10<00:10, 9.50it/s]
52%|##########################################################2 | Computing thresholds ... : 107/204 [00:11<00:10, 9.33it/s]
53%|##########################################################7 | Computing thresholds ... : 108/204 [00:11<00:10, 9.26it/s]
53%|###########################################################3 | Computing thresholds ... : 109/204 [00:11<00:10, 9.19it/s]
54%|###########################################################8 | Computing thresholds ... : 110/204 [00:11<00:10, 9.21it/s]
54%|############################################################3 | Computing thresholds ... : 111/204 [00:11<00:10, 9.16it/s]
55%|############################################################9 | Computing thresholds ... : 112/204 [00:11<00:10, 9.13it/s]
55%|#############################################################4 | Computing thresholds ... : 113/204 [00:11<00:09, 9.15it/s]
56%|############################################################## | Computing thresholds ... : 114/204 [00:11<00:09, 9.14it/s]
56%|##############################################################5 | Computing thresholds ... : 115/204 [00:11<00:09, 9.14it/s]
57%|###############################################################1 | Computing thresholds ... : 116/204 [00:12<00:09, 9.16it/s]
57%|###############################################################6 | Computing thresholds ... : 117/204 [00:12<00:09, 9.10it/s]
58%|################################################################2 | Computing thresholds ... : 118/204 [00:12<00:09, 9.00it/s]
58%|################################################################7 | Computing thresholds ... : 119/204 [00:12<00:09, 8.97it/s]
59%|#################################################################2 | Computing thresholds ... : 120/204 [00:12<00:09, 8.94it/s]
59%|#################################################################8 | Computing thresholds ... : 121/204 [00:12<00:09, 8.96it/s]
60%|##################################################################3 | Computing thresholds ... : 122/204 [00:12<00:09, 8.93it/s]
60%|##################################################################9 | Computing thresholds ... : 123/204 [00:12<00:09, 8.78it/s]
61%|###################################################################4 | Computing thresholds ... : 124/204 [00:13<00:09, 8.83it/s]
61%|#################################################################### | Computing thresholds ... : 125/204 [00:13<00:08, 8.84it/s]
62%|####################################################################5 | Computing thresholds ... : 126/204 [00:13<00:08, 8.79it/s]
62%|#####################################################################1 | Computing thresholds ... : 127/204 [00:13<00:08, 8.81it/s]
63%|#####################################################################6 | Computing thresholds ... : 128/204 [00:13<00:08, 8.88it/s]
63%|######################################################################1 | Computing thresholds ... : 129/204 [00:13<00:08, 8.77it/s]
64%|######################################################################7 | Computing thresholds ... : 130/204 [00:13<00:08, 8.81it/s]
64%|#######################################################################2 | Computing thresholds ... : 131/204 [00:13<00:08, 8.86it/s]
65%|#######################################################################8 | Computing thresholds ... : 132/204 [00:13<00:08, 8.91it/s]
65%|########################################################################3 | Computing thresholds ... : 133/204 [00:14<00:07, 8.89it/s]
66%|########################################################################9 | Computing thresholds ... : 134/204 [00:14<00:07, 8.90it/s]
66%|#########################################################################4 | Computing thresholds ... : 135/204 [00:14<00:07, 8.97it/s]
67%|########################################################################## | Computing thresholds ... : 136/204 [00:14<00:07, 8.98it/s]
67%|##########################################################################5 | Computing thresholds ... : 137/204 [00:14<00:07, 8.98it/s]
68%|########################################################################### | Computing thresholds ... : 138/204 [00:14<00:07, 8.99it/s]
68%|###########################################################################6 | Computing thresholds ... : 139/204 [00:14<00:07, 8.99it/s]
69%|############################################################################1 | Computing thresholds ... : 140/204 [00:14<00:07, 9.00it/s]
69%|############################################################################7 | Computing thresholds ... : 141/204 [00:14<00:06, 9.01it/s]
70%|#############################################################################2 | Computing thresholds ... : 142/204 [00:15<00:06, 9.08it/s]
70%|#############################################################################8 | Computing thresholds ... : 143/204 [00:15<00:06, 9.08it/s]
71%|##############################################################################3 | Computing thresholds ... : 144/204 [00:15<00:06, 9.08it/s]
71%|##############################################################################8 | Computing thresholds ... : 145/204 [00:15<00:06, 9.15it/s]
72%|###############################################################################4 | Computing thresholds ... : 146/204 [00:15<00:06, 9.21it/s]
72%|###############################################################################9 | Computing thresholds ... : 147/204 [00:15<00:06, 9.21it/s]
73%|################################################################################5 | Computing thresholds ... : 148/204 [00:15<00:06, 9.19it/s]
73%|################################################################################# | Computing thresholds ... : 149/204 [00:15<00:05, 9.23it/s]
74%|#################################################################################6 | Computing thresholds ... : 150/204 [00:15<00:05, 9.32it/s]
74%|##################################################################################1 | Computing thresholds ... : 151/204 [00:15<00:05, 9.29it/s]
75%|##################################################################################7 | Computing thresholds ... : 152/204 [00:16<00:05, 9.33it/s]
75%|###################################################################################2 | Computing thresholds ... : 153/204 [00:16<00:05, 9.36it/s]
75%|###################################################################################7 | Computing thresholds ... : 154/204 [00:16<00:05, 9.42it/s]
76%|####################################################################################3 | Computing thresholds ... : 155/204 [00:16<00:05, 9.47it/s]
76%|####################################################################################8 | Computing thresholds ... : 156/204 [00:16<00:05, 9.52it/s]
77%|#####################################################################################4 | Computing thresholds ... : 157/204 [00:16<00:04, 9.50it/s]
77%|#####################################################################################9 | Computing thresholds ... : 158/204 [00:16<00:04, 9.55it/s]
78%|######################################################################################5 | Computing thresholds ... : 159/204 [00:16<00:04, 9.60it/s]
78%|####################################################################################### | Computing thresholds ... : 160/204 [00:16<00:04, 9.57it/s]
79%|#######################################################################################6 | Computing thresholds ... : 161/204 [00:16<00:04, 9.62it/s]
79%|########################################################################################1 | Computing thresholds ... : 162/204 [00:17<00:04, 9.67it/s]
80%|########################################################################################6 | Computing thresholds ... : 163/204 [00:17<00:04, 9.64it/s]
80%|#########################################################################################2 | Computing thresholds ... : 164/204 [00:17<00:04, 9.68it/s]
81%|#########################################################################################7 | Computing thresholds ... : 165/204 [00:17<00:04, 9.72it/s]
81%|##########################################################################################3 | Computing thresholds ... : 166/204 [00:17<00:03, 9.69it/s]
82%|##########################################################################################8 | Computing thresholds ... : 167/204 [00:17<00:03, 9.72it/s]
82%|###########################################################################################4 | Computing thresholds ... : 168/204 [00:17<00:03, 9.77it/s]
83%|###########################################################################################9 | Computing thresholds ... : 169/204 [00:17<00:03, 9.80it/s]
83%|############################################################################################5 | Computing thresholds ... : 170/204 [00:17<00:03, 9.77it/s]
84%|############################################################################################# | Computing thresholds ... : 171/204 [00:17<00:03, 9.81it/s]
84%|#############################################################################################5 | Computing thresholds ... : 172/204 [00:18<00:03, 9.77it/s]
85%|##############################################################################################1 | Computing thresholds ... : 173/204 [00:18<00:03, 9.81it/s]
85%|##############################################################################################6 | Computing thresholds ... : 174/204 [00:18<00:03, 9.77it/s]
86%|###############################################################################################2 | Computing thresholds ... : 175/204 [00:18<00:02, 9.81it/s]
86%|###############################################################################################7 | Computing thresholds ... : 176/204 [00:18<00:02, 9.77it/s]
87%|################################################################################################3 | Computing thresholds ... : 177/204 [00:18<00:02, 9.81it/s]
87%|################################################################################################8 | Computing thresholds ... : 178/204 [00:18<00:02, 9.85it/s]
88%|#################################################################################################3 | Computing thresholds ... : 179/204 [00:18<00:02, 9.81it/s]
88%|#################################################################################################9 | Computing thresholds ... : 180/204 [00:18<00:02, 9.75it/s]
89%|##################################################################################################4 | Computing thresholds ... : 181/204 [00:18<00:02, 9.76it/s]
89%|################################################################################################### | Computing thresholds ... : 182/204 [00:19<00:02, 9.77it/s]
90%|###################################################################################################5 | Computing thresholds ... : 183/204 [00:19<00:02, 9.78it/s]
90%|####################################################################################################1 | Computing thresholds ... : 184/204 [00:19<00:02, 9.85it/s]
91%|####################################################################################################6 | Computing thresholds ... : 185/204 [00:19<00:01, 9.81it/s]
91%|#####################################################################################################2 | Computing thresholds ... : 186/204 [00:19<00:01, 9.81it/s]
92%|#####################################################################################################7 | Computing thresholds ... : 187/204 [00:19<00:01, 9.89it/s]
92%|######################################################################################################2 | Computing thresholds ... : 188/204 [00:19<00:01, 9.85it/s]
93%|######################################################################################################8 | Computing thresholds ... : 189/204 [00:19<00:01, 9.89it/s]
93%|#######################################################################################################3 | Computing thresholds ... : 190/204 [00:19<00:01, 9.84it/s]
94%|#######################################################################################################9 | Computing thresholds ... : 191/204 [00:19<00:01, 9.80it/s]
94%|########################################################################################################4 | Computing thresholds ... : 192/204 [00:20<00:01, 9.76it/s]
95%|######################################################################################################### | Computing thresholds ... : 193/204 [00:20<00:01, 9.80it/s]
95%|#########################################################################################################5 | Computing thresholds ... : 194/204 [00:20<00:01, 9.84it/s]
96%|##########################################################################################################1 | Computing thresholds ... : 195/204 [00:20<00:00, 9.87it/s]
96%|##########################################################################################################6 | Computing thresholds ... : 196/204 [00:20<00:00, 9.84it/s]
97%|###########################################################################################################1 | Computing thresholds ... : 197/204 [00:20<00:00, 9.87it/s]
97%|###########################################################################################################7 | Computing thresholds ... : 198/204 [00:20<00:00, 9.89it/s]
98%|############################################################################################################2 | Computing thresholds ... : 199/204 [00:20<00:00, 9.87it/s]
98%|############################################################################################################8 | Computing thresholds ... : 200/204 [00:20<00:00, 9.82it/s]
99%|#############################################################################################################3 | Computing thresholds ... : 201/204 [00:20<00:00, 9.79it/s]
99%|#############################################################################################################9 | Computing thresholds ... : 202/204 [00:21<00:00, 9.75it/s]
100%|##############################################################################################################4| Computing thresholds ... : 203/204 [00:21<00:00, 9.79it/s]
100%|###############################################################################################################| Computing thresholds ... : 204/204 [00:21<00:00, 9.75it/s]
100%|###############################################################################################################| Computing thresholds ... : 204/204 [00:21<00:00, 9.58it/s]
0%| | Repairing epochs : 0/72 [00:00<?, ?it/s]
3%|###3 | Repairing epochs : 2/72 [00:00<00:01, 42.68it/s]
4%|##### | Repairing epochs : 3/72 [00:00<00:01, 47.91it/s]
6%|######7 | Repairing epochs : 4/72 [00:00<00:01, 42.30it/s]
8%|##########1 | Repairing epochs : 6/72 [00:00<00:01, 48.17it/s]
10%|###########8 | Repairing epochs : 7/72 [00:00<00:01, 44.46it/s]
12%|###############2 | Repairing epochs : 9/72 [00:00<00:01, 44.00it/s]
14%|################8 | Repairing epochs : 10/72 [00:00<00:01, 42.15it/s]
15%|##################4 | Repairing epochs : 11/72 [00:00<00:01, 40.67it/s]
18%|#####################8 | Repairing epochs : 13/72 [00:00<00:01, 41.03it/s]
21%|#########################2 | Repairing epochs : 15/72 [00:00<00:01, 41.23it/s]
24%|############################5 | Repairing epochs : 17/72 [00:00<00:01, 41.44it/s]
26%|###############################9 | Repairing epochs : 19/72 [00:00<00:01, 41.37it/s]
29%|###################################2 | Repairing epochs : 21/72 [00:00<00:01, 41.70it/s]
32%|######################################6 | Repairing epochs : 23/72 [00:00<00:01, 41.81it/s]
33%|########################################3 | Repairing epochs : 24/72 [00:00<00:01, 42.61it/s]
35%|########################################## | Repairing epochs : 25/72 [00:00<00:01, 41.82it/s]
38%|#############################################3 | Repairing epochs : 27/72 [00:00<00:01, 43.43it/s]
39%|############################################### | Repairing epochs : 28/72 [00:00<00:01, 42.58it/s]
42%|##################################################4 | Repairing epochs : 30/72 [00:00<00:00, 42.59it/s]
44%|#####################################################7 | Repairing epochs : 32/72 [00:00<00:00, 44.00it/s]
46%|#######################################################4 | Repairing epochs : 33/72 [00:00<00:00, 43.19it/s]
49%|##########################################################8 | Repairing epochs : 35/72 [00:00<00:00, 43.14it/s]
51%|##############################################################1 | Repairing epochs : 37/72 [00:00<00:00, 43.07it/s]
54%|#################################################################5 | Repairing epochs : 39/72 [00:00<00:00, 44.30it/s]
56%|###################################################################2 | Repairing epochs : 40/72 [00:00<00:00, 43.58it/s]
58%|######################################################################5 | Repairing epochs : 42/72 [00:00<00:00, 44.68it/s]
60%|########################################################################2 | Repairing epochs : 43/72 [00:00<00:00, 44.00it/s]
62%|###########################################################################6 | Repairing epochs : 45/72 [00:01<00:00, 45.14it/s]
64%|#############################################################################3 | Repairing epochs : 46/72 [00:01<00:00, 44.40it/s]
65%|##############################################################################9 | Repairing epochs : 47/72 [00:01<00:00, 44.93it/s]
68%|##################################################################################3 | Repairing epochs : 49/72 [00:01<00:00, 44.74it/s]
71%|#####################################################################################7 | Repairing epochs : 51/72 [00:01<00:00, 44.78it/s]
72%|#######################################################################################3 | Repairing epochs : 52/72 [00:01<00:00, 45.07it/s]
75%|##########################################################################################7 | Repairing epochs : 54/72 [00:01<00:00, 46.11it/s]
76%|############################################################################################4 | Repairing epochs : 55/72 [00:01<00:00, 45.34it/s]
78%|##############################################################################################1 | Repairing epochs : 56/72 [00:01<00:00, 45.57it/s]
79%|###############################################################################################7 | Repairing epochs : 57/72 [00:01<00:00, 45.07it/s]
82%|###################################################################################################1 | Repairing epochs : 59/72 [00:01<00:00, 44.88it/s]
85%|######################################################################################################5 | Repairing epochs : 61/72 [00:01<00:00, 45.87it/s]
86%|########################################################################################################1 | Repairing epochs : 62/72 [00:01<00:00, 45.13it/s]
89%|###########################################################################################################5 | Repairing epochs : 64/72 [00:01<00:00, 46.13it/s]
90%|#############################################################################################################2 | Repairing epochs : 65/72 [00:01<00:00, 45.39it/s]
93%|################################################################################################################5 | Repairing epochs : 67/72 [00:01<00:00, 45.18it/s]
96%|###################################################################################################################9 | Repairing epochs : 69/72 [00:01<00:00, 46.15it/s]
97%|#####################################################################################################################6 | Repairing epochs : 70/72 [00:01<00:00, 45.39it/s]
100%|#########################################################################################################################| Repairing epochs : 72/72 [00:01<00:00, 46.35it/s]
100%|#########################################################################################################################| Repairing epochs : 72/72 [00:01<00:00, 45.01it/s]
0%| | n_interp : 0/3 [00:00<?, ?it/s]
0%| | Repairing epochs : 0/72 [00:00<?, ?it/s]
1%|#6 | Repairing epochs : 1/72 [00:04<05:14, 4.42s/it]
3%|###3 | Repairing epochs : 2/72 [00:04<02:35, 2.23s/it]
4%|##### | Repairing epochs : 3/72 [00:04<01:43, 1.50s/it]
6%|######7 | Repairing epochs : 4/72 [00:04<01:16, 1.13s/it]
7%|########4 | Repairing epochs : 5/72 [00:04<01:00, 1.10it/s]
8%|##########1 | Repairing epochs : 6/72 [00:05<00:50, 1.31it/s]
10%|###########8 | Repairing epochs : 7/72 [00:05<00:42, 1.53it/s]
11%|#############5 | Repairing epochs : 8/72 [00:05<00:37, 1.73it/s]
12%|###############2 | Repairing epochs : 9/72 [00:05<00:32, 1.92it/s]
14%|################8 | Repairing epochs : 10/72 [00:05<00:29, 2.12it/s]
15%|##################4 | Repairing epochs : 11/72 [00:05<00:26, 2.31it/s]
17%|####################1 | Repairing epochs : 12/72 [00:05<00:23, 2.50it/s]
18%|#####################8 | Repairing epochs : 13/72 [00:06<00:21, 2.68it/s]
19%|#######################5 | Repairing epochs : 14/72 [00:06<00:20, 2.86it/s]
21%|#########################2 | Repairing epochs : 15/72 [00:06<00:18, 3.04it/s]
22%|##########################8 | Repairing epochs : 16/72 [00:06<00:17, 3.20it/s]
24%|############################5 | Repairing epochs : 17/72 [00:06<00:16, 3.36it/s]
25%|##############################2 | Repairing epochs : 18/72 [00:06<00:15, 3.51it/s]
26%|###############################9 | Repairing epochs : 19/72 [00:06<00:14, 3.68it/s]
28%|#################################6 | Repairing epochs : 20/72 [00:06<00:13, 3.82it/s]
29%|###################################2 | Repairing epochs : 21/72 [00:07<00:12, 3.98it/s]
31%|####################################9 | Repairing epochs : 22/72 [00:07<00:12, 4.11it/s]
32%|######################################6 | Repairing epochs : 23/72 [00:07<00:11, 4.26it/s]
33%|########################################3 | Repairing epochs : 24/72 [00:07<00:10, 4.38it/s]
35%|########################################## | Repairing epochs : 25/72 [00:07<00:10, 4.50it/s]
36%|###########################################6 | Repairing epochs : 26/72 [00:07<00:09, 4.64it/s]
38%|#############################################3 | Repairing epochs : 27/72 [00:07<00:09, 4.75it/s]
39%|############################################### | Repairing epochs : 28/72 [00:08<00:09, 4.81it/s]
40%|################################################7 | Repairing epochs : 29/72 [00:08<00:08, 4.88it/s]
42%|##################################################4 | Repairing epochs : 30/72 [00:08<00:08, 4.98it/s]
43%|#################################################### | Repairing epochs : 31/72 [00:08<00:08, 5.07it/s]
44%|#####################################################7 | Repairing epochs : 32/72 [00:08<00:07, 5.19it/s]
46%|#######################################################4 | Repairing epochs : 33/72 [00:08<00:07, 5.28it/s]
47%|#########################################################1 | Repairing epochs : 34/72 [00:08<00:07, 5.34it/s]
49%|##########################################################8 | Repairing epochs : 35/72 [00:09<00:06, 5.44it/s]
50%|############################################################5 | Repairing epochs : 36/72 [00:09<00:06, 5.52it/s]
51%|##############################################################1 | Repairing epochs : 37/72 [00:09<00:06, 5.59it/s]
53%|###############################################################8 | Repairing epochs : 38/72 [00:09<00:05, 5.69it/s]
54%|#################################################################5 | Repairing epochs : 39/72 [00:09<00:05, 5.76it/s]
56%|###################################################################2 | Repairing epochs : 40/72 [00:09<00:05, 5.85it/s]
57%|####################################################################9 | Repairing epochs : 41/72 [00:09<00:05, 5.94it/s]
58%|######################################################################5 | Repairing epochs : 42/72 [00:10<00:05, 6.00it/s]
60%|########################################################################2 | Repairing epochs : 43/72 [00:10<00:04, 6.08it/s]
61%|#########################################################################9 | Repairing epochs : 44/72 [00:10<00:04, 6.13it/s]
62%|###########################################################################6 | Repairing epochs : 45/72 [00:10<00:04, 6.21it/s]
64%|#############################################################################3 | Repairing epochs : 46/72 [00:10<00:04, 6.28it/s]
65%|##############################################################################9 | Repairing epochs : 47/72 [00:10<00:03, 6.32it/s]
67%|################################################################################6 | Repairing epochs : 48/72 [00:10<00:03, 6.40it/s]
68%|##################################################################################3 | Repairing epochs : 49/72 [00:10<00:03, 6.44it/s]
69%|#################################################################################### | Repairing epochs : 50/72 [00:11<00:03, 6.53it/s]
71%|#####################################################################################7 | Repairing epochs : 51/72 [00:11<00:03, 6.56it/s]
72%|#######################################################################################3 | Repairing epochs : 52/72 [00:11<00:03, 6.62it/s]
74%|######################################################################################### | Repairing epochs : 53/72 [00:11<00:02, 6.68it/s]
75%|##########################################################################################7 | Repairing epochs : 54/72 [00:11<00:02, 6.74it/s]
76%|############################################################################################4 | Repairing epochs : 55/72 [00:11<00:02, 6.76it/s]
78%|##############################################################################################1 | Repairing epochs : 56/72 [00:11<00:02, 6.81it/s]
79%|###############################################################################################7 | Repairing epochs : 57/72 [00:11<00:02, 6.83it/s]
81%|#################################################################################################4 | Repairing epochs : 58/72 [00:12<00:02, 6.88it/s]
82%|###################################################################################################1 | Repairing epochs : 59/72 [00:12<00:01, 6.90it/s]
83%|####################################################################################################8 | Repairing epochs : 60/72 [00:12<00:01, 6.94it/s]
85%|######################################################################################################5 | Repairing epochs : 61/72 [00:12<00:01, 6.95it/s]
86%|########################################################################################################1 | Repairing epochs : 62/72 [00:12<00:01, 6.95it/s]
88%|#########################################################################################################8 | Repairing epochs : 63/72 [00:12<00:01, 6.96it/s]
89%|###########################################################################################################5 | Repairing epochs : 64/72 [00:12<00:01, 7.01it/s]
90%|#############################################################################################################2 | Repairing epochs : 65/72 [00:13<00:00, 7.05it/s]
92%|##############################################################################################################9 | Repairing epochs : 66/72 [00:13<00:00, 7.05it/s]
93%|################################################################################################################5 | Repairing epochs : 67/72 [00:13<00:00, 7.10it/s]
94%|##################################################################################################################2 | Repairing epochs : 68/72 [00:13<00:00, 7.12it/s]
96%|###################################################################################################################9 | Repairing epochs : 69/72 [00:13<00:00, 7.13it/s]
97%|#####################################################################################################################6 | Repairing epochs : 70/72 [00:13<00:00, 7.17it/s]
99%|#######################################################################################################################3 | Repairing epochs : 71/72 [00:13<00:00, 7.17it/s]
100%|#########################################################################################################################| Repairing epochs : 72/72 [00:13<00:00, 7.21it/s]
100%|#########################################################################################################################| Repairing epochs : 72/72 [00:13<00:00, 5.15it/s]
0%| | Fold : 0/10 [00:00<?, ?it/s]
10%|#############4 | Fold : 1/10 [00:00<00:01, 4.55it/s]
20%|##########################8 | Fold : 2/10 [00:00<00:01, 4.35it/s]
30%|########################################1 | Fold : 3/10 [00:00<00:01, 4.34it/s]
40%|#####################################################6 | Fold : 4/10 [00:00<00:01, 4.32it/s]
50%|################################################################### | Fold : 5/10 [00:01<00:01, 4.37it/s]
60%|################################################################################3 | Fold : 6/10 [00:01<00:00, 4.34it/s]
70%|#############################################################################################8 | Fold : 7/10 [00:01<00:00, 4.38it/s]
80%|###########################################################################################################2 | Fold : 8/10 [00:01<00:00, 4.36it/s]
90%|########################################################################################################################6 | Fold : 9/10 [00:02<00:00, 4.34it/s]
100%|#####################################################################################################################################| Fold : 10/10 [00:02<00:00, 4.33it/s]
100%|#####################################################################################################################################| Fold : 10/10 [00:02<00:00, 4.33it/s]
33%|###########################################6 | n_interp : 1/3 [00:16<00:33, 16.71s/it]
0%| | Repairing epochs : 0/72 [00:00<?, ?it/s]
1%|#6 | Repairing epochs : 1/72 [00:00<00:10, 7.09it/s]
3%|###3 | Repairing epochs : 2/72 [00:00<00:09, 7.09it/s]
4%|##### | Repairing epochs : 3/72 [00:00<00:09, 7.37it/s]
6%|######7 | Repairing epochs : 4/72 [00:00<00:09, 7.29it/s]
7%|########4 | Repairing epochs : 5/72 [00:00<00:09, 7.43it/s]
8%|##########1 | Repairing epochs : 6/72 [00:00<00:08, 7.53it/s]
10%|###########8 | Repairing epochs : 7/72 [00:00<00:08, 7.60it/s]
11%|#############5 | Repairing epochs : 8/72 [00:01<00:08, 7.65it/s]
12%|###############2 | Repairing epochs : 9/72 [00:01<00:08, 7.53it/s]
14%|################8 | Repairing epochs : 10/72 [00:01<00:08, 7.62it/s]
15%|##################4 | Repairing epochs : 11/72 [00:01<00:08, 7.55it/s]
17%|####################1 | Repairing epochs : 12/72 [00:01<00:07, 7.51it/s]
18%|#####################8 | Repairing epochs : 13/72 [00:01<00:07, 7.54it/s]
19%|#######################5 | Repairing epochs : 14/72 [00:01<00:07, 7.58it/s]
21%|#########################2 | Repairing epochs : 15/72 [00:01<00:07, 7.54it/s]
22%|##########################8 | Repairing epochs : 16/72 [00:02<00:07, 7.57it/s]
24%|############################5 | Repairing epochs : 17/72 [00:02<00:07, 7.60it/s]
25%|##############################2 | Repairing epochs : 18/72 [00:02<00:07, 7.63it/s]
26%|###############################9 | Repairing epochs : 19/72 [00:02<00:06, 7.59it/s]
28%|#################################6 | Repairing epochs : 20/72 [00:02<00:06, 7.61it/s]
29%|###################################2 | Repairing epochs : 21/72 [00:02<00:06, 7.64it/s]
31%|####################################9 | Repairing epochs : 22/72 [00:02<00:06, 7.66it/s]
32%|######################################6 | Repairing epochs : 23/72 [00:03<00:06, 7.68it/s]
33%|########################################3 | Repairing epochs : 24/72 [00:03<00:06, 7.70it/s]
35%|########################################## | Repairing epochs : 25/72 [00:03<00:06, 7.66it/s]
36%|###########################################6 | Repairing epochs : 26/72 [00:03<00:05, 7.68it/s]
38%|#############################################3 | Repairing epochs : 27/72 [00:03<00:05, 7.64it/s]
39%|############################################### | Repairing epochs : 28/72 [00:03<00:05, 7.66it/s]
40%|################################################7 | Repairing epochs : 29/72 [00:03<00:05, 7.65it/s]
42%|##################################################4 | Repairing epochs : 30/72 [00:03<00:05, 7.70it/s]
43%|#################################################### | Repairing epochs : 31/72 [00:04<00:05, 7.71it/s]
44%|#####################################################7 | Repairing epochs : 32/72 [00:04<00:05, 7.73it/s]
46%|#######################################################4 | Repairing epochs : 33/72 [00:04<00:05, 7.69it/s]
47%|#########################################################1 | Repairing epochs : 34/72 [00:04<00:04, 7.65it/s]
49%|##########################################################8 | Repairing epochs : 35/72 [00:04<00:04, 7.67it/s]
50%|############################################################5 | Repairing epochs : 36/72 [00:04<00:04, 7.68it/s]
51%|##############################################################1 | Repairing epochs : 37/72 [00:04<00:04, 7.64it/s]
53%|###############################################################8 | Repairing epochs : 38/72 [00:04<00:04, 7.66it/s]
54%|#################################################################5 | Repairing epochs : 39/72 [00:05<00:04, 7.66it/s]
56%|###################################################################2 | Repairing epochs : 40/72 [00:05<00:04, 7.70it/s]
57%|####################################################################9 | Repairing epochs : 41/72 [00:05<00:04, 7.66it/s]
58%|######################################################################5 | Repairing epochs : 42/72 [00:05<00:03, 7.68it/s]
60%|########################################################################2 | Repairing epochs : 43/72 [00:05<00:03, 7.64it/s]
61%|#########################################################################9 | Repairing epochs : 44/72 [00:05<00:03, 7.66it/s]
62%|###########################################################################6 | Repairing epochs : 45/72 [00:05<00:03, 7.68it/s]
64%|#############################################################################3 | Repairing epochs : 46/72 [00:06<00:03, 7.69it/s]
65%|##############################################################################9 | Repairing epochs : 47/72 [00:06<00:03, 7.71it/s]
67%|################################################################################6 | Repairing epochs : 48/72 [00:06<00:03, 7.72it/s]
68%|##################################################################################3 | Repairing epochs : 49/72 [00:06<00:02, 7.68it/s]
69%|#################################################################################### | Repairing epochs : 50/72 [00:06<00:02, 7.55it/s]
71%|#####################################################################################7 | Repairing epochs : 51/72 [00:06<00:02, 7.54it/s]
72%|#######################################################################################3 | Repairing epochs : 52/72 [00:06<00:02, 7.50it/s]
74%|######################################################################################### | Repairing epochs : 53/72 [00:06<00:02, 7.52it/s]
75%|##########################################################################################7 | Repairing epochs : 54/72 [00:07<00:02, 7.53it/s]
76%|############################################################################################4 | Repairing epochs : 55/72 [00:07<00:02, 7.56it/s]
78%|##############################################################################################1 | Repairing epochs : 56/72 [00:07<00:02, 7.54it/s]
79%|###############################################################################################7 | Repairing epochs : 57/72 [00:07<00:02, 7.47it/s]
81%|#################################################################################################4 | Repairing epochs : 58/72 [00:07<00:01, 7.49it/s]
82%|###################################################################################################1 | Repairing epochs : 59/72 [00:07<00:01, 7.52it/s]
83%|####################################################################################################8 | Repairing epochs : 60/72 [00:07<00:01, 7.52it/s]
85%|######################################################################################################5 | Repairing epochs : 61/72 [00:08<00:01, 7.51it/s]
86%|########################################################################################################1 | Repairing epochs : 62/72 [00:08<00:01, 7.54it/s]
88%|#########################################################################################################8 | Repairing epochs : 63/72 [00:08<00:01, 7.52it/s]
89%|###########################################################################################################5 | Repairing epochs : 64/72 [00:08<00:01, 7.53it/s]
90%|#############################################################################################################2 | Repairing epochs : 65/72 [00:08<00:00, 7.51it/s]
92%|##############################################################################################################9 | Repairing epochs : 66/72 [00:08<00:00, 7.53it/s]
93%|################################################################################################################5 | Repairing epochs : 67/72 [00:08<00:00, 7.55it/s]
94%|##################################################################################################################2 | Repairing epochs : 68/72 [00:08<00:00, 7.57it/s]
96%|###################################################################################################################9 | Repairing epochs : 69/72 [00:09<00:00, 7.59it/s]
97%|#####################################################################################################################6 | Repairing epochs : 70/72 [00:09<00:00, 7.57it/s]
99%|#######################################################################################################################3 | Repairing epochs : 71/72 [00:09<00:00, 7.59it/s]
100%|#########################################################################################################################| Repairing epochs : 72/72 [00:09<00:00, 7.60it/s]
100%|#########################################################################################################################| Repairing epochs : 72/72 [00:09<00:00, 7.60it/s]
0%| | Fold : 0/10 [00:00<?, ?it/s]
10%|#############4 | Fold : 1/10 [00:00<00:02, 4.24it/s]
20%|##########################8 | Fold : 2/10 [00:00<00:01, 4.25it/s]
30%|########################################1 | Fold : 3/10 [00:00<00:01, 4.25it/s]
40%|#####################################################6 | Fold : 4/10 [00:00<00:01, 4.32it/s]
50%|################################################################### | Fold : 5/10 [00:01<00:01, 4.31it/s]
60%|################################################################################3 | Fold : 6/10 [00:01<00:00, 4.35it/s]
70%|#############################################################################################8 | Fold : 7/10 [00:01<00:00, 4.34it/s]
80%|###########################################################################################################2 | Fold : 8/10 [00:01<00:00, 4.32it/s]
90%|########################################################################################################################6 | Fold : 9/10 [00:02<00:00, 4.31it/s]
100%|#####################################################################################################################################| Fold : 10/10 [00:02<00:00, 4.31it/s]
100%|#####################################################################################################################################| Fold : 10/10 [00:02<00:00, 4.31it/s]
67%|#######################################################################################3 | n_interp : 2/3 [00:28<00:14, 14.38s/it]
0%| | Repairing epochs : 0/72 [00:00<?, ?it/s]
1%|#6 | Repairing epochs : 1/72 [00:00<00:08, 7.97it/s]
3%|###3 | Repairing epochs : 2/72 [00:00<00:08, 7.96it/s]
4%|##### | Repairing epochs : 3/72 [00:00<00:08, 7.97it/s]
6%|######7 | Repairing epochs : 4/72 [00:00<00:08, 7.97it/s]
7%|########4 | Repairing epochs : 5/72 [00:00<00:08, 7.75it/s]
8%|##########1 | Repairing epochs : 6/72 [00:00<00:08, 7.79it/s]
10%|###########8 | Repairing epochs : 7/72 [00:00<00:08, 7.67it/s]
11%|#############5 | Repairing epochs : 8/72 [00:01<00:08, 7.71it/s]
12%|###############2 | Repairing epochs : 9/72 [00:01<00:08, 7.75it/s]
14%|################8 | Repairing epochs : 10/72 [00:01<00:07, 7.77it/s]
15%|##################4 | Repairing epochs : 11/72 [00:01<00:07, 7.80it/s]
17%|####################1 | Repairing epochs : 12/72 [00:01<00:07, 7.81it/s]
18%|#####################8 | Repairing epochs : 13/72 [00:01<00:07, 7.73it/s]
19%|#######################5 | Repairing epochs : 14/72 [00:01<00:07, 7.76it/s]
21%|#########################2 | Repairing epochs : 15/72 [00:01<00:07, 7.69it/s]
22%|##########################8 | Repairing epochs : 16/72 [00:02<00:07, 7.79it/s]
24%|############################5 | Repairing epochs : 17/72 [00:02<00:07, 7.73it/s]
25%|##############################2 | Repairing epochs : 18/72 [00:02<00:06, 7.75it/s]
26%|###############################9 | Repairing epochs : 19/72 [00:02<00:06, 7.76it/s]
28%|#################################6 | Repairing epochs : 20/72 [00:02<00:06, 7.78it/s]
29%|###################################2 | Repairing epochs : 21/72 [00:02<00:06, 7.87it/s]
31%|####################################9 | Repairing epochs : 22/72 [00:02<00:06, 7.81it/s]
32%|######################################6 | Repairing epochs : 23/72 [00:02<00:06, 7.68it/s]
33%|########################################3 | Repairing epochs : 24/72 [00:03<00:06, 7.57it/s]
35%|########################################## | Repairing epochs : 25/72 [00:03<00:06, 7.60it/s]
36%|###########################################6 | Repairing epochs : 26/72 [00:03<00:06, 7.56it/s]
38%|#############################################3 | Repairing epochs : 27/72 [00:03<00:05, 7.56it/s]
39%|############################################### | Repairing epochs : 28/72 [00:03<00:05, 7.55it/s]
40%|################################################7 | Repairing epochs : 29/72 [00:03<00:05, 7.58it/s]
42%|##################################################4 | Repairing epochs : 30/72 [00:03<00:05, 7.54it/s]
43%|#################################################### | Repairing epochs : 31/72 [00:04<00:05, 7.57it/s]
44%|#####################################################7 | Repairing epochs : 32/72 [00:04<00:05, 7.54it/s]
46%|#######################################################4 | Repairing epochs : 33/72 [00:04<00:05, 7.56it/s]
47%|#########################################################1 | Repairing epochs : 34/72 [00:04<00:05, 7.58it/s]
49%|##########################################################8 | Repairing epochs : 35/72 [00:04<00:04, 7.61it/s]
50%|############################################################5 | Repairing epochs : 36/72 [00:04<00:04, 7.57it/s]
51%|##############################################################1 | Repairing epochs : 37/72 [00:04<00:04, 7.60it/s]
53%|###############################################################8 | Repairing epochs : 38/72 [00:04<00:04, 7.56it/s]
54%|#################################################################5 | Repairing epochs : 39/72 [00:05<00:04, 7.59it/s]
56%|###################################################################2 | Repairing epochs : 40/72 [00:05<00:04, 7.60it/s]
57%|####################################################################9 | Repairing epochs : 41/72 [00:05<00:04, 7.63it/s]
58%|######################################################################5 | Repairing epochs : 42/72 [00:05<00:03, 7.65it/s]
60%|########################################################################2 | Repairing epochs : 43/72 [00:05<00:03, 7.61it/s]
61%|#########################################################################9 | Repairing epochs : 44/72 [00:05<00:03, 7.63it/s]
62%|###########################################################################6 | Repairing epochs : 45/72 [00:05<00:03, 7.65it/s]
64%|#############################################################################3 | Repairing epochs : 46/72 [00:06<00:03, 7.67it/s]
65%|##############################################################################9 | Repairing epochs : 47/72 [00:06<00:03, 7.58it/s]
67%|################################################################################6 | Repairing epochs : 48/72 [00:06<00:03, 7.65it/s]
68%|##################################################################################3 | Repairing epochs : 49/72 [00:06<00:02, 7.67it/s]
69%|#################################################################################### | Repairing epochs : 50/72 [00:06<00:02, 7.69it/s]
71%|#####################################################################################7 | Repairing epochs : 51/72 [00:06<00:02, 7.70it/s]
72%|#######################################################################################3 | Repairing epochs : 52/72 [00:06<00:02, 7.71it/s]
74%|######################################################################################### | Repairing epochs : 53/72 [00:06<00:02, 7.73it/s]
75%|##########################################################################################7 | Repairing epochs : 54/72 [00:07<00:02, 7.69it/s]
76%|############################################################################################4 | Repairing epochs : 55/72 [00:07<00:02, 7.65it/s]
78%|##############################################################################################1 | Repairing epochs : 56/72 [00:07<00:02, 7.62it/s]
79%|###############################################################################################7 | Repairing epochs : 57/72 [00:07<00:01, 7.64it/s]
81%|#################################################################################################4 | Repairing epochs : 58/72 [00:07<00:01, 7.61it/s]
82%|###################################################################################################1 | Repairing epochs : 59/72 [00:07<00:01, 7.63it/s]
83%|####################################################################################################8 | Repairing epochs : 60/72 [00:07<00:01, 7.64it/s]
85%|######################################################################################################5 | Repairing epochs : 61/72 [00:07<00:01, 7.61it/s]
86%|########################################################################################################1 | Repairing epochs : 62/72 [00:08<00:01, 7.58it/s]
88%|#########################################################################################################8 | Repairing epochs : 63/72 [00:08<00:01, 7.56it/s]
89%|###########################################################################################################5 | Repairing epochs : 64/72 [00:08<00:01, 7.62it/s]
90%|#############################################################################################################2 | Repairing epochs : 65/72 [00:08<00:00, 7.64it/s]
92%|##############################################################################################################9 | Repairing epochs : 66/72 [00:08<00:00, 7.66it/s]
93%|################################################################################################################5 | Repairing epochs : 67/72 [00:08<00:00, 7.67it/s]
94%|##################################################################################################################2 | Repairing epochs : 68/72 [00:08<00:00, 7.64it/s]
96%|###################################################################################################################9 | Repairing epochs : 69/72 [00:09<00:00, 7.66it/s]
97%|#####################################################################################################################6 | Repairing epochs : 70/72 [00:09<00:00, 7.66it/s]
99%|#######################################################################################################################3 | Repairing epochs : 71/72 [00:09<00:00, 7.69it/s]
100%|#########################################################################################################################| Repairing epochs : 72/72 [00:09<00:00, 7.70it/s]
100%|#########################################################################################################################| Repairing epochs : 72/72 [00:09<00:00, 7.66it/s]
0%| | Fold : 0/10 [00:00<?, ?it/s]
10%|#############4 | Fold : 1/10 [00:00<00:01, 4.90it/s]
20%|##########################8 | Fold : 2/10 [00:00<00:01, 4.72it/s]
30%|########################################1 | Fold : 3/10 [00:00<00:01, 4.69it/s]
40%|#####################################################6 | Fold : 4/10 [00:00<00:01, 4.72it/s]
50%|################################################################### | Fold : 5/10 [00:01<00:01, 4.76it/s]
60%|################################################################################3 | Fold : 6/10 [00:01<00:00, 4.72it/s]
70%|#############################################################################################8 | Fold : 7/10 [00:01<00:00, 4.75it/s]
80%|###########################################################################################################2 | Fold : 8/10 [00:01<00:00, 4.72it/s]
90%|########################################################################################################################6 | Fold : 9/10 [00:01<00:00, 4.74it/s]
100%|#####################################################################################################################################| Fold : 10/10 [00:02<00:00, 4.72it/s]
100%|#####################################################################################################################################| Fold : 10/10 [00:02<00:00, 4.72it/s]
100%|###################################################################################################################################| n_interp : 3/3 [00:40<00:00, 13.41s/it]
100%|###################################################################################################################################| n_interp : 3/3 [00:40<00:00, 13.50s/it]
Estimated consensus=0.50 and n_interpolate=4
0%| | Repairing epochs : 0/72 [00:00<?, ?it/s]
1%|#6 | Repairing epochs : 1/72 [00:00<00:08, 7.97it/s]
3%|###3 | Repairing epochs : 2/72 [00:00<00:09, 7.54it/s]
4%|##### | Repairing epochs : 3/72 [00:00<00:09, 7.65it/s]
6%|######7 | Repairing epochs : 4/72 [00:00<00:08, 7.73it/s]
7%|########4 | Repairing epochs : 5/72 [00:00<00:08, 7.78it/s]
8%|##########1 | Repairing epochs : 6/72 [00:00<00:08, 7.64it/s]
10%|###########8 | Repairing epochs : 7/72 [00:00<00:08, 7.54it/s]
11%|#############5 | Repairing epochs : 8/72 [00:01<00:08, 7.60it/s]
12%|###############2 | Repairing epochs : 9/72 [00:01<00:08, 7.65it/s]
14%|################8 | Repairing epochs : 10/72 [00:01<00:08, 7.69it/s]
15%|##################4 | Repairing epochs : 11/72 [00:01<00:07, 7.72it/s]
17%|####################1 | Repairing epochs : 12/72 [00:01<00:07, 7.65it/s]
18%|#####################8 | Repairing epochs : 13/72 [00:01<00:07, 7.68it/s]
19%|#######################5 | Repairing epochs : 14/72 [00:01<00:07, 7.71it/s]
21%|#########################2 | Repairing epochs : 15/72 [00:01<00:07, 7.73it/s]
22%|##########################8 | Repairing epochs : 16/72 [00:02<00:07, 7.75it/s]
24%|############################5 | Repairing epochs : 17/72 [00:02<00:07, 7.69it/s]
25%|##############################2 | Repairing epochs : 18/72 [00:02<00:07, 7.71it/s]
26%|###############################9 | Repairing epochs : 19/72 [00:02<00:06, 7.70it/s]
28%|#################################6 | Repairing epochs : 20/72 [00:02<00:06, 7.68it/s]
29%|###################################2 | Repairing epochs : 21/72 [00:02<00:06, 7.70it/s]
31%|####################################9 | Repairing epochs : 22/72 [00:02<00:06, 7.72it/s]
32%|######################################6 | Repairing epochs : 23/72 [00:02<00:06, 7.74it/s]
33%|########################################3 | Repairing epochs : 24/72 [00:03<00:06, 7.75it/s]
35%|########################################## | Repairing epochs : 25/72 [00:03<00:06, 7.70it/s]
36%|###########################################6 | Repairing epochs : 26/72 [00:03<00:05, 7.72it/s]
38%|#############################################3 | Repairing epochs : 27/72 [00:03<00:05, 7.74it/s]
39%|############################################### | Repairing epochs : 28/72 [00:03<00:05, 7.75it/s]
40%|################################################7 | Repairing epochs : 29/72 [00:03<00:05, 7.71it/s]
42%|##################################################4 | Repairing epochs : 30/72 [00:03<00:05, 7.72it/s]
43%|#################################################### | Repairing epochs : 31/72 [00:04<00:05, 7.74it/s]
44%|#####################################################7 | Repairing epochs : 32/72 [00:04<00:05, 7.75it/s]
46%|#######################################################4 | Repairing epochs : 33/72 [00:04<00:05, 7.74it/s]
47%|#########################################################1 | Repairing epochs : 34/72 [00:04<00:04, 7.78it/s]
49%|##########################################################8 | Repairing epochs : 35/72 [00:04<00:04, 7.79it/s]
50%|############################################################5 | Repairing epochs : 36/72 [00:04<00:04, 7.74it/s]
51%|##############################################################1 | Repairing epochs : 37/72 [00:04<00:04, 7.76it/s]
53%|###############################################################8 | Repairing epochs : 38/72 [00:04<00:04, 7.77it/s]
54%|#################################################################5 | Repairing epochs : 39/72 [00:05<00:04, 7.78it/s]
56%|###################################################################2 | Repairing epochs : 40/72 [00:05<00:04, 7.79it/s]
57%|####################################################################9 | Repairing epochs : 41/72 [00:05<00:03, 7.80it/s]
58%|######################################################################5 | Repairing epochs : 42/72 [00:05<00:03, 7.81it/s]
60%|########################################################################2 | Repairing epochs : 43/72 [00:05<00:03, 7.82it/s]
61%|#########################################################################9 | Repairing epochs : 44/72 [00:05<00:03, 7.77it/s]
62%|###########################################################################6 | Repairing epochs : 45/72 [00:05<00:03, 7.73it/s]
64%|#############################################################################3 | Repairing epochs : 46/72 [00:05<00:03, 7.74it/s]
65%|##############################################################################9 | Repairing epochs : 47/72 [00:06<00:03, 7.70it/s]
67%|################################################################################6 | Repairing epochs : 48/72 [00:06<00:03, 7.72it/s]
68%|##################################################################################3 | Repairing epochs : 49/72 [00:06<00:02, 7.73it/s]
69%|#################################################################################### | Repairing epochs : 50/72 [00:06<00:02, 7.69it/s]
71%|#####################################################################################7 | Repairing epochs : 51/72 [00:06<00:02, 7.71it/s]
72%|#######################################################################################3 | Repairing epochs : 52/72 [00:06<00:02, 7.72it/s]
74%|######################################################################################### | Repairing epochs : 53/72 [00:06<00:02, 7.63it/s]
75%|##########################################################################################7 | Repairing epochs : 54/72 [00:07<00:02, 7.65it/s]
76%|############################################################################################4 | Repairing epochs : 55/72 [00:07<00:02, 7.67it/s]
78%|##############################################################################################1 | Repairing epochs : 56/72 [00:07<00:02, 7.64it/s]
79%|###############################################################################################7 | Repairing epochs : 57/72 [00:07<00:01, 7.65it/s]
81%|#################################################################################################4 | Repairing epochs : 58/72 [00:07<00:01, 7.67it/s]
82%|###################################################################################################1 | Repairing epochs : 59/72 [00:07<00:01, 7.63it/s]
83%|####################################################################################################8 | Repairing epochs : 60/72 [00:07<00:01, 7.65it/s]
85%|######################################################################################################5 | Repairing epochs : 61/72 [00:07<00:01, 7.62it/s]
86%|########################################################################################################1 | Repairing epochs : 62/72 [00:08<00:01, 7.58it/s]
88%|#########################################################################################################8 | Repairing epochs : 63/72 [00:08<00:01, 7.56it/s]
89%|###########################################################################################################5 | Repairing epochs : 64/72 [00:08<00:01, 7.54it/s]
90%|#############################################################################################################2 | Repairing epochs : 65/72 [00:08<00:00, 7.56it/s]
92%|##############################################################################################################9 | Repairing epochs : 66/72 [00:08<00:00, 7.58it/s]
93%|################################################################################################################5 | Repairing epochs : 67/72 [00:08<00:00, 7.60it/s]
94%|##################################################################################################################2 | Repairing epochs : 68/72 [00:08<00:00, 7.52it/s]
96%|###################################################################################################################9 | Repairing epochs : 69/72 [00:09<00:00, 7.50it/s]
97%|#####################################################################################################################6 | Repairing epochs : 70/72 [00:09<00:00, 7.48it/s]
99%|#######################################################################################################################3 | Repairing epochs : 71/72 [00:09<00:00, 7.50it/s]
100%|#########################################################################################################################| Repairing epochs : 72/72 [00:09<00:00, 7.48it/s]
100%|#########################################################################################################################| Repairing epochs : 72/72 [00:09<00:00, 7.63it/s]
No bad epochs were found for your data. Returning a copy of the data you wanted to clean. Interpolation may have been done.
NOTE: pick_channels() is a legacy function. New code should use inst.pick(...).
NOTE: pick_channels() is a legacy function. New code should use inst.pick(...).
Now, we will manually mark the bad channels just for plotting.
evoked.info['bads'] = ['MEG 2443']
evoked_clean.info['bads'] = ['MEG 2443']
Let us plot the results.
import matplotlib.pyplot as plt # noqa
set_matplotlib_defaults(plt)
fig, axes = plt.subplots(2, 1, figsize=(6, 6))
for ax in axes:
ax.tick_params(axis='x', which='both', bottom='off', top='off')
ax.tick_params(axis='y', which='both', left='off', right='off')
ylim = dict(grad=(-170, 200))
evoked.pick_types(meg='grad', exclude=[])
evoked.plot(exclude=[], axes=axes[0], ylim=ylim, show=False)
axes[0].set_title('Before autoreject')
evoked_clean.pick_types(meg='grad', exclude=[])
evoked_clean.plot(exclude=[], axes=axes[1], ylim=ylim)
axes[1].set_title('After autoreject')
plt.tight_layout()
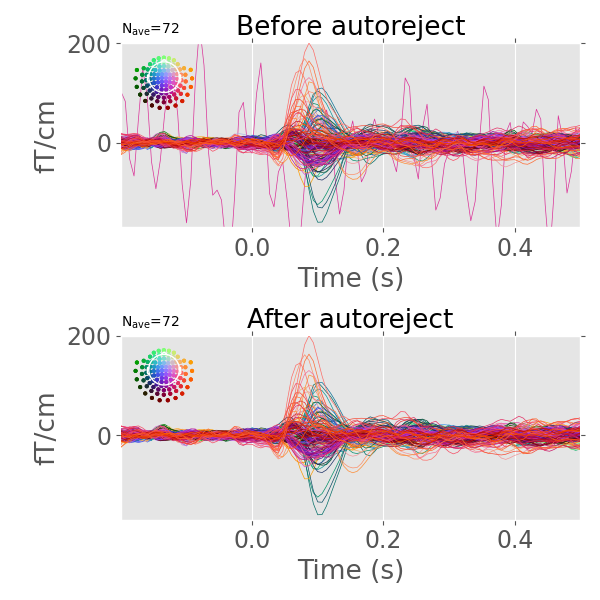
NOTE: pick_types() is a legacy function. New code should use inst.pick(...).
NOTE: pick_types() is a legacy function. New code should use inst.pick(...).
C:\Users\stefan\Desktop\git-repos\autoreject\examples\plot_auto_repair.py:133: UserWarning: This figure includes Axes that are not compatible with tight_layout, so results might be incorrect.
plt.tight_layout()
To top things up, we can also visualize the bad sensors for each trial using a heatmap.
ar.get_reject_log(epochs['Auditory/Left']).plot()
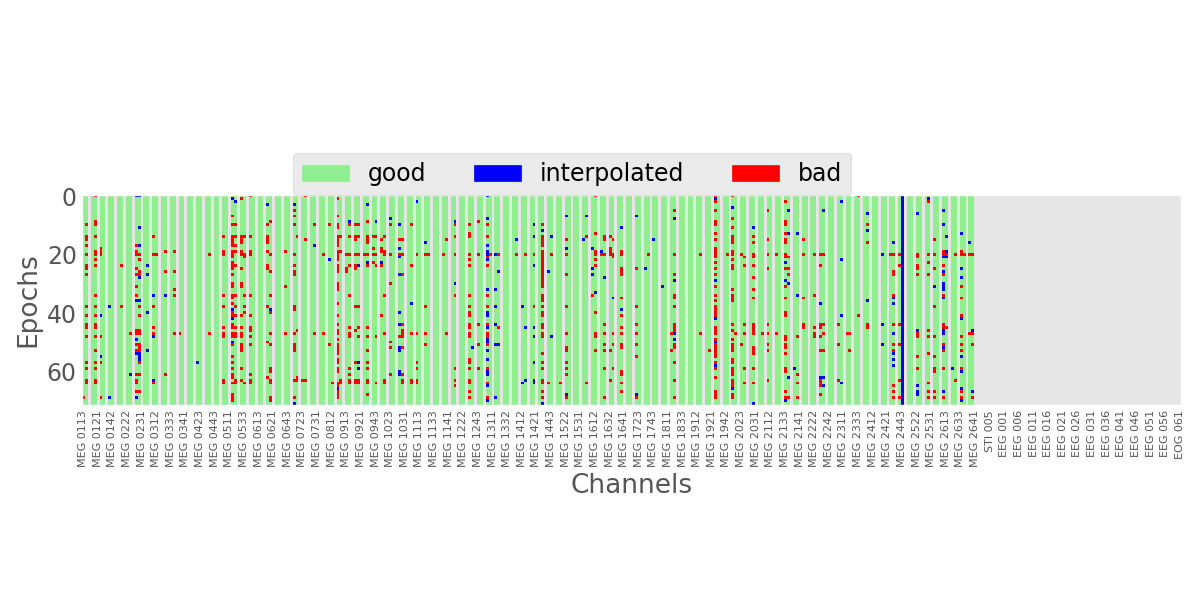
<Figure size 1200x600 with 1 Axes>
Total running time of the script: ( 1 minutes 47.137 seconds)